用C++如何通过自定义QAbstractItemModel类实现一个表格模型
时间: 2023-12-14 14:37:35 浏览: 128
要通过自定义 `QAbstractItemModel` 类实现一个表格模型,需要以下步骤:
1. 定义一个继承自 `QAbstractItemModel` 的子类。
2. 实现 `rowCount()`、`columnCount()`、`data()` 和 `setData()` 函数,它们是模型的核心函数。其中:
- `rowCount()` 和 `columnCount()` 函数分别返回行数和列数。
- `data()` 函数返回一个 `QVariant` 类型的值,该值表示模型中指定索引处的数据。当视图需要显示某个索引处的数据时,它会调用 `data()` 函数。
- `setData()` 函数用于设置模型中指定索引处的数据。当视图需要更改某个索引处的数据时,它会调用 `setData()` 函数。
3. 实现 `index()` 和 `parent()` 函数,它们是用于访问模型中的节点的函数。
- `index()` 函数返回一个 `QModelIndex` 类型的对象,该对象包含节点的行和列信息以及指向该节点的指针。
- `parent()` 函数返回指向父节点的指针。
4. 为了支持模型中的数据更改,需要在模型中定义一个数据结构,用于存储表格中的数据。数据结构可以是一个二维数组、一个列表或其他容器对象。
5. 在 `QTableView` 或其他视图中使用自定义模型。
下面是一个简单的示例代码,演示如何创建一个自定义表格模型:
```cpp
#include <QAbstractItemModel>
#include <QModelIndex>
#include <QVariant>
class MyTableModel : public QAbstractItemModel
{
public:
MyTableModel(QObject *parent = nullptr);
~MyTableModel();
// 重写必要的函数
QModelIndex index(int row, int column, const QModelIndex &parent = QModelIndex()) const override;
QModelIndex parent(const QModelIndex &index) const override;
int rowCount(const QModelIndex &parent = QModelIndex()) const override;
int columnCount(const QModelIndex &parent = QModelIndex()) const override;
QVariant data(const QModelIndex &index, int role = Qt::DisplayRole) const override;
bool setData(const QModelIndex &index, const QVariant &value, int role = Qt::EditRole) override;
private:
// 存储数据的数据结构
QVector<QVector<QVariant>> m_data;
};
```
其中,`m_data` 是用于存储表格数据的二维向量。
接下来,我们需要实现这些函数:
```cpp
MyTableModel::MyTableModel(QObject *parent)
: QAbstractItemModel(parent)
{
// 初始化数据结构
m_data.resize(10);
for (int i = 0; i < 10; ++i) {
m_data[i].resize(10);
for (int j = 0; j < 10; ++j) {
m_data[i][j] = i * j; // 假设表格的数据是行列相乘的结果
}
}
}
MyTableModel::~MyTableModel()
{
}
QModelIndex MyTableModel::index(int row, int column, const QModelIndex &parent) const
{
if (!hasIndex(row, column, parent))
return QModelIndex();
return createIndex(row, column);
}
QModelIndex MyTableModel::parent(const QModelIndex &index) const
{
return QModelIndex();
}
int MyTableModel::rowCount(const QModelIndex &parent) const
{
if (parent.isValid())
return 0;
return m_data.size();
}
int MyTableModel::columnCount(const QModelIndex &parent) const
{
if (parent.isValid())
return 0;
return m_data[0].size();
}
QVariant MyTableModel::data(const QModelIndex &index, int role) const
{
if (!index.isValid())
return QVariant();
if (role == Qt::DisplayRole) {
return m_data[index.row()][index.column()];
}
return QVariant();
}
bool MyTableModel::setData(const QModelIndex &index, const QVariant &value, int role)
{
if (!index.isValid())
return false;
if (role == Qt::EditRole) {
m_data[index.row()][index.column()] = value;
emit dataChanged(index, index);
return true;
}
return false;
}
```
最后,我们需要在 `QTableView` 中使用这个自定义模型:
```cpp
#include <QApplication>
#include <QTableView>
int main(int argc, char *argv[])
{
QApplication a(argc, argv);
MyTableModel model;
QTableView view;
view.setModel(&model);
view.show();
return a.exec();
}
```
通过以上步骤,便可以创建一个简单的自定义表格模型,可以在视图中显示和编辑表格数据。
阅读全文
相关推荐
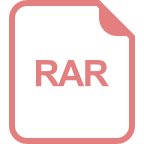
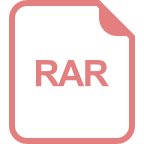
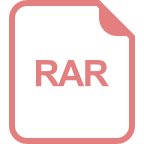


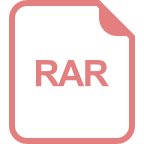
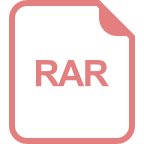
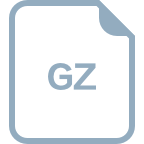
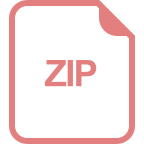
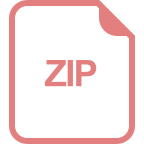
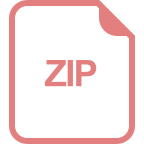
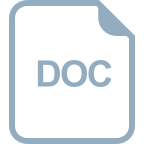
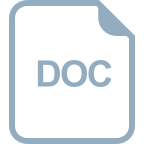
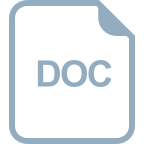
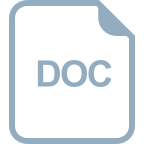
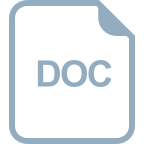
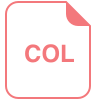
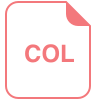
