vue3+Django3实现登陆接口
时间: 2023-11-22 07:04:57 浏览: 96
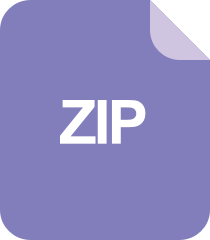
实现基于Vue3组合式API的登陆系统
在 Vue3 中调用 Django3 的登录接口,需要在 Django3 中编写登录视图,并使用 Django 自带的用户认证系统来验证用户身份。以下是实现步骤:
1. 在 Django3 项目中创建一个 app,并在 app 中创建一个登录视图:
```python
from django.contrib.auth import authenticate, login
from django.http import JsonResponse
from django.views.decorators.csrf import csrf_exempt
@csrf_exempt
def login_view(request):
if request.method == 'POST':
username = request.POST.get('username')
password = request.POST.get('password')
user = authenticate(request, username=username, password=password)
if user is not None:
login(request, user)
return JsonResponse({'success': True})
else:
return JsonResponse({'success': False, 'message': '用户名或密码错误'})
```
在这里,使用 Django 自带的 `authenticate` 函数来验证用户身份,并使用 `login` 函数将用户登录。登录成功时返回 `{'success': True}`,登录失败时返回 `{'success': False, 'message': '用户名或密码错误'}`。
2. 在 Vue3 组件中使用 axios 发送登录请求:
```javascript
import axios from 'axios'
export default {
data() {
return {
username: '',
password: ''
}
},
methods: {
async login() {
try {
const response = await axios.post('/api/login/', {
username: this.username,
password: this.password
})
if (response.data.success) {
// 登录成功后的逻辑
} else {
console.error(response.data.message)
// 登录失败后的逻辑
}
} catch (error) {
console.error(error)
// 登录失败后的逻辑
}
}
}
}
```
在这里,假设 Django3 的登录接口是 `/api/login/`,并且接收 `username` 和 `password` 两个参数。登录成功时调用登录成功后的逻辑,登录失败时调用登录失败后的逻辑。
这样就可以在 Vue3 中调用 Django3 的登录接口了。注意,在开发过程中,需要同时启动 Vue3 和 Django3 的开发服务器,并且将 Vue3 的开发服务器代理到 Django3 的开发服务器上。
阅读全文
相关推荐
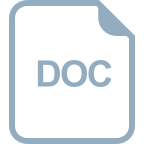
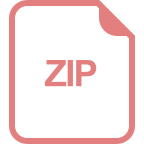
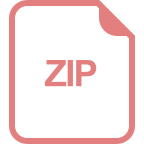
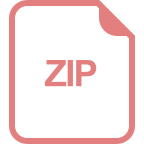
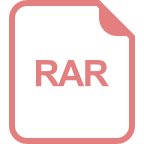
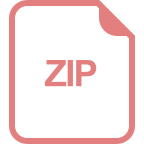
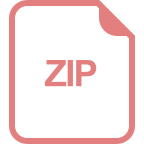
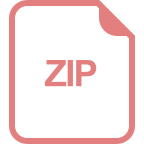
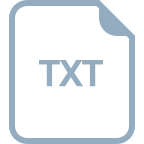
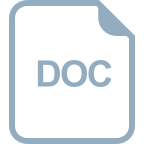
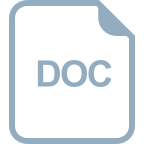
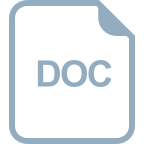
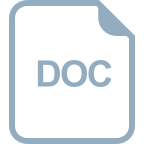
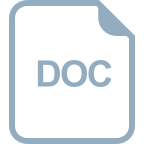
