matplotlib画多条折线图
时间: 2023-09-12 16:07:05 浏览: 133
要在 matplotlib 中画多条折线图,可以使用 plt.plot() 函数并传入不同的数据。以下是一个示例代码,其中包含三条折线图:
```python
import matplotlib.pyplot as plt
import numpy as np
# 生成随机数据
x = np.linspace(0, 10, 100)
y1 = np.sin(x)
y2 = np.cos(x)
y3 = np.tan(x)
# 绘制折线图
plt.plot(x, y1)
plt.plot(x, y2)
plt.plot(x, y3)
# 设置图例和标题
plt.legend(['sin(x)', 'cos(x)', 'tan(x)'])
plt.title('Multiple Line Chart')
# 显示图形
plt.show()
```
在这个示例中,我们使用 numpy 库生成了三个不同的数据集,然后使用 plt.plot() 函数分别绘制了它们。我们还使用 plt.legend() 函数添加了一个图例,并使用 plt.title() 函数设置了标题。最后,我们调用 plt.show() 函数显示图形。
相关问题
如何使用python的matplotlib画多条折线图
要使用Python的Matplotlib库画多条折线图,可以按照以下步骤进行操作:
1. 导入所需的库:
```python
import matplotlib.pyplot as plt
```
2. 准备数据:
准备好要绘制的多条折线的数据。假设有两条折线,分别对应x和y1、y2的坐标值。
3. 创建图表:
```python
plt.figure()
```
4. 绘制折线图:
使用`plt.plot()`函数绘制多条折线图。传入x和y的坐标值即可。
```python
plt.plot(x, y1, label='Line 1')
plt.plot(x, y2, label='Line 2')
```
可以通过修改`label`参数来为每条折线添加标签。
5. 添加图例:
使用`plt.legend()`函数添加图例,显示每条折线对应的标签。
```python
plt.legend()
```
6. 添加标题和标签:
使用`plt.title()`、`plt.xlabel()`和`plt.ylabel()`函数可以分别添加图表的标题、x轴标签和y轴标签。
```python
plt.title('Multiple Line Chart')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
```
7. 显示图表:
最后使用`plt.show()`函数显示绘制好的图表。
```python
plt.show()
```
完整的代码示例:
```python
import matplotlib.pyplot as plt
# 准备数据
x = [1, 2, 3, 4, 5]
y1 = [1, 4, 9, 16, 25]
y2 = [1, 8, 27, 64, 125]
# 创建图表
plt.figure()
# 绘制折线图
plt.plot(x, y1, label='Line 1')
plt.plot(x, y2, label='Line 2')
# 添加图例
plt.legend()
# 添加标题和标签
plt.title('Multiple Line Chart')
plt.xlabel('X-axis')
plt.ylabel('Y-axis')
# 显示图表
plt.show()
```
运行以上代码,就能够绘制出包含两条折线的多条折线图。你可以根据自己的需求修改数据和图表样式。
matplotlib绘制多条折线图代码
下面是一个简单的例子,演示如何使用matplotlib绘制多条折线图:
```python
import matplotlib.pyplot as plt
# 数据
x = [1, 2, 3, 4, 5]
y1 = [1, 3, 2, 4, 5]
y2 = [2, 4, 3, 5, 6]
y3 = [3, 5, 4, 6, 7]
# 绘制折线图
plt.plot(x, y1, label='Line 1')
plt.plot(x, y2, label='Line 2')
plt.plot(x, y3, label='Line 3')
# 设置图例
plt.legend()
# 设置标题和坐标轴标签
plt.title('Multiple Line Plot')
plt.xlabel('X Axis')
plt.ylabel('Y Axis')
# 显示图形
plt.show()
```
这个例子中,我们使用了三个列表 `x`、`y1`、`y2`和 `y3` 来表示三条折线图的横坐标和纵坐标数据。然后,我们使用 `plt.plot()` 函数分别绘制了三条折线图,并使用 `label` 参数设置每条折线图的标签。最后,我们使用 `plt.legend()` 函数来显示图例,使得用户可以区分不同的折线图。在设置完标题和坐标轴标签后,我们调用 `plt.show()` 函数显示图形。可以根据需要在代码中修改数据、标签和其他参数,以生成自己需要的多条折线图。
阅读全文
相关推荐
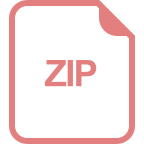
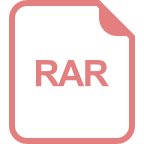
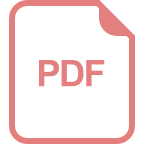






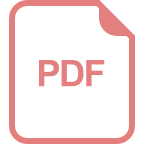
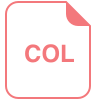
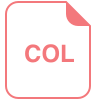




