vue3 ts写cnode
时间: 2023-08-28 11:06:27 浏览: 45
首先,你需要引入相关的依赖包,包括vue、vue-router、axios等。
安装依赖:
```bash
npm install vue vue-router axios --save
npm install @types/vue @types/vue-router @types/axios --save-dev
```
然后,你需要创建一个Vue组件,该组件将显示CNode社区的帖子列表。你可以使用Axios库从CNode社区的API中获取帖子列表。
```typescript
<template>
<div class="post-list">
<div v-for="post in posts" :key="post.id">
<router-link :to="'/post/' + post.id">{{ post.title }}</router-link>
</div>
</div>
</template>
<script lang="ts">
import { defineComponent } from 'vue'
import axios from 'axios'
export default defineComponent({
data() {
return {
posts: []
}
},
async created() {
const response = await axios.get('https://cnodejs.org/api/v1/topics')
this.posts = response.data.data
}
})
</script>
<style scoped>
.post-list {
margin-top: 20px;
}
.post-list div {
margin-bottom: 10px;
}
</style>
```
这个组件使用了Vue的单文件组件格式,它包含了一个模板、一个脚本和一个样式。
在脚本中,我们使用Axios库从CNode社区的API中获取帖子列表,并将其存储在组件的数据属性中。我们使用created钩子来执行异步请求。
在模板中,我们使用v-for指令来遍历帖子列表,并使用router-link组件来生成每个帖子的链接。
最后,在样式中,我们为帖子列表添加了一些样式,使其看起来更美观。
接下来,你需要创建一个Vue路由器,并在其中定义路由。在这里,我们将定义一个路由,该路由将显示单个帖子的详细信息。
```typescript
import { createRouter, createWebHistory, RouteRecordRaw } from 'vue-router'
import PostList from './components/PostList.vue'
import PostDetail from './components/PostDetail.vue'
const routes: Array<RouteRecordRaw> = [
{
path: '/',
name: 'PostList',
component: PostList
},
{
path: '/post/:id',
name: 'PostDetail',
component: PostDetail
}
]
const router = createRouter({
history: createWebHistory(),
routes
})
export default router
```
在这个路由器中,我们定义了两个路由。第一个路由将显示帖子列表,第二个路由将显示单个帖子的详细信息。
在第二个路由中,我们使用了动态路由参数:id。这使得我们可以在路由中传递帖子的ID,并在PostDetail组件中使用它来加载正确的帖子。
最后,我们使用createWebHistory函数创建一个Vue路由器,该函数使用HTML5历史记录API来管理路由状态。我们将路由器导出,以便在Vue应用程序中使用它。
最后,你需要将这些组件和路由器集成到一个Vue应用程序中。
在main.ts文件中,你可以创建一个Vue应用程序实例,并将其挂载到DOM中。
```typescript
import { createApp } from 'vue'
import App from './App.vue'
import router from './router'
createApp(App).use(router).mount('#app')
```
在这个应用程序中,我们使用createApp函数创建一个Vue应用程序实例,并将其绑定到App组件上。我们还使用use方法将路由器添加到应用程序中。
最后,我们使用mount方法将应用程序实例挂载到DOM中。
现在,你可以使用npm run serve命令启动应用程序,并在浏览器中查看它。
相关推荐
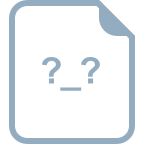














