(1)定义一个接口Compute含有一个方法int computer(int n,int m); (2)设计四个类分别实现此接口,完成+-*/运算 (3)设计一个类UseCompute,含有方法: p...
时间: 2024-03-23 13:38:04 浏览: 92
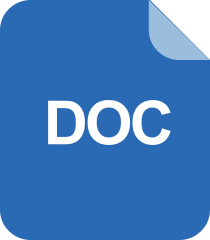
请编制函数Compute()分别计算出xx中偶数的个数eve
好的,我理解了你的问题。以下是我对你的问题的回答:
1. 首先,我们定义一个接口Compute,其中包含一个方法compute(int n, int m),如下所示:
```
public interface Compute {
int compute(int n, int m);
}
```
2. 然后,我们设计四个类Addition、Subtraction、Multiplication、Division分别实现Compute接口,完成加、减、乘、除运算,如下所示:
```
public class Addition implements Compute {
@Override
public int compute(int n, int m) {
return n + m;
}
}
public class Subtraction implements Compute {
@Override
public int compute(int n, int m) {
return n - m;
}
}
public class Multiplication implements Compute {
@Override
public int compute(int n, int m) {
return n * m;
}
}
public class Division implements Compute {
@Override
public int compute(int n, int m) {
if (m == 0) {
throw new IllegalArgumentException("Divisor cannot be zero");
}
return n / m;
}
}
```
3. 接下来,我们设计一个类UseCompute,其中包含一个方法printResult(Compute compute, int n, int m),接受一个Compute实例和两个整型参数n和m,用来调用compute方法并输出结果,如下所示:
```
public class UseCompute {
public void printResult(Compute compute, int n, int m) {
int result = compute.compute(n, m);
System.out.println("Result: " + result);
}
}
```
4. 最后,我们在主函数中使用UseCompute类,创建Addition、Subtraction、Multiplication和Division实例,并调用printResult方法输出结果,如下所示:
```
public static void main(String[] args) {
UseCompute useCompute = new UseCompute();
Compute addition = new Addition();
Compute subtraction = new Subtraction();
Compute multiplication = new Multiplication();
Compute division = new Division();
useCompute.printResult(addition, 10, 5); // Output: Result: 15
useCompute.printResult(subtraction, 10, 5); // Output: Result: 5
useCompute.printResult(multiplication, 10, 5); // Output: Result: 50
useCompute.printResult(division, 10, 5); // Output: Result: 2
}
```
希望这个回答能够帮到你。如果你还有其他问题,请随时问我。
阅读全文
相关推荐
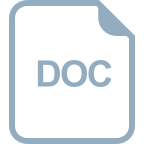
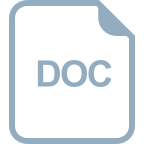















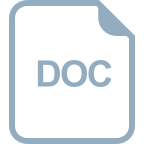