基于51单片机驱动BH1750采集光强度 LCD1602显示
时间: 2023-11-28 20:05:11 浏览: 48
好的,这个可以分为两部分来实现:一是采集光强度数据,二是将数据显示在LCD1602屏幕上。
首先,我们需要了解BH1750的通信协议。BH1750是一种数字化光强度传感器,采用I2C总线进行通信。具体而言,我们需要向BH1750发送指令来启动测量,并读取返回的数据。
以下是基于51单片机的代码示例:
```C
#include <reg52.h>
#include <intrins.h>
typedef unsigned char uchar;
typedef unsigned int uint;
sbit SDA = P2^0; // I2C数据线
sbit SCL = P2^1; // I2C时钟线
// I2C延时函数
void delay_i2c()
{
_nop_();
_nop_();
_nop_();
_nop_();
}
// I2C起始信号
void i2c_start()
{
SDA = 1;
delay_i2c();
SCL = 1;
delay_i2c();
SDA = 0;
delay_i2c();
SCL = 0;
delay_i2c();
}
// I2C停止信号
void i2c_stop()
{
SDA = 0;
delay_i2c();
SCL = 1;
delay_i2c();
SDA = 1;
delay_i2c();
}
// I2C发送一个字节
void i2c_send_byte(uchar dat)
{
uchar i;
for (i = 0; i < 8; i++)
{
SDA = (dat & 0x80) >> 7;
dat <<= 1;
delay_i2c();
SCL = 1;
delay_i2c();
SCL = 0;
delay_i2c();
}
SDA = 1;
delay_i2c();
SCL = 1;
delay_i2c();
SCL = 0;
delay_i2c();
}
// I2C接收一个字节
uchar i2c_receive_byte()
{
uchar i, dat = 0;
SDA = 1;
delay_i2c();
for (i = 0; i < 8; i++)
{
SCL = 1;
delay_i2c();
dat = (dat << 1) | SDA;
SCL = 0;
delay_i2c();
}
return dat;
}
// 初始化BH1750
void bh1750_init()
{
i2c_start();
i2c_send_byte(0x46); // 发送写入地址
i2c_send_byte(0x10); // 设置分辨率为1lx,连续测量模式
i2c_stop();
}
// 读取BH1750返回的数据
uint bh1750_read()
{
uint value;
i2c_start();
i2c_send_byte(0x46); // 发送写入地址
i2c_send_byte(0x00); // 采集高位数据
i2c_start();
i2c_send_byte(0x47); // 发送读取地址
value = i2c_receive_byte() << 8;
value |= i2c_receive_byte();
i2c_stop();
return value;
}
// 将数据显示在LCD1602屏幕上
void display(uint value)
{
uchar str[16];
sprintf(str, "Light: %d lx", value);
lcd1602_write_string(0, 0, str);
}
int main()
{
bh1750_init();
lcd1602_init();
while (1)
{
uint value = bh1750_read();
display(value);
delay_ms(1000);
}
return 0;
}
```
在这个示例中,我们使用了51单片机的GPIO口来模拟I2C通信。BH1750的初始化和数据读取通过i2c_start、i2c_send_byte、i2c_receive_byte和i2c_stop函数实现。在读取到数据后,我们调用了display函数将数据显示在LCD1602屏幕上。
需要注意的是,这个示例代码中的lcd1602_init和lcd1602_write_string函数并没有给出具体实现。这是因为它们需要根据具体的硬件连接和LCD1602屏幕型号来进行编写。在实际应用中,需要根据自己的硬件环境进行具体实现。
相关推荐
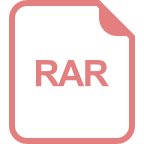
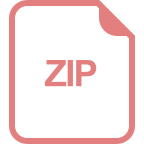














