springcloud连接数据库代码
时间: 2023-06-13 14:07:26 浏览: 109
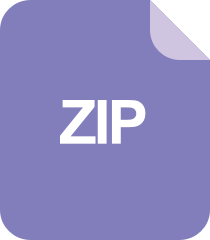
白色大气风格的旅游酒店企业网站模板.zip
以下是使用Spring Cloud连接MySQL数据库的示例代码:
1. 添加依赖
在pom.xml文件中添加以下依赖:
```
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-config</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.cloud</groupId>
<artifactId>spring-cloud-starter-netflix-eureka-client</artifactId>
</dependency>
<dependency>
<groupId>org.springframework.boot</groupId>
<artifactId>spring-boot-starter-data-jpa</artifactId>
</dependency>
<dependency>
<groupId>mysql</groupId>
<artifactId>mysql-connector-java</artifactId>
</dependency>
```
2. 添加配置文件
在resources目录下添加application.yml文件,配置数据库连接信息:
```
spring:
datasource:
url: jdbc:mysql://localhost:3306/test?useUnicode=true&characterEncoding=utf8&useSSL=false&serverTimezone=GMT%2B8
username: root
password: 123456
driver-class-name: com.mysql.jdbc.Driver
```
3. 创建实体类
创建一个实体类,对应数据库中的表:
```
@Entity
@Table(name = "user")
public class User {
@Id
@GeneratedValue(strategy = GenerationType.IDENTITY)
private Long id;
@Column(nullable = false)
private String name;
@Column(nullable = false)
private Integer age;
// getter和setter方法
}
```
4. 创建仓库接口
创建一个继承自JpaRepository的接口:
```
public interface UserRepository extends JpaRepository<User, Long> {
}
```
5. 创建服务类
创建一个服务类,注入UserRepository,并实现一些数据操作方法:
```
@Service
public class UserService {
@Autowired
private UserRepository userRepository;
public List<User> getAllUsers() {
return userRepository.findAll();
}
public User getUserById(Long id) {
return userRepository.findById(id).orElse(null);
}
public User saveUser(User user) {
return userRepository.save(user);
}
public void deleteUser(Long id) {
userRepository.deleteById(id);
}
}
```
6. 创建控制器
创建一个控制器,注入UserService,并实现一些接口:
```
@RestController
public class UserController {
@Autowired
private UserService userService;
@GetMapping("/users")
public List<User> getAllUsers() {
return userService.getAllUsers();
}
@GetMapping("/users/{id}")
public User getUserById(@PathVariable Long id) {
return userService.getUserById(id);
}
@PostMapping("/users")
public User saveUser(@RequestBody User user) {
return userService.saveUser(user);
}
@DeleteMapping("/users/{id}")
public void deleteUser(@PathVariable Long id) {
userService.deleteUser(id);
}
}
```
7. 启动应用程序
在应用程序的主类上添加注解@EnableEurekaClient和@SpringBootApplication,并在main方法中启动应用程序:
```
@EnableEurekaClient
@SpringBootApplication
public class Application {
public static void main(String[] args) {
SpringApplication.run(Application.class, args);
}
}
```
这样就完成了使用Spring Cloud连接MySQL数据库的代码示例。
阅读全文
相关推荐
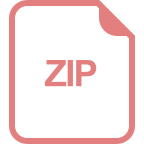
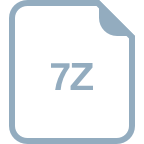
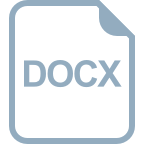
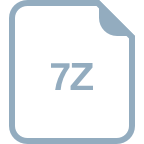
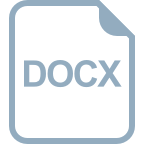
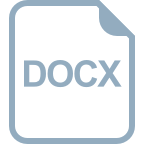
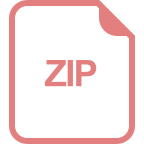