写一个贪吃蛇的程序c语言
时间: 2023-09-06 17:14:33 浏览: 89
以下是一个简单的贪吃蛇游戏程序,使用了C语言和Windows API:
```c
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#include <windows.h>
// 定义常量
#define WIDTH 50
#define HEIGHT 20
#define SNAKE_MAX_LENGTH (WIDTH * HEIGHT)
// 定义结构体
typedef struct {
int x;
int y;
} Position;
// 定义全局变量
Position snake[SNAKE_MAX_LENGTH];
int snakeLength = 3;
Position food;
int score = 0;
// 打印地图
void drawMap() {
system("cls"); // 清屏
for (int y = 0; y < HEIGHT; y++) {
for (int x = 0; x < WIDTH; x++) {
if (x == 0 || x == WIDTH - 1 || y == 0 || y == HEIGHT - 1) {
printf("#"); // 边界
} else if (x == food.x && y == food.y) {
printf("$"); // 食物
} else {
int isSnakeBody = 0;
for (int i = 0; i < snakeLength; i++) {
if (snake[i].x == x && snake[i].y == y) {
printf("*"); // 蛇身
isSnakeBody = 1;
break;
}
}
if (!isSnakeBody) {
printf(" "); // 空格
}
}
}
printf("\n");
}
printf("Score: %d\n", score); // 分数
}
// 生成食物
void generateFood() {
int x, y;
do {
x = rand() % (WIDTH - 2) + 1;
y = rand() % (HEIGHT - 2) + 1;
} while (x == food.x && y == food.y);
food.x = x;
food.y = y;
}
// 向左移动
void moveLeft() {
for (int i = snakeLength - 1; i > 0; i--) {
snake[i].x = snake[i - 1].x;
snake[i].y = snake[i - 1].y;
}
snake[0].x--;
}
// 向右移动
void moveRight() {
for (int i = snakeLength - 1; i > 0; i--) {
snake[i].x = snake[i - 1].x;
snake[i].y = snake[i - 1].y;
}
snake[0].x++;
}
// 向上移动
void moveUp() {
for (int i = snakeLength - 1; i > 0; i--) {
snake[i].x = snake[i - 1].x;
snake[i].y = snake[i - 1].y;
}
snake[0].y--;
}
// 向下移动
void moveDown() {
for (int i = snakeLength - 1; i > 0; i--) {
snake[i].x = snake[i - 1].x;
snake[i].y = snake[i - 1].y;
}
snake[0].y++;
}
// 判断游戏是否结束
int isGameOver() {
if (snake[0].x == 0 || snake[0].x == WIDTH - 1
|| snake[0].y == 0 || snake[0].y == HEIGHT - 1) {
return 1; // 撞墙了
}
for (int i = 1; i < snakeLength; i++) {
if (snake[i].x == snake[0].x && snake[i].y == snake[0].y) {
return 1; // 撞到自己了
}
}
return 0;
}
int main() {
srand((unsigned int)time(NULL)); // 初始化随机数种子
snake[0].x = WIDTH / 2;
snake[0].y = HEIGHT / 2;
snake[1].x = snake[0].x - 1;
snake[1].y = snake[0].y;
snake[2].x = snake[1].x - 1;
snake[2].y = snake[1].y;
generateFood();
drawMap();
while (!isGameOver()) {
// 处理输入
if (GetAsyncKeyState(VK_LEFT) & 0x8000) {
moveLeft();
} else if (GetAsyncKeyState(VK_RIGHT) & 0x8000) {
moveRight();
} else if (GetAsyncKeyState(VK_UP) & 0x8000) {
moveUp();
} else if (GetAsyncKeyState(VK_DOWN) & 0x8000) {
moveDown();
}
// 判断是否吃到食物
if (snake[0].x == food.x && snake[0].y == food.y) {
snakeLength++;
score++;
generateFood();
}
// 绘制地图
drawMap();
// 等待一会儿
Sleep(100);
}
printf("Game Over!\n");
printf("Your Score: %d\n", score);
return 0;
}
```
这个程序使用了Windows API中的GetAsyncKeyState和Sleep函数,需要在Windows操作系统下运行。如果要在其他操作系统下运行,需要使用其他的输入和延时函数。
阅读全文
相关推荐
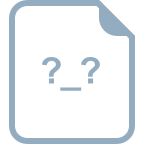
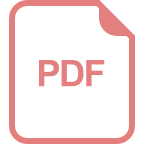
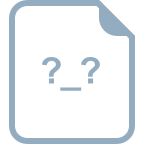





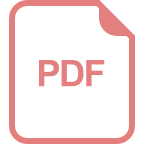