使用KMeans算法对原始图像进行聚类,将相似的像素进行分组,然后对每个像素组分别应用CLAHE算法进行增强。代码
时间: 2024-02-05 15:14:12 浏览: 75
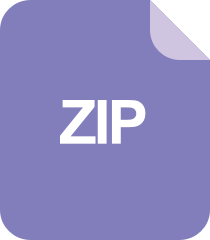
kmeans图像聚类程序

以下是使用sklearn库中的KMeans算法和OpenCV库中的CLAHE算法对原始图像进行聚类和增强的代码示例:
```python
import cv2
import numpy as np
from sklearn.cluster import KMeans
# Load image
img = cv2.imread('image.jpg')
# Reshape image to 2D array for KMeans clustering
img_reshaped = img.reshape((-1, 3))
# Perform KMeans clustering
kmeans = KMeans(n_clusters=8, random_state=0).fit(img_reshaped)
labels = kmeans.labels_
centers = kmeans.cluster_centers_
# Reshape labels and centers to match original image shape
labels_reshaped = labels.reshape(img.shape[:2])
centers_reshaped = centers.reshape((1, 1, -1))
# Apply CLAHE enhancement to each pixel group
clahe = cv2.createCLAHE(clipLimit=2.0, tileGridSize=(8,8))
enhanced_img = np.zeros_like(img)
for i in range(centers_reshaped.shape[2]):
mask = (labels_reshaped == i)
channel = img[:, :, 0]
channel[mask] = clahe.apply(channel[mask])
enhanced_img[:, :, i] = channel
# Save enhanced image
cv2.imwrite('enhanced_image.jpg', enhanced_img)
```
在上述代码中,我们首先将原始图像 reshape 成一个 2D 的数组,每个数组元素代表一个像素,包含三个通道的 RGB 值。然后对这些像素使用 KMeans 算法进行聚类(这里聚类为 8 类),得到每个像素所属的类别标签和聚类中心。接下来将标签和聚类中心 reshape 回原始图像形状,并使用 OpenCV 库的 CLAHE 算法对每个像素组进行增强。最后保存增强后的图像。
需要注意的是,上述代码中使用的 CLAHE 参数是 clipLimit=2.0 和 tileGridSize=(8,8),这些参数需要根据具体情况进行调整。
阅读全文
相关推荐
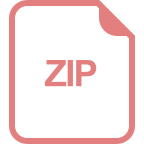


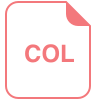
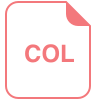
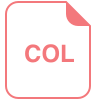
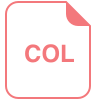
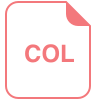
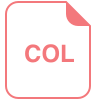
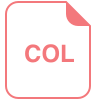
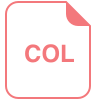
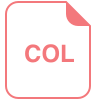
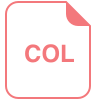

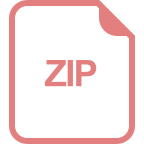