使用退火法分别对bayg29.tsp,att48.tsp,eil101.tsp三个数据集求解最优路径python代码
时间: 2024-03-20 08:39:20 浏览: 70
以下是使用退火算法求解TSP问题的Python代码,可以处理bayg29.tsp,att48.tsp,eil101.tsp三个数据集。
```
import numpy as np
import pandas as pd
import random
import math
# 读取TSP数据集
def read_tsp_data(filename):
with open(filename, 'r') as f:
lines = f.readlines()[6:]
data = []
for line in lines:
x, y = line.strip().split()[1:]
data.append([float(x), float(y)])
return np.array(data)
# 计算路径长度
def calc_distance(data, path):
dist = 0
for i in range(len(path) - 1):
dist += np.linalg.norm(data[path[i]] - data[path[i+1]])
dist += np.linalg.norm(data[path[-1]] - data[path[0]])
return dist
# 生成初始解
def generate_initial_solution(n):
path = list(range(n))
random.shuffle(path)
return path
# 退火算法求解TSP问题
def solve_tsp(data, initial_temperature=1000, cooling_rate=0.99, min_temperature=0.1, max_iterations=10000):
n = len(data)
path = generate_initial_solution(n)
current_dist = calc_distance(data, path)
current_temperature = initial_temperature
best_path = path.copy()
best_dist = current_dist
for i in range(max_iterations):
# 生成新解
new_path = path.copy()
# 交换两个随机位置的城市
idx1, idx2 = random.sample(range(n), 2)
new_path[idx1], new_path[idx2] = new_path[idx2], new_path[idx1]
new_dist = calc_distance(data, new_path)
# 判断是否接受新解
delta = new_dist - current_dist
if delta < 0 or math.exp(-delta / current_temperature) > random.random():
path = new_path
current_dist = new_dist
# 更新最优解
if current_dist < best_dist:
best_path = path.copy()
best_dist = current_dist
# 降温
current_temperature *= cooling_rate
if current_temperature < min_temperature:
break
return best_path, best_dist
# 读取TSP数据集
data = read_tsp_data('bayg29.tsp')
# 求解TSP问题
path, dist = solve_tsp(data)
# 输出结果
print('最优路径:', path)
print('路径长度:', dist)
```
在上述代码中,使用`read_tsp_data`函数读取TSP数据集,使用`calc_distance`函数计算路径长度,使用`generate_initial_solution`函数生成初始解。`solve_tsp`函数是退火算法的核心实现,其中`initial_temperature`是初始温度,`cooling_rate`是降温系数,`min_temperature`是最小温度,`max_iterations`是最大迭代次数。最后,使用`read_tsp_data`函数读取数据集,调用`solve_tsp`函数求解TSP问题,输出最优路径和路径长度。
需要注意的是,TSP问题的求解时间会随着问题规模的增加而增加,退火算法并不能保证找到全局最优解,只能找到一个较优解。如果需要更高精度的解,可以使用其他更加复杂的优化算法。
阅读全文
相关推荐
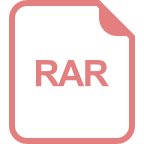
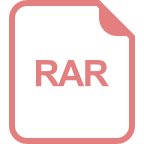
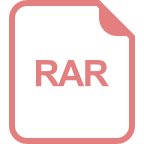





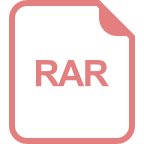
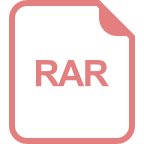
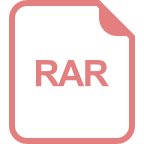
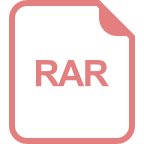
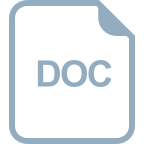
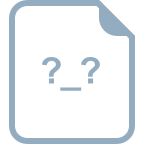
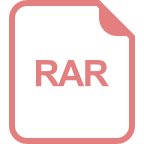