if request.method == "POST": result = [{"Result": 0, "Message": "指定库位不存在!", "LocationId": "L0023B1002", "LightColor": 96, "Twinkle": 0, "IsLocked": 0, "IsMustCollect": 0, "Quantity": 0, "SubText": None, "BatchCode": None, "Name": None, "R1": None, "R2": None, "R3": None, "SubTitle": None, "Title": None, "Unit": None, "RelateToTower": True }] req = reqparse.RequestParser() req.add_argument('LocationId',type=str, required=True) req.add_argument('LightColor',type=int, required=True) req.add_argument('Twinkle',type=int, required=True) req.add_argument('IsLocked',type=int, required=True) req.add_argument('IsMustCollect',type=int, required=True) req.add_argument('Quantity',type=int, required=True) args = req.parse_args() if args['LightColor'] not in color_json: pass else: material_location_code = MaterialLocation.query.filter(MaterialLocation.location_code == args['LocationId']) if not material_location_code: return jsonify({ 'Result': 0, 'Message': None, 'Details': result }) return jsonify({ "Result": 1, "Message": None, "Details": [] })
时间: 2023-05-22 11:07:10 浏览: 67
这是一个Python的Web应用程序请求,包含参数的解析以及对数据库的查询。如果请求中的LightColor参数不在color_json中,则程序会什么都不做。如果MaterialLocation表中不存在指定的location_code,则返回包含错误信息的JSON响应。否则,返回一个空列表作为详情。
相关问题
public WebMgrResponse GetLocationProperty(LocationPropertyQueryDto data) { WebMgrResponse webMgrResponse = new WebMgrResponse(); //查询信息 var locationDataList = string.IsNullOrEmpty(data.WareHouseCode) ? locationManager.GetList() : locationManager.GetList(d => d.WareHouseCode == data.WareHouseCode); var dtoList = locationDataList.Adapt<List<LocationPropertyDto>>(); for (int i = 0; i < dtoList.Count; i++) { var dto = dtoList[i]; dto.TargetWareHouseCode = dto.LocationCode.GetLocationMember("目标仓库"); dto.SourceWareHouseCode = dto.LocationCode.GetLocationMember("源仓库"); } webMgrResponse.code = 20000; webMgrResponse.data = Newtonsoft.Json.JsonConvert.SerializeObject(dtoList); webMgrResponse.message = "成功"; return webMgrResponse; }
这段代码是一个 C# 方法,接收一个名为 LocationPropertyQueryDto 的数据对象作为参数,返回一个 WebMgrResponse 对象。主要功能是查询库位的属性信息,并将查询结果封装成一个包含响应码、响应数据和响应消息的 WebMgrResponse 对象返回。
具体实现过程如下:
1. 创建一个 WebMgrResponse 对象,用于存储响应信息。
2. 根据传入的 LocationPropertyQueryDto 对象中的 WareHouseCode 属性值是否为空来判断是否需要过滤查询结果,如果 WareHouseCode 为空,则获取所有库位信息,否则根据 WareHouseCode 过滤查询结果。
3. 将查询结果转换成 LocationPropertyDto 列表对象,然后遍历列表中的每一个 LocationPropertyDto 对象。
4. 对于每一个 LocationPropertyDto 对象,通过调用 LocationCode 属性的 GetLocationMember 方法获取目标仓库和源仓库的信息,并将其分别存储到 TargetWareHouseCode 和 SourceWareHouseCode 属性中。
5. 将转换后的 LocationPropertyDto 列表对象序列化成 JSON 字符串,并将其存储到 WebMgrResponse 对象的 data 属性中。
6. 将响应码和响应消息分别存储到 WebMgrResponse 对象的 code 和 message 属性中。
7. 返回 WebMgrResponse 对象。
总体来说,这段代码实现了查询库位属性信息并进行简单处理后返回的功能。
2.更新库位信息 Post http://localhost:63300/api/Pms/UpdateLocationProperty 发送Body例子 { "LocationCode": "原材料1", "Status": 2 } { "LocationCode": "原材料1", "Status": 1 } Status状态 1:满 2:空
根据您提供的信息,这是一个使用 HTTP POST 方法向 `http://localhost:63300/api/Pms/UpdateLocationProperty` 发送 JSON 格式数据的例子。其中 JSON 数据包含两个属性 `LocationCode` 和 `Status`,用于更新库位信息。属性 `LocationCode` 表示库位编号,属性 `Status` 表示库位状态,可选值为 1 和 2,分别表示满和空。
您需要编写一个能够接收 POST 请求的 API 接口,从请求中读取 JSON 数据,然后根据 `LocationCode` 属性更新对应库位的状态。具体实现方式可以参考您所使用的 Web 框架的文档或示例代码。
相关推荐
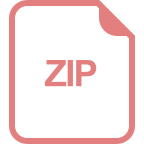













