import numpy as np import matplotlib.pyplot as plt def ricker(f, length, dt): t = np.arange(-length/2,(length-dt)/2, dt) y = (1.0 - 2.0*(np.pi**2)*(f**2)*(t**2)) * np.exp(-(np.pi**2)*(f**2)*(t**2)) return t, y def plot_data(ax, data, time, title, ylabel=''): ax.plot(data, time) ax.xaxis.set_ticks_position('top') ax.invert_yaxis() ax.set_title(title, fontsize=12) ax.set_ylabel(ylabel, fontsize=12) # Parameters Frequency = 20 length = 0.128 dt = 0.001 t0, w0 = ricker(Frequency, length, dt) rho = np.array([1.6, 1.8, 2.3, 2.4, 2.8, 2.6, 3.0, 3.4]) v = np.array([1200, 1600, 1300, 2000, 4400, 4000, 5500, 8000]) depth = np.array([100, 200, 500, 1000, 1500, 2000, 3000, 5000]) L = np.zeros(8); Z = rho * v L = np.diff(Z) / (Z[:-1] + Z[1:]) t1 = np.arange(0, 2.75, dt) L1 = np.zeros(np.size(t1)) t = np.cumsum(np.concatenate(([depth[0]*2/v[0]], np.diff(depth)*2/v[1:]))) L1[np.round(t/dt).astype(int)] = L syn = np.convolve(L1, w0, 'same') fig, axes = plt.subplots(1, 3, figsize=(20, 15), dpi=300) plot_data(axes[0], w0, t0, "Amplitude", "Time(s)") plot_data(axes[1], L1, t1, "Reflection coefficient", "Two-way travel time(s)") plot_data(axes[2], syn, t1, "Amplitude", "Two-way travel time(s)") fig.suptitle('Eight-layer synthetic seismogram', fontsize=18) plt.tight_layout() plt.show()中出现了 L1[np.round(t/dt).astype(int)] = L ValueError: shape mismatch: value array of shape (7,) could not be broadcast to indexing result of shape (8,)帮我改正
时间: 2023-09-14 17:07:14 浏览: 100
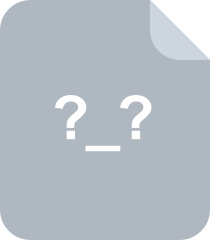
import numpy as np import matplotlib.pyplot as plt import math
在代码的第19行,你使用了np.zeros函数创建了一个长度为np.size(t1)的零数组L1。然后在第21行中,你使用了np.round和astype函数将深度数组t转换为索引数组,并将L数组的值赋给L1的这些索引位置。这里出现了错误,因为L1数组的长度是np.size(t1),而L数组的长度是8,它们的长度不匹配。因此,你需要将L1数组的长度更改为8,或者使用与深度数组长度相同的长度来创建L数组。可以在代码的第12行添加一个变量thickness,表示每个层的厚度,并在第16行中使用thickness数组替换depth数组。这样,L数组的长度将与thickness数组的长度相同,并且L1数组可以使用与thickness数组相同的长度创建。修改后的代码如下所示:
```python
import numpy as np
import matplotlib.pyplot as plt
def ricker(f, length, dt):
t = np.arange(-length/2,(length-dt)/2, dt)
y = (1.0 - 2.0*(np.pi**2)*(f**2)*(t**2)) * np.exp(-(np.pi**2)*(f**2)*(t**2))
return t, y
def plot_data(ax, data, time, title, ylabel=''):
ax.plot(data, time)
ax.xaxis.set_ticks_position('top')
ax.invert_yaxis()
ax.set_title(title, fontsize=12)
ax.set_ylabel(ylabel, fontsize=12)
# Parameters
Frequency = 20
length = 0.128
dt = 0.001
t0, w0 = ricker(Frequency, length, dt)
rho = np.array([1.6, 1.8, 2.3, 2.4, 2.8, 2.6, 3.0, 3.4])
v = np.array([1200, 1600, 1300, 2000, 4400, 4000, 5500, 8000])
thickness = np.array([100, 100, 300, 500, 500, 500, 1000, 1500])
L = np.zeros(len(thickness))
Z = rho * v
L = np.diff(Z) / (Z[:-1] + Z[1:])
t1 = np.arange(0, 2.75, dt)
L1 = np.zeros(len(thickness))
t = np.cumsum(np.concatenate(([thickness[0]*2/v[0]], np.diff(thickness)*2/v[1:])))
L1[np.round(t/dt).astype(int)] = L
syn = np.convolve(L1, w0, 'same')
fig, axes = plt.subplots(1, 3, figsize=(20, 15), dpi=300)
plot_data(axes[0], w0, t0, "Amplitude", "Time(s)")
plot_data(axes[1], L1, t1, "Reflection coefficient", "Two-way travel time(s)")
plot_data(axes[2], syn, t1, "Amplitude", "Two-way travel time(s)")
fig.suptitle('Eight-layer synthetic seismogram', fontsize=18)
plt.tight_layout()
plt.show()
```
现在,代码应该可以正常运行,没有ValueError:shape mismatch错误。
阅读全文
相关推荐






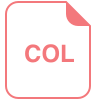


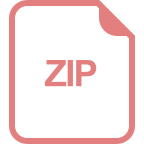
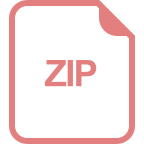