请写一个python代码,弹出一个画板,我可以用鼠标指针绘制圆,正方形,长方形等二维图形,其中我可以选择绘制固定图形和移动图形,移动图形会按照基本物理规律,收到相应的重力弹力摩擦力,发生坠落,并相应表现在画板上
时间: 2023-10-05 07:12:42 浏览: 84
以下是一个基于 Pygame 库的 Python 代码,可以实现绘制固定图形和移动图形,并按照物理规律进行运动和碰撞检测,相应地在画板上显示:
```python
import pygame
import random
# 初始化 Pygame 库
pygame.init()
# 定义常数
FPS = 60 # 帧率
WIDTH = 800 # 画板宽度
HEIGHT = 600 # 画板高度
GRAVITY = 0.2 # 重力加速度
ELASTICITY = 0.8 # 弹力系数
FRICTION = 0.9 # 摩擦系数
# 创建画板对象
screen = pygame.display.set_mode((WIDTH, HEIGHT))
# 定义颜色常数
BLACK = (0, 0, 0)
WHITE = (255, 255, 255)
RED = (255, 0, 0)
GREEN = (0, 255, 0)
BLUE = (0, 0, 255)
# 定义基本图形类
class Shape:
def __init__(self, x, y, color):
self.x = x
self.y = y
self.color = color
def draw(self, surface):
pass
def move(self, dx, dy):
self.x += dx
self.y += dy
# 定义圆形类
class Circle(Shape):
def __init__(self, x, y, radius, color):
super().__init__(x, y, color)
self.radius = radius
self.vx = 0
self.vy = 0
def draw(self, surface):
pygame.draw.circle(surface, self.color, (int(self.x), int(self.y)), self.radius)
def move(self, dx, dy):
super().move(dx, dy)
self.vx += dx
self.vy += dy
def update(self):
self.move(self.vx, self.vy)
self.vy += GRAVITY
if self.x - self.radius < 0:
self.x = self.radius
self.vx = -self.vx * ELASTICITY
if self.x + self.radius > WIDTH:
self.x = WIDTH - self.radius
self.vx = -self.vx * ELASTICITY
if self.y - self.radius < 0:
self.y = self.radius
self.vy = -self.vy * ELASTICITY
if self.y + self.radius > HEIGHT:
self.y = HEIGHT - self.radius
self.vy = -self.vy * ELASTICITY * FRICTION
# 定义正方形类
class Square(Shape):
def __init__(self, x, y, size, color):
super().__init__(x, y, color)
self.size = size
def draw(self, surface):
pygame.draw.rect(surface, self.color, (self.x, self.y, self.size, self.size))
# 定义长方形类
class Rectangle(Shape):
def __init__(self, x, y, width, height, color):
super().__init__(x, y, color)
self.width = width
self.height = height
def draw(self, surface):
pygame.draw.rect(surface, self.color, (self.x, self.y, self.width, self.height))
# 创建图形列表
shapes = []
# 创建时钟对象
clock = pygame.time.Clock()
# 循环检测事件
while True:
# 限制帧率
clock.tick(FPS)
# 处理事件
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
exit()
elif event.type == pygame.MOUSEBUTTONDOWN:
# 鼠标左键绘制圆形
if event.button == 1:
x, y = event.pos
radius = random.randint(10, 50)
color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
circle = Circle(x, y, radius, color)
shapes.append(circle)
# 鼠标右键绘制正方形
elif event.button == 3:
x, y = event.pos
size = random.randint(10, 50)
color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
square = Square(x, y, size, color)
shapes.append(square)
# 鼠标中键绘制长方形
elif event.button == 2:
x, y = event.pos
width = random.randint(20, 80)
height = random.randint(10, 40)
color = (random.randint(0, 255), random.randint(0, 255), random.randint(0, 255))
rectangle = Rectangle(x, y, width, height, color)
shapes.append(rectangle)
# 更新图形位置和状态
for shape in shapes:
if isinstance(shape, Circle):
shape.update()
elif isinstance(shape, Rectangle) or isinstance(shape, Square):
shape.move(0, GRAVITY)
if shape.x < 0:
shape.x = 0
if shape.x + shape.width > WIDTH:
shape.x = WIDTH - shape.width
if shape.y + shape.height > HEIGHT:
shape.y = HEIGHT - shape.height
# 绘制背景
screen.fill(WHITE)
# 绘制图形
for shape in shapes:
shape.draw(screen)
# 刷新画板
pygame.display.flip()
```
这段代码使用 Pygame 库实现了绘制圆形、正方形和长方形的功能,并根据物理规律对图形进行运动和碰撞检测,相应地在画板上显示。可以根据需要调整常数和图形类的实现来模拟不同的情况。
相关推荐
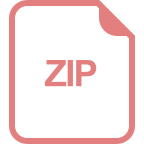
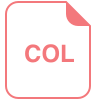
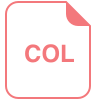
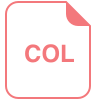
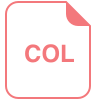
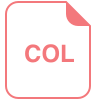









