在js重构reduce函数
时间: 2023-07-04 19:16:29 浏览: 47
可以使用循环实现 reduce 函数的功能,如下所示:
```javascript
function reduce(arr, callback, initialVal) {
let accumulator = initialVal === undefined ? undefined : initialVal;
for (let i = 0; i < arr.length; i++) {
if (accumulator !== undefined) {
accumulator = callback.call(undefined, accumulator, arr[i], i, arr);
} else {
accumulator = arr[i];
}
}
return accumulator;
}
```
这个函数接受三个参数,分别是需要进行 reduce 操作的数组 arr,一个回调函数 callback 和可选的初始值 initialVal。在函数内部,我们定义了一个累加器 accumulator,并根据初始值的有无来判断是否需要将它初始化为 undefined。接着,我们使用一个 for 循环遍历数组,如果累加器不是 undefined,则调用回调函数对累加器和当前元素进行计算,否则直接将当前元素作为累加器的值。最后返回累加器的值即可。
相关问题
js原型重构reduce
在 JavaScript 中,reduce() 是一个高阶函数,它接受一个回调函数作为参数,用于对数组中的每个元素进行操作并返回一个累加器的结果。在使用 reduce() 函数时,可以通过原型重构的方式来扩展其功能。
以下是一个示例代码:
```
Array.prototype.myReduce = function(callback, initialValue) {
let accumulator = initialValue === undefined ? undefined : initialValue;
for (let i = 0; i < this.length; i++) {
if (accumulator !== undefined) {
accumulator = callback.call(undefined, accumulator, this[i], i, this);
} else {
accumulator = this[i];
}
}
return accumulator;
}
```
在这个示例中,我们通过将 reduce() 函数添加到 Array 的原型中来扩展其功能。我们使用了类似于原始 reduce() 函数的实现方式,但是添加了一个新的参数 initialValue,用于设置初始累加器的值。
通过这种方式,我们可以像使用原始的 reduce() 函数一样使用自定义的 myReduce() 函数,并且可以将其用作数组的方法。
在js中利用原型的特性重构filter函数
好的,我理解了你的问题。下面是利用原型的特性重构filter函数的示例代码:
```javascript
Array.prototype.myFilter = function(callback) {
let filteredArr = [];
for(let i=0; i<this.length; i++) {
if(callback(this[i], i, this)) {
filteredArr.push(this[i]);
}
}
return filteredArr;
}
```
在这个示例中,我们使用原型对象给Array添加了一个新的方法`myFilter`。`myFilter`方法接受一个回调函数作为参数,该回调函数接受3个参数:当前元素的值,当前元素的索引,以及被调用的数组对象本身。
在`myFilter`方法内部,我们遍历数组中的每个元素,并分别调用回调函数进行判断。如果回调函数返回`true`,则将当前元素添加到一个新数组`filteredArr`中。最后,返回`filteredArr`数组。
请注意,这只是一个简单的示例,实际上JavaScript的内置`Array.prototype.filter`方法更为复杂,支持多种用法和参数。
相关推荐
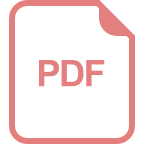












