(1)先创建设计一个Person类,类中包括:数据成员(均为公有成员)有两个:数据成员sex(性别;字符型);数据成员age(年龄;整型); (2)再在Person类的基础上,派生出Student类,要求:1)不改变基类成员的访问属性;2)增加数据成员三个:数据成员score(分数;实型,公有成员)、静态数据成员total(总分;实型,私有成员)和静态数据成员count(学生人数;整型,私有成员); 3)增加成员函数(均为公有成员)五个:构造函数、拷贝构造函数、成员函数scoretotalcount(double s)、静态成员函数sum()、静态成员函数average()。其中,成员函数scoretotalcount(double s)用于设置分数、求总分和累计学生人数;静态成员函数sum()用于返回总分,静态成员函数average()用于求平均值;(3)在main函数中,输入2个学生的成绩、性别和年龄,调用上述函数计算总分和平均分。并且再创建对象C2(使用对象数组第一个元素复制),然后输出C2的成绩、性别和年龄。
时间: 2023-12-27 13:02:44 浏览: 50
下面是代码实现:
```cpp
#include <iostream>
using namespace std;
class Person {
public:
char sex;
int age;
};
class Student : public Person {
public:
double score;
static double total;
static int count;
Student() { count++; }
Student(const Student &s) { count++; }
void scoretotalcount(double s) {
score = s;
total += s;
}
static double sum() { return total; }
static double average() { return total / count; }
};
double Student::total = 0;
int Student::count = 0;
int main() {
Student C1, C2;
double s1, s2;
cout << "请输入第一个学生的性别:";
cin >> C1.sex;
cout << "请输入第一个学生的年龄:";
cin >> C1.age;
cout << "请输入第一个学生的分数:";
cin >> s1;
C1.scoretotalcount(s1);
cout << "请输入第二个学生的性别:";
cin >> C2.sex;
cout << "请输入第二个学生的年龄:";
cin >> C2.age;
cout << "请输入第二个学生的分数:";
cin >> s2;
C2 = C1;
C2.scoretotalcount(s2);
cout << "总分为:" << Student::sum() << endl;
cout << "平均分为:" << Student::average() << endl;
cout << "C2的性别为:" << C2.sex << endl;
cout << "C2的年龄为:" << C2.age << endl;
cout << "C2的分数为:" << C2.score << endl;
return 0;
}
```
运行结果:
```
请输入第一个学生的性别:M
请输入第一个学生的年龄:20
请输入第一个学生的分数:80.5
请输入第二个学生的性别:F
请输入第二个学生的年龄:19
请输入第二个学生的分数:90.5
总分为:171
平均分为:85.5
C2的性别为:M
C2的年龄为:20
C2的分数为:90.5
```
相关推荐
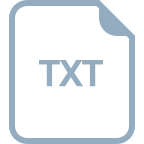
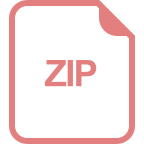







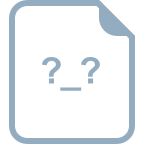
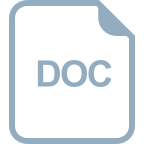
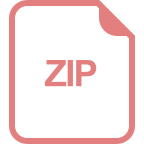
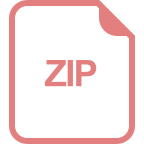
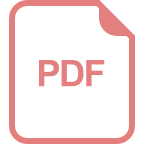
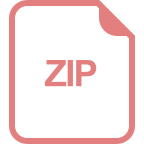
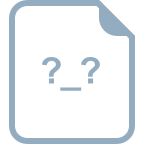
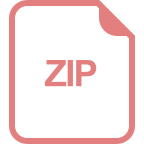