定义人员类Person,它派生出学生类Student和教师类Teacher,学生类和教师类又共同派生出助教类RA。在主函数中使用构造函数初始化助教类对象的信息并打印输出。 公有继承实现 人员类有姓名、性别、出生年月 学生类有学号、专业信息, 教师类有所属学校信息
时间: 2024-02-21 09:00:03 浏览: 113
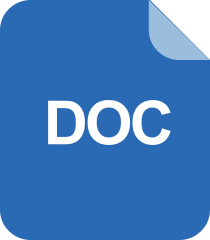
由Person类派生出学生类Student和教师类Teacher
这个问题和上一个问题类似,只是稍微修改了一下类成员的定义。我帮你写出代码示例:
```
#include <iostream>
#include <string>
using namespace std;
// 定义人员类Person
class Person {
protected:
string name;
string sex;
string birth;
public:
Person(string n, string s, string b) : name(n), sex(s), birth(b) {}
};
// 定义学生类Student,公有继承自Person
class Student : public Person {
private:
string student_id;
string major;
public:
Student(string n, string s, string b, string id, string m) :
Person(n, s, b), student_id(id), major(m) {}
};
// 定义教师类Teacher,公有继承自Person
class Teacher : public Person {
private:
string school;
public:
Teacher(string n, string s, string b, string sch) :
Person(n, s, b), school(sch) {}
};
// 定义助教类RA,公有继承自Student和Teacher
class RA : public Student, public Teacher {
public:
RA(string n, string s, string b, string id, string m, string sch) :
Student(n, s, b, id, m), Teacher(n, s, b, sch) {}
};
int main() {
// 使用构造函数初始化助教类对象信息
RA ra("Zhang San", "M", "1998-09-26", "2021001", "Computer Science", "University of Science and Technology");
// 打印输出助教类对象信息
cout << "Name: " << ra.Student::name << endl;
cout << "Sex: " << ra.Student::sex << endl;
cout << "Birth: " << ra.Student::birth << endl;
cout << "Student ID: " << ra.student_id << endl;
cout << "Major: " << ra.major << endl;
cout << "School: " << ra.Teacher::school << endl;
return 0;
}
```
在上面的代码中,我们重新定义了Person、Student、Teacher和RA四个类,其中Person类是基类,Student和Teacher类公有继承自Person类,RA类则公有继承自Student和Teacher类。在主函数中,我们使用构造函数初始化助教类对象ra的信息,并打印输出。注意,我们使用作用域运算符来访问从两个父类继承下来的成员变量name、sex、birth和school。
阅读全文
相关推荐
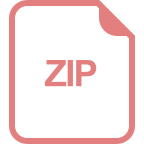
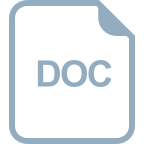




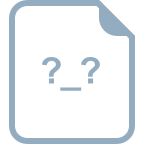
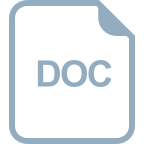
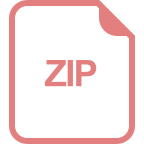
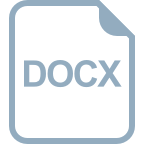
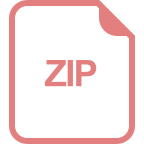