用c++把问题都写出答案
时间: 2024-10-21 18:04:48 浏览: 15
为了完成《实验报告3(算法)》中的题目,以下是C++代码实现:
### 题目1:水果销售情况明细表
```cpp
#include <iostream>
#include <map>
#include <vector>
#include <algorithm>
using namespace std;
struct Sale {
string name;
string origin;
int quantity;
};
bool compare(const pair<string, vector<Sale>>& a, const pair<string, vector<Sale>>& b) {
return a.first < b.first;
}
int main() {
int N;
cin >> N;
while (N--) {
map<string, vector<Sale>> sales;
int M;
cin >> M;
for (int i = 0; i < M; ++i) {
string name, origin;
int quantity;
cin >> name >> origin >> quantity;
sales[origin].push_back({name, origin, quantity});
}
for (auto& entry : sales) {
sort(entry.second.begin(), entry.second.end(), [](const Sale& a, const Sale& b) {
return a.name < b.name;
});
}
for (auto& entry : sales) {
cout << entry.first << endl;
for (const auto& sale : entry.second) {
cout << sale.name << ' ' << sale.quantity << endl;
}
}
if (N > 0) {
cout << endl;
}
}
return 0;
}
```
### 题目2:火车进出站问题
```cpp
#include <iostream>
#include <stack>
#include <string>
using namespace std;
bool canArrange(int n, const string& inOrder, const string& outOrder) {
stack<int> railway;
int inIndex = 0, outIndex = 0;
while (outIndex < n) {
// Push trains into the railway until we find the next train that needs to leave
while (inIndex < n && (railway.empty() || railway.top() != outOrder[outIndex] - '0')) {
railway.push(inOrder[inIndex++] - '0');
}
// If the top of the stack matches the next train to leave, pop it
if (!railway.empty() && railway.top() == outOrder[outIndex] - '0') {
railway.pop();
outIndex++;
} else {
return false;
}
}
return true;
}
int main() {
string inOrder, outOrder;
int n;
while (cin >> n >> inOrder >> outOrder) {
if (canArrange(n, inOrder, outOrder)) {
cout << "Yes." << endl;
stack<int> railway;
int inIndex = 0, outIndex = 0;
while (outIndex < n) {
while (inIndex < n && (railway.empty() || railway.top() != outOrder[outIndex] - '0')) {
railway.push(inOrder[inIndex++] - '0');
cout << "in" << endl;
}
if (!railway.empty() && railway.top() == outOrder[outIndex] - '0') {
railway.pop();
cout << "out" << endl;
outIndex++;
}
}
} else {
cout << "No." << endl;
}
cout << "FINISH" << endl;
}
return 0;
}
```
### 题目3:看病要排队
```cpp
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
struct Patient {
int id;
int priority;
};
class PriorityQueue {
public:
void push(int id, int priority) {
data.push_back({id, priority});
push_heap(data.begin(), data.end(), greater<>());
}
bool empty() {
return data.empty();
}
Patient top() {
return data.front();
}
void pop() {
pop_heap(data.begin(), data.end(), greater<>());
data.pop_back();
}
private:
vector<Patient> data;
};
int main() {
int N;
while (cin >> N) {
PriorityQueue queues[3];
int eventCount = 0;
for (int i = 0; i < N; ++i) {
string command;
cin >> command;
if (command == "IN") {
int doctor, priority;
cin >> doctor >> priority;
queues[doctor - 1].push(++eventCount, priority);
} else if (command == "OUT") {
int doctor;
cin >> doctor;
if (!queues[doctor - 1].empty()) {
cout << queues[doctor - 1].top().id << endl;
queues[doctor - 1].pop();
} else {
cout << "EMPTY" << endl;
}
}
}
}
return 0;
}
```
### 提交说明
- 将上述代码保存为单独的C++源文件,并在实验过程中记录中详细记录每一步的操作和调试过程。
- 完成后将所有代码整合到实验报告中,并按照要求生成PDF文件。
- 按照以下格式命名文件:
```
年级专业班级_学号_姓名_实验次数_实验名称.pdf
```
例如:
```
23计科7班_23052500_张三_实验1_算法设计-STL应用二.pdf
```
希望这些代码和指导对你有所帮助!如果有任何问题,请随时提问。
阅读全文
相关推荐
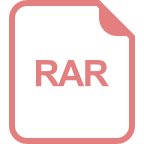
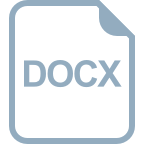
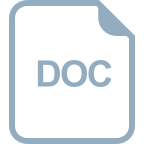

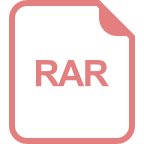
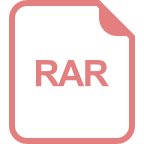
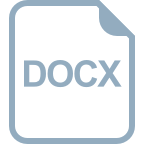
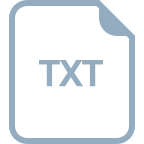
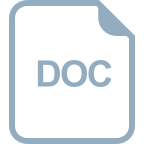
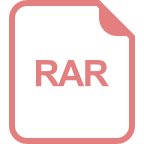
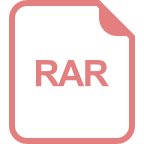
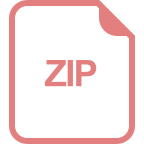
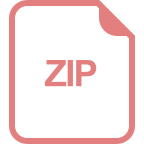
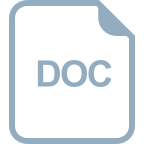




