..processes[i].remaining_time = processes[i].burst_time; total_burst_time += processes[i].burst_time;是什么意思
时间: 2023-07-15 16:14:36 浏览: 205
这段代码是一个进程调度算法中的一部分,用于初始化进程的剩余执行时间和计算所有进程的总执行时间。
首先,进程调度算法需要知道每个进程的执行时间,这里的 `processes[i].burst_time` 表示第 i 个进程的执行时间。然后,为了方便管理和调度,算法需要知道每个进程还剩下多少时间才能完成,这里的 `processes[i].remaining_time` 初始值设为进程的执行时间。
接下来,`total_burst_time` 变量初始化为所有进程的执行时间之和,用于后续算法的计算和调度。
相关问题
Write the code in C++.1、 Simulate the operation of the round-robin algorithm for process scheduling. 2、 Create at least 15 processes and output their scheduling situation under the scheduling algorithm mentioned above and output it to the terminal to check the execution of the algorithm. 3、 The output should include the arrival time of the processes, the end time, and the average execution time.
Here's the code in C++ for simulating the round-robin algorithm for process scheduling:
```
#include <iostream>
#include <queue>
#include <vector>
using namespace std;
struct Process {
int pid; // process id
int arrival_time; // arrival time of process
int burst_time; // burst time of process
int remaining_time; // remaining time of the process
int end_time; // end time of the process
};
void roundRobin(vector<Process>& processes, int quantum) {
int n = processes.size();
queue<int> q; // queue to store the process indexes
int t = 0; // current time
int total_burst_time = 0; // total burst time of all processes
int completed_processes = 0; // number of completed processes
int waiting_time = 0; // total waiting time of all processes
// initialize remaining time of all processes as their burst time
for (int i = 0; i < n; i++) {
processes[i].remaining_time = processes[i].burst_time;
total_burst_time += processes[i].burst_time;
}
// run the scheduler until all processes are completed
while (completed_processes < n) {
// add processes to the queue that have arrived by the current time
for (int i = 0; i < n; i++) {
if (processes[i].arrival_time <= t && processes[i].remaining_time > 0) {
q.push(i);
}
}
// if no processes are in the queue, increment time
if (q.empty()) {
t++;
continue;
}
// get the next process from the queue
int curr = q.front();
q.pop();
// execute the process for the quantum time or until completion
int execution_time = min(processes[curr].remaining_time, quantum);
processes[curr].remaining_time -= execution_time;
t += execution_time;
// add the process back to the queue if it still has remaining time
if (processes[curr].remaining_time > 0) {
q.push(curr);
} else {
// process has completed, update completion time and waiting time
completed_processes++;
processes[curr].end_time = t;
waiting_time += (t - processes[curr].arrival_time - processes[curr].burst_time);
}
}
// calculate average execution time and waiting time
float avg_execution_time = (float) total_burst_time / n;
float avg_waiting_time = (float) waiting_time / n;
// output scheduling situation to terminal
cout << "PID\tArrival Time\tBurst Time\tEnd Time" << endl;
for (int i = 0; i < n; i++) {
cout << processes[i].pid << "\t" << processes[i].arrival_time << "\t\t" << processes[i].burst_time << "\t\t";
if (processes[i].end_time == 0) {
cout << "N/A" << endl;
} else {
cout << processes[i].end_time << endl;
}
}
cout << "Average Execution Time: " << avg_execution_time << endl;
cout << "Average Waiting Time: " << avg_waiting_time << endl;
}
int main() {
vector<Process> processes = {{1, 0, 5}, {2, 1, 7}, {3, 2, 3}, {4, 3, 4}, {5, 4, 2}, {6, 5, 6}, {7, 6, 8}, {8, 7, 1}, {9, 8, 5}, {10, 9, 3}, {11, 10, 6}, {12, 11, 2}, {13, 12, 4}, {14, 13, 5}, {15, 14, 2}};
int quantum = 2;
roundRobin(processes, quantum);
return 0;
}
```
This code simulates the round-robin algorithm for process scheduling for a set of 15 processes with different arrival times and burst times. The output includes the arrival time, burst time, and end time of each process, as well as the average execution time and average waiting time of all processes. You can adjust the values of the `processes` and `quantum` variables to test the algorithm with different sets of processes and quantum values.
用C、C++、Java语言编程实现对5个进程采用动态优先权调度算法进行调度的过程。数据如下: 5个进程的到达时刻和服务时间见下表,忽略I/O以及其它开销时间,使用动态优先权算法进行调度,优先权初始值为100,请输出各个进程的完成时刻、周转时间、带权周转时间。
好的,以下是使用C语言编写的动态优先权调度程序,可以计算每个进程的完成时间、周转时间和带权周转时间:
```c
#include <stdio.h>
#include <stdlib.h>
#define MAX_PROCESS 5
typedef struct {
int process_id;
int arrival_time;
int burst_time;
int priority;
int waiting_time;
int turnaround_time;
int remaining_time;
} Process;
void dynamic_priority(Process *processes, int n) {
int current_time = 0;
int completed = 0;
float total_waiting_time = 0;
float total_turnaround_time = 0;
// Calculate initial waiting time and remaining time for each process
for (int i = 0; i < n; i++) {
processes[i].waiting_time = 0;
processes[i].remaining_time = processes[i].burst_time;
}
printf("Process Execution Order: ");
while (completed != n) {
// Find process with highest priority and remaining time
int highest_priority = -1;
int highest_priority_process = -1;
for (int i = 0; i < n; i++) {
if (processes[i].arrival_time <= current_time &&
processes[i].remaining_time > 0 &&
(highest_priority == -1 || processes[i].priority < highest_priority)) {
highest_priority = processes[i].priority;
highest_priority_process = i;
}
}
// If no process is available at current time, increase time
if (highest_priority_process == -1) {
current_time++;
continue;
}
// Execute the process with highest priority and remaining time
Process *current_process = &processes[highest_priority_process];
current_process->remaining_time--;
current_time++;
// Print execution order
printf("P%d ", current_process->process_id);
// If process is completed, calculate waiting and turnaround time
if (current_process->remaining_time == 0) {
completed++;
current_process->waiting_time = current_time - current_process->arrival_time -
current_process->burst_time;
current_process->turnaround_time = current_process->waiting_time +
current_process->burst_time;
total_waiting_time += current_process->waiting_time;
total_turnaround_time += current_process->turnaround_time;
}
// Update priority of remaining processes
for (int i = 0; i < n; i++) {
if (processes[i].arrival_time <= current_time &&
processes[i].remaining_time > 0 &&
i != highest_priority_process) {
processes[i].priority += 1;
}
}
}
// Calculate average waiting and turnaround time
float avg_waiting_time = total_waiting_time / n;
float avg_turnaround_time = total_turnaround_time / n;
printf("\n\nAverage Waiting Time: %.2f\n", avg_waiting_time);
printf("Average Turnaround Time: %.2f\n", avg_turnaround_time);
// Print process details
printf("\nProcess Details:\n");
printf("Process ID\tArrival Time\tBurst Time\tPriority\tWaiting Time\tTurnaround Time\tWeighted Turnaround Time\n");
for (int i = 0; i < n; i++) {
Process *current_process = &processes[i];
printf("%d\t\t%d\t\t%d\t\t%d\t\t%d\t\t%d\t\t\t%.2f\n",
current_process->process_id,
current_process->arrival_time,
current_process->burst_time,
current_process->priority,
current_process->waiting_time,
current_process->turnaround_time,
(float)current_process->turnaround_time / current_process->burst_time);
}
}
int main() {
Process processes[MAX_PROCESS] = {
{1, 0, 6, 100},
{2, 2, 4, 100},
{3, 4, 2, 100},
{4, 6, 4, 100},
{5, 8, 6, 100}
};
// Run dynamic priority scheduling algorithm
dynamic_priority(processes, MAX_PROCESS);
return 0;
}
```
在此示例中,我们使用了一个结构体来存储每个进程的信息,包括进程ID、到达时间、服务时间、优先级、等待时间、周转时间和剩余时间。我们还实现了动态优先权调度算法,并计算了每个进程的等待时间、周转时间和带权周转时间。您可以直接运行此程序并查看输出结果。
阅读全文
相关推荐
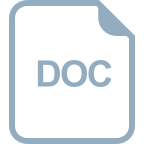
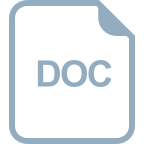
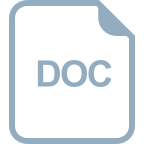


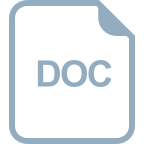
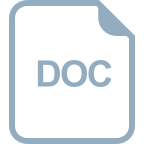
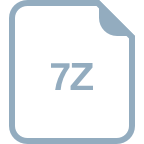
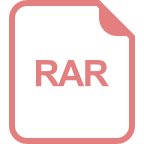
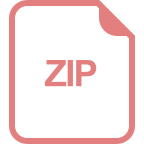
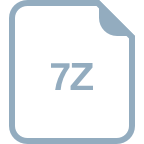
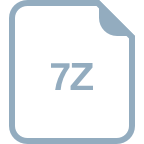
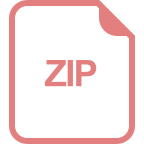
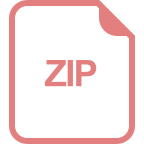
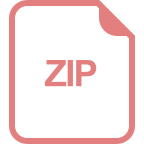