对CNN回归模型中指定卷积层实现Grad-CAM。PyTorch 生成代码
时间: 2024-02-06 08:11:47 浏览: 43
好的,以下是PyTorch生成的代码示例:
```python
import torch
import torch.nn as nn
import cv2
import numpy as np
# 定义CNN模型
class CNNModel(nn.Module):
def __init__(self):
super(CNNModel, self).__init__()
self.conv1 = nn.Conv2d(3, 32, kernel_size=3, stride=1, padding=1)
self.conv2 = nn.Conv2d(32, 64, kernel_size=3, stride=1, padding=1)
self.conv3 = nn.Conv2d(64, 128, kernel_size=3, stride=1, padding=1)
self.fc1 = nn.Linear(128 * 8 * 8, 1024)
self.fc2 = nn.Linear(1024, 10)
def forward(self, x):
x = nn.functional.relu(self.conv1(x))
x = nn.functional.max_pool2d(x, 2)
x = nn.functional.relu(self.conv2(x))
x = nn.functional.max_pool2d(x, 2)
x = nn.functional.relu(self.conv3(x))
x = nn.functional.max_pool2d(x, 2)
x = x.view(-1, 128 * 8 * 8)
x = nn.functional.relu(self.fc1(x))
x = self.fc2(x)
return x
# 加载模型和图像
model = CNNModel()
model.load_state_dict(torch.load('model.pth'))
img = cv2.imread('image.jpg')
img = cv2.cvtColor(img, cv2.COLOR_BGR2RGB)
img = cv2.resize(img, (32, 32))
img = np.transpose(img, (2, 0, 1))
img = torch.tensor(img).unsqueeze(0)
# 定义Grad-CAM方法
def grad_cam(model, img, layer_name):
# 获取指定卷积层
conv_layer = None
for name, module in model.named_modules():
if name == layer_name:
conv_layer = module
break
if conv_layer is None:
raise ValueError('指定的卷积层不存在')
# 前向传播
out = model(img)
_, pred = torch.max(out, 1)
# 反向传播
model.zero_grad()
one_hot = torch.zeros((1, out.size()[-1]), dtype=torch.float32)
one_hot[0][pred] = 1
out.backward(gradient=one_hot, retain_graph=True)
# 计算权重和特征图
weights = torch.mean(conv_layer.weight.grad, axis=(2, 3)).squeeze()
features = conv_layer(img)
features = nn.functional.relu(features).squeeze()
cam = torch.zeros(features.size()[-2:], dtype=torch.float32)
for i in range(weights.size()[0]):
cam += weights[i] * features[i]
# 归一化处理
cam = nn.functional.relu(cam)
cam = cam - torch.min(cam)
cam = cam / torch.max(cam)
# 转换为图像尺寸
cam = cv2.resize(cam.detach().numpy(), (img.size()[2], img.size()[3]))
cam = np.uint8(cam * 255)
return cam
# 调用Grad-CAM方法并可视化结果
cam = grad_cam(model, img, 'conv3')
heatmap = cv2.applyColorMap(cam, cv2.COLORMAP_JET)
result = cv2.addWeighted(cv2.cvtColor(img.squeeze().numpy().transpose(1, 2, 0), cv2.COLOR_RGB2BGR), 0.5, heatmap, 0.5, 0)
cv2.imwrite('result.jpg', result)
```
在上述代码中,我们定义了一个CNN模型,加载了预训练的权重和一张输入图像。然后,我们定义了一个Grad-CAM方法,该方法将模型、输入图像和指定的卷积层名称作为输入,返回一个热力图。最后,我们将热力图可视化为彩色图像,并将其保存到本地。
相关推荐
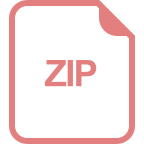
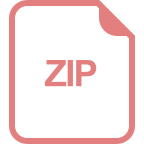
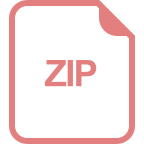














