基于CNN回归的GradCam可视化,pytorch生成代码
时间: 2024-02-13 15:01:10 浏览: 216
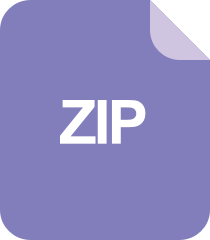
【源代码文件】pytorch-grad-cam源代码阅读和调试

以下是基于CNN回归的GradCam可视化的pytorch代码:
```python
import torch
import torch.nn.functional as F
import cv2
import numpy as np
# Load the pre-trained model
model = YourCNNRegressionModel()
# Define the image preprocessing function
def preprocess_image(img_path):
img = cv2.imread(img_path)
img = cv2.resize(img, (224, 224))
img = np.float32(img) / 255
img = np.transpose(img, (2, 0, 1))
img = np.expand_dims(img, axis=0)
return img
# Define the GradCam function
def GradCam(model, input_image, target_layer):
# Set model to evaluation mode
model.eval()
# Get the model's output and feature maps
output, features = model(input_image)
# Zero the gradients
model.zero_grad()
# Compute the gradients of the output with respect to the feature maps
output.backward(torch.ones_like(output))
# Get the gradients
gradients = target_layer.grad
# Get the weights of the target layer
weights = torch.mean(gradients, axis=(2, 3))
# Get the feature maps of the target layer
maps = features.detach()
# Compute the cam
cams = F.relu(torch.sum(weights[:, :, None, None] * maps, axis=1))
# Resize the cam to match the input image size
cams = F.interpolate(cams, size=input_image.shape[2:], mode='bilinear', align_corners=False)
# Normalize the cam
cams = F.normalize(cams, p=2, dim=1)
return cams
# Load the input image
img_path = 'path/to/image.jpg'
input_image = preprocess_image(img_path)
# Get the target layer
target_layer = model.layer_name
# Compute the GradCam
cam = GradCam(model, input_image, target_layer)
# Convert the cam to a numpy array
cam = cam.squeeze().cpu().numpy()
# Rescale the cam to be between 0 and 255
cam = np.uint8(255 * cam)
# Apply the cam as an overlay on the input image
overlay = cv2.applyColorMap(cam, cv2.COLORMAP_JET)
output_image = cv2.addWeighted(cv2.cvtColor(input_image.squeeze().transpose(1, 2, 0) * 255, cv2.COLOR_BGR2RGB), 0.5, overlay, 0.5, 0)
# Show the output image
cv2.imshow('GradCam', output_image)
cv2.waitKey(0)
cv2.destroyAllWindows()
```
在上面的代码中,你需要替换以下内容:
- `YourCNNRegressionModel()`:你使用的CNN回归模型。
- `preprocess_image()`:你的图像预处理函数。
- `model.layer_name`:你希望可视化的目标层的名称。可以在模型的定义中找到。
- `cv2.applyColorMap()`:你希望使用的颜色映射。在这里,我们使用了Jet颜色映射。
运行代码后,你应该能够看到一个窗口,显示输入图像和可视化的GradCam结果。
阅读全文
相关推荐
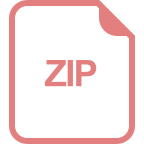















