这道题用到的知识点:卡车要装载一批货物,货物有3种商品:电视、冰箱和洗衣机。需要计算出大货车和小货车各自所装载的3种货物的总重量。编写一个Java应用程序:(1)要求有一个ComputeWeight接口,该接口中有一个方法:public double computeWeight(); (2)有3个实现该接口的类:Television、Refrigerator和WashMachine。这3个类通过实现接口ComputeWeight给出自重。 (3)有一个Truck类,该类用ComputeWeight接口类型的数组作为成员,那么该数组的元素就可以存放Television对象的引用、Refrigerator对象的引用或WashMachine对象的引用。 (4)程序能输出Truck对象所装载的货物的总重量。
时间: 2024-02-22 09:57:05 浏览: 65
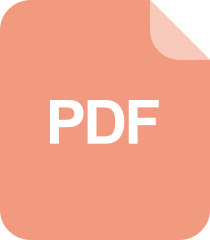
MIL_STD_1320_279.580.pdf
这道题主要考察Java中的接口和类的使用,下面是代码实现:
1. ComputeWeight接口:
```java
public interface ComputeWeight {
public double computeWeight();
}
```
2. Television、Refrigerator和WashMachine类的实现:
```java
public class Television implements ComputeWeight {
private double weight = 10.0; // 电视的自重为10.0kg
public double computeWeight() {
return weight;
}
}
public class Refrigerator implements ComputeWeight {
private double weight = 50.0; // 冰箱的自重为50.0kg
public double computeWeight() {
return weight;
}
}
public class WashMachine implements ComputeWeight {
private double weight = 30.0; // 洗衣机的自重为30.0kg
public double computeWeight() {
return weight;
}
}
```
3. Truck类:
```java
public class Truck {
private ComputeWeight[] goods;
public Truck(ComputeWeight[] goods) {
this.goods = goods;
}
public double getTotalWeight() {
double totalWeight = 0.0;
for (int i = 0; i < goods.length; i++) {
totalWeight += goods[i].computeWeight();
}
return totalWeight;
}
}
```
4. 调用Truck类获取货物总重量:
```java
public class Main {
public static void main(String[] args) {
ComputeWeight[] goods = new ComputeWeight[5];
goods[0] = new Television();
goods[1] = new Refrigerator();
goods[2] = new WashMachine();
goods[3] = new Television();
goods[4] = new WashMachine();
Truck truck = new Truck(goods);
System.out.println("Truck total weight: " + truck.getTotalWeight() + "kg");
}
}
```
输出结果:
```
Truck total weight: 130.0kg
```
阅读全文
相关推荐
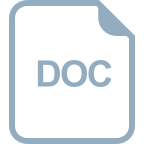
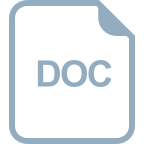
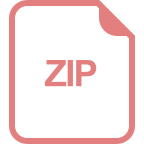
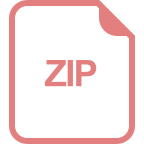
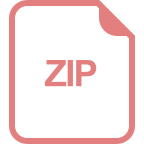
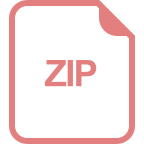
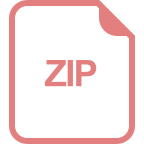
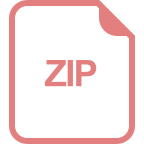
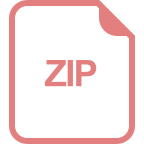
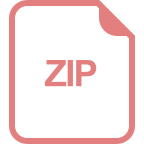
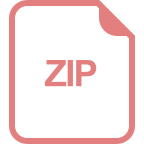
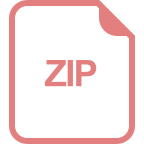
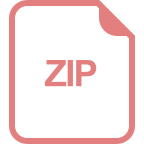
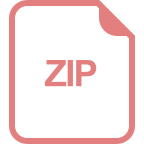
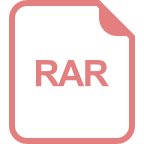
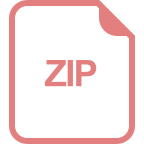