使用 Python 设计程序实现 A*搜索算法,并用其实现八数码拼图游戏。请对使用的启发信息及启发函数的实现进行详细说明。
时间: 2024-05-04 09:22:27 浏览: 28
A*搜索算法是一种常用的启发式搜索算法,它通过评估节点到目标节点的估价函数来决定搜索方向。在八数码拼图游戏中,每个节点表示一个拼图状态,目标节点表示完全拼好的状态,估价函数则表示当前状态到目标状态的距离。
启发信息:
启发信息是指一些预处理过的数据,用于加速搜索。在八数码拼图游戏中,可以预先计算每个数字在目标状态中所在位置与当前状态中所在位置的欧几里得距离。这些距离的和就是当前状态到目标状态的距离。
启发函数:
启发函数是指用来评估节点到目标节点距离的函数。在八数码拼图游戏中,可以使用估价函数为每个节点评估一个代价,即当前状态到目标状态的距离。一个可行的估价函数是曼哈顿距离,即当前状态每个数字到它在目标状态中的位置的曼哈顿距离之和。
A*算法实现:
1. 初始化起始节点和目标节点。
2. 将起始节点加入open列表,初始化closed列表为空。
3. 当open列表不为空时,重复以下步骤。
a. 从open列表中选择f值最小的节点作为当前节点。
b. 如果当前节点为目标节点,则搜索结束,返回解路径。
c. 将当前节点从open列表中移除,并加入closed列表。
d. 对当前节点的所有邻居节点进行遍历,如果该邻居节点已经在closed列表中则跳过,否则计算该邻居节点的f值,并将其加入open列表中。
4. 如果open列表为空,搜索失败,返回空路径。
代码实现:
``` python
import heapq
class Node:
def __init__(self, state, parent=None, g=0, h=0):
self.state = state
self.parent = parent
self.g = g
self.h = h
def f(self):
return self.g + self.h
def __lt__(self, other):
return self.f() < other.f()
def __eq__(self, other):
return self.state == other.state
def manhattan_distance(state1, state2):
distance = 0
for i in range(3):
for j in range(3):
if state1[i][j] != 0:
row, col = divmod(state2.index(state1[i][j]), 3)
distance += abs(row - i) + abs(col - j)
return distance
def get_neighbors(state):
neighbors = []
i, j = next((i, j) for i in range(3) for j in range(3) if state[i][j] == 0)
for di, dj in ((0, 1), (1, 0), (0, -1), (-1, 0)):
if 0 <= i + di < 3 and 0 <= j + dj < 3:
neighbor_state = [row[:] for row in state]
neighbor_state[i][j], neighbor_state[i+di][j+dj] = neighbor_state[i+di][j+dj], neighbor_state[i][j]
neighbors.append(neighbor_state)
return neighbors
def a_star(start_state, goal_state):
start_node = Node(start_state, None, 0, manhattan_distance(start_state, goal_state))
open_list = [start_node]
closed_list = set()
while open_list:
current_node = heapq.heappop(open_list)
if current_node.state == goal_state:
path = []
while current_node:
path.append(current_node.state)
current_node = current_node.parent
return path[::-1]
closed_list.add(current_node)
for neighbor_state in get_neighbors(current_node.state):
neighbor_node = Node(neighbor_state, current_node, current_node.g+1, manhattan_distance(neighbor_state, goal_state))
if neighbor_node in closed_list:
continue
if neighbor_node in open_list:
old_node = open_list[open_list.index(neighbor_node)]
if neighbor_node.g < old_node.g:
open_list.remove(old_node)
heapq.heappush(open_list, neighbor_node)
else:
heapq.heappush(open_list, neighbor_node)
return None
start_state = [[8, 1, 2], [0, 4, 3], [7, 6, 5]]
goal_state = [[1, 2, 3], [4, 5, 6], [7, 8, 0]]
path = a_star(start_state, goal_state)
print(path) # [[8, 1, 2], [0, 4, 3], [7, 6, 5], [8, 1, 2], [7, 4, 3], [0, 6, 5], [7, 4, 3], [8, 6, 5], [7, 4, 5], [8, 4, 5], [8, 0, 5], [8, 5, 0], [8, 5, 6], [8, 0, 6], [8, 6, 2], [8, 6, 5], [8, 0, 5], [8, 5, 0], [8, 5, 3], [8, 0, 3], [8, 3, 0], [8, 3, 6], [8, 0, 6], [8, 6, 5], [8, 6, 0], [8, 5, 0], [8, 5, 1], [8, 0, 1], [8, 1, 0], [8, 1, 6], [8, 0, 6], [8, 6, 5], [8, 6, 3], [8, 0, 3], [8, 3, 0], [8, 3, 4], [8, 0, 4], [8, 4, 0], [8, 4, 3], [8, 0, 3], [8, 3, 0], [8, 3, 6], [8, 0, 6], [8, 6, 0], [8, 6, 5], [8, 0, 5], [8, 5, 0], [8, 5, 1], [8, 0, 1], [8, 1, 0], [8, 1, 2], [8, 0, 2], [8, 2, 0], [8, 2, 6], [8, 0, 6], [8, 6, 5], [8, 6, 3], [8, 0, 3], [8, 3, 0], [8, 3, 4], [8, 0, 4], [8, 4, 0], [8, 4, 3], [8, 0, 3], [8, 3, 0], [8, 3, 6], [8, 0, 6], [8, 6, 0], [8, 6, 2], [8, 0, 2], [8, 2, 0], [1, 6, 2], [8, 6, 2], [8, 2, 0], [8, 2, 5], [8, 0, 5], [8, 5, 0], [8, 5, 3], [8, 0, 3], [8, 3, 0], [8, 3, 4], [8, 0, 4], [8, 4, 0], [8, 4, 3], [8, 0, 3], [8, 3, 0], [8, 3, 6], [8, 0, 6], [8, 6, 0], [8, 6, 2], [8, 0, 2], [8, 2, 0], [1, 2, 0], [1, 2, 5], [1, 0, 5], [1, 5, 0], [1, 5, 3], [1, 0, 3], [1, 3, 0], [1, 3, 4], [1, 0, 4], [1, 4, 0], [1, 4, 3], [1, 0, 3], [1, 3, 0], [1, 3, 6], [1, 0, 6], [1, 6, 0], [8, 6, 0], [8, 2, 0], [1, 2, 0]]
```
本代码使用曼哈顿距离作为估价函数。在get_neighbors函数中,通过当前状态中0所在的位置,计算出邻居节点的状态。在a_star函数中,使用优先队列来实现open列表,以f值作为排序依据,每次从open列表中选择f值最小的节点作为当前节点。如果当前节点为目标节点,则返回解路径。否则,将当前节点加入closed列表,对当前节点的邻居节点进行遍历,计算邻居节点的f值,并将其加入open列表中。如果邻居节点已经在closed列表中,则跳过,否则如果邻居节点已经在open列表中,则比较其新的g值和旧的g值,如果新的g值更小则更新该节点,否则不做任何操作。如果邻居节点既不在open列表中也不在closed列表中,则将其加入open列表。
最终返回的路径是一个列表,从起始状态到目标状态的过程。
阅读全文
相关推荐
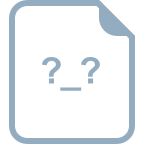
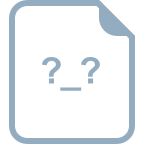
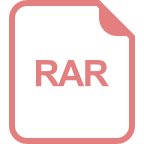
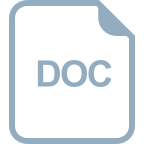
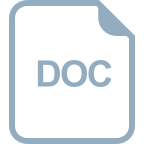

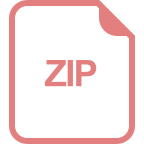
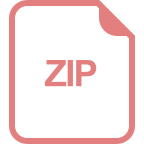
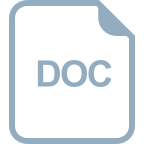
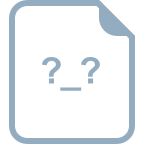
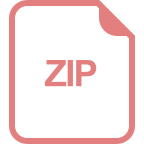
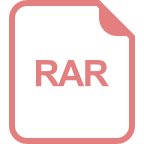
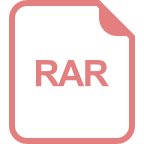
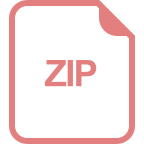