用python探索启发A*算法解决八数码问题
时间: 2023-02-06 21:12:02 浏览: 82
好的,我来为你解释一下如何使用 Python 探索 A* 算法来解决八数码问题。
首先,你需要了解 A* 算法的基本原理。A* 算法是一种启发式搜索算法,它在搜索解决方案的过程中使用了一个启发函数来估算当前状态与目标状态的距离。这样可以帮助 A* 算法在搜索解决方案时更快地找到最优解。
八数码问题是一个经典的状态空间搜索问题,它的目标是通过改变每个数字的位置来将初始状态转换为目标状态。为了使用 A* 算法来解决八数码问题,你需要实现以下步骤:
1. 定义状态类型:首先,你需要定义一个状态类型来表示八数码问题的每个状态。你可以使用一个数组来表示这个状态,其中每个元素都是一个数字,表示该数字所在的位置。
2. 实现启发函数:你需要实现一个启发函数,用来估算当前状态与目标状态的距离。你可以使用曼哈顿距离或折返距离作为启发函数。
3. 实现搜索算法:
相关问题
用python语言使用A*算法求解八数码问题
八数码问题是一种经典的搜索问题,要求把一个3x3的棋盘上的数字1-8排列成特定的顺序。本文将使用A*算法来求解八数码问题。
A*算法是一种启发式搜索算法,它使用估价函数来评估每个节点的价值,并选择最有希望的节点进行扩展。估价函数是一个启发式函数,它可以预测从当前节点到目标节点的最小代价。在八数码问题中,我们可以使用曼哈顿距离作为估价函数。
以下是使用Python语言实现A*算法求解八数码问题的代码:
```python
import heapq
# 计算曼哈顿距离
def manhattan_distance(state):
distance = 0
for i in range(3):
for j in range(3):
if state[i][j] != 0:
row = (state[i][j] - 1) // 3
col = (state[i][j] - 1) % 3
distance += abs(row - i) + abs(col - j)
return distance
# 判断状态是否合法
def is_valid(state):
nums = set()
for row in state:
for num in row:
if num != 0:
if num in nums:
return False
nums.add(num)
return True
# 获取空格位置
def get_blank(state):
for i in range(3):
for j in range(3):
if state[i][j] == 0:
return (i, j)
# 获取下一步可能的状态
def get_next_states(state):
blank = get_blank(state)
row, col = blank
next_states = []
for i, j in [(row-1, col), (row+1, col), (row, col-1), (row, col+1)]:
if i >= 0 and i < 3 and j >= 0 and j < 3:
next_state = [row[:] for row in state]
next_state[row][col], next_state[i][j] = next_state[i][j], next_state[row][col]
next_states.append(next_state)
return next_states
# A*算法求解八数码问题
def solve_puzzle(start_state, goal_state):
if not is_valid(start_state) or not is_valid(goal_state):
return None
# 初始化起始状态
start_node = {'state': start_state, 'g': 0, 'h': manhattan_distance(start_state), 'parent': None}
open_list = [start_node]
closed_list = set()
while len(open_list) > 0:
# 选择最小估价函数值的节点进行扩展
current_node = heapq.heappop(open_list)
# 判断是否到达目标状态
if current_node['state'] == goal_state:
path = []
while current_node is not None:
path.append(current_node['state'])
current_node = current_node['parent']
path.reverse()
return path
# 将当前节点加入关闭列表
closed_list.add(str(current_node['state']))
# 扩展下一步可能的状态
for next_state in get_next_states(current_node['state']):
if str(next_state) not in closed_list:
# 计算估价函数值,并加入开放列表
next_node = {'state': next_state, 'g': current_node['g']+1, 'h': manhattan_distance(next_state), 'parent': current_node}
heapq.heappush(open_list, next_node)
# 无解
return None
# 测试
start_state = [[2, 8, 3], [1, 6, 4], [7, 0, 5]]
goal_state = [[1, 2, 3], [8, 0, 4], [7, 6, 5]]
path = solve_puzzle(start_state, goal_state)
if path is not None:
for state in path:
print(state)
else:
print("无解")
```
以上代码中,`manhattan_distance`函数计算曼哈顿距离;`is_valid`函数判断状态是否合法;`get_blank`函数获取空格位置;`get_next_states`函数获取下一步可能的状态;`solve_puzzle`函数使用A*算法求解八数码问题。在测试中,我们设置初始状态为`[[2, 8, 3], [1, 6, 4], [7, 0, 5]]`,目标状态为`[[1, 2, 3], [8, 0, 4], [7, 6, 5]]`,并输出求解路径。
a*算法解决八数码问题python
### 回答1:
a*算法是一种启发式搜索算法,可以用于解决八数码问题。八数码问题是一种经典的游戏,目标是将一个3*3的九宫格中的数字按照特定顺序排列,其中一个格子为空。每次操作可以将与空格相邻的一个数字移动到空格中,直到达到目标状态。
使用a*算法解决八数码问题主要有以下几个步骤:
1. 定义状态空间:将九宫格中的数字排列看作状态,即每个状态由一个长度为9的数组来表示。
2. 定义启发函数:启发函数用来评估当前状态距离目标状态的距离,通常使用曼哈顿距离或欧几里得距离。在八数码问题中,使用曼哈顿距离计算两个状态之间的距离。
3. 定义操作:定义将一个数字移动到空格中的操作,以及对应的代价。在八数码问题中,每次操作的代价都为1。
4. 使用优先队列存储状态:使用优先队列存储每个状态以及与起始状态的距离 + 启发函数值。
5. 开始搜索:从初始状态开始,每次取出距离 + 启发函数值最小的状态,并对其进行操作,得到一系列可能的下一个状态。将这些状态加入优先队列,并继续搜索,直到找到目标状态。
6. 输出解:当找到目标状态后,可以通过反向遍历得到从目标状态到初始状态的一条路径,即为解。将路径输出即可。
使用Python实现a*算法解决八数码问题具体实现可以参考相关教程或代码库。
### 回答2:
在八数码问题中,有一个3x3的矩阵,其中包含1-8号数字,以及一个空位。基本目标是将矩阵重排、使得排列成指定的形式。
a*算法,是一种基于启发式搜索的算法,它可以在有较大状态空间的问题中找到最优解。在求解八数码问题时,a*算法可以被用来搜索空位所处位置的不同状态,并采用估价函数来判断哪些状态更有可能走向正确的解决方案。
基于估价函数,a*算法被用来搜索状态时已经做好了最小化搜索路径长度的准备,也就是说,它可以尽可能快地找到最优解。
实现a*算法解决八数码问题的Python代码,可以分多层解决。首先,需要定义一个函数,用于获取空格的位置。通过该函数,可以确定出当前状况空格往四个方向所能到达的状态。
下一步,需要判断每一个移动后的状态是否合法。移动状态需要计算出一个估价函数的值,来评估该状态是否最有可能走向目标正确状态。
用Python实现时,估价函数可以定义为当前状态离目标状态越远,则评估函数值越大。估价函数的实现可以使用曼哈顿距离来计算两个状态之间的距离。
接下来,通过a*算法进行搜索,直到找到最优解。在算法中,首先通过一个优先级队列(priority queue)来对状态进行排序和筛选。在每一个移动后的状态中,选择估价函数值最小的状态进行搜索。最终,可以找到最优的解决方案。
### 回答3:
A*算法是一种用于路径规划的算法,它能够有效地解决八数码问题。八数码问题是指在 3×3 的九宫格中,一个初始状态可以移到目标状态的谜题。在八数码问题中,每个格子可以放置数字1-8或空格,规则是只能上下左右移动,将空格移到目标状态,同时保证空格移动路径最短。
在Python中,构建A*算法解决八数码问题的步骤如下:
1.构建初始的状态和目标状态
定义一个 3 * 3 的列表,用0表示空格,用1-8表示数字。例如,一个样例状态为:[1,2,3,4,5,6,0,7,8]。
2.计算需要移动的步数
通过计算当前状态和目标状态之间不同的数字的个数,即曼哈顿距离(Manhattan distance),来计算出当前状态的评估函数(f(n))。
3.确定移动的方向
向当前空格的周围四个方向依次移动,计算移动后的状态的评估函数f(n)。
4.加入已探索列表
将移动后的状态加入已探索的状态列表中。
5.重复步骤2-4,直到找到目标状态。
如果当前状态和目标状态一致,则搜索结束。否则,重复步骤2-4直到找到目标状态。此时,需要返回最短路径。
6.输出最终答案
输出从初始状态到目标状态的路径。
总体来说,A*算法是一种有效的搜索算法,在处理八数码问题中有着不错的应用效果。在实现A*算法时,要注意选择正确的数据结构和算法实现方法,并严格控制代码的时间复杂度,以提高算法的效率。
相关推荐
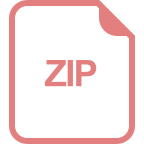












