a*算法八数码启发式函数设计代码
时间: 2023-07-16 21:13:56 浏览: 46
A* 算法是一种启发式搜索算法,可以用于解决八数码等问题。其中启发式函数是 A* 算法的核心,可以通过不同的启发式函数来提高搜索效率。以下是一个八数码问题的 A* 算法代码,包括启发式函数的设计。
```python
# 八数码问题的启发式函数设计代码
import copy
import heapq
# 定义八数码的目标状态
goal_state = [[1, 2, 3], [4, 5, 6], [7, 8, 0]]
# 计算当前状态与目标状态之间的曼哈顿距离
def manhattan_distance(state):
distance = 0
for i in range(3):
for j in range(3):
if state[i][j] != 0:
x, y = divmod(state[i][j]-1, 3)
distance += abs(x-i) + abs(y-j)
return distance
# 定义节点类
class Node:
def __init__(self, state, parent, g, h):
self.state = state
self.parent = parent
self.g = g
self.h = h
# 定义节点的比较函数
def __lt__(self, other):
return self.g + self.h < other.g + other.h
# 定义 A* 算法函数
def a_star(start_state):
open_list = []
closed_list = set()
heapq.heappush(open_list, Node(start_state, None, 0, manhattan_distance(start_state)))
while open_list:
node = heapq.heappop(open_list)
if node.state == goal_state:
path = []
while node:
path.append(node.state)
node = node.parent
return path[::-1]
closed_list.add(tuple(map(tuple, node.state)))
for i, j in [(0, 1), (1, 0), (0, -1), (-1, 0)]:
x, y = divmod(node.state.index(0), 3)
if 0 <= x+i < 3 and 0 <= y+j < 3:
new_state = copy.deepcopy(node.state)
new_state[x][y], new_state[x+i][y+j] = new_state[x+i][y+j], new_state[x][y]
if tuple(map(tuple, new_state)) not in closed_list:
heapq.heappush(open_list, Node(new_state, node, node.g+1, manhattan_distance(new_state)))
# 测试
start_state = [[2, 8, 3], [1, 6, 4], [7, 0, 5]]
path = a_star(start_state)
for state in path:
for row in state:
print(row)
print()
```
在上述代码中,我们首先定义了八数码的目标状态 `goal_state`,然后实现了启发式函数 `manhattan_distance`,用于计算当前状态与目标状态之间的曼哈顿距离。接着定义了节点类 `Node`,其中包含了当前状态、父节点、从起始节点到当前节点的代价 `g`、以及当前状态的启发式函数值 `h`。在 `Node` 类中,我们还实现了比较函数 `__lt__`,用于在优先队列中比较节点的优先级。最后,我们实现了 A* 算法函数 `a_star`,其中使用了优先队列来存储待扩展的节点,并使用 `set()` 来存储已经扩展过的节点。在每次从队列中取出节点时,我们首先判断当前状态是否为目标状态,如果是,则返回从起始节点到当前节点的路径;否则,我们将当前状态加入 `closed_list` 中,然后按照上下左右四个方向拓展节点,并将新的节点加入 `open_list` 中。在拓展节点时,我们需要注意判断当前状态是否已经被扩展过,如果已经扩展过,则直接跳过。
在实际应用中,我们可以根据具体问题设计不同的启发式函数,以提高搜索效率。
相关推荐
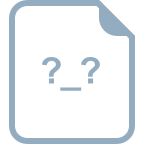
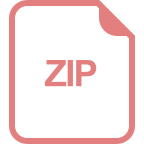
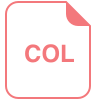
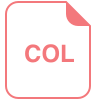
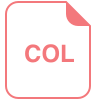
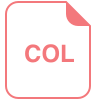
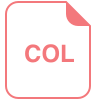









