A*算法解决15数码问题_Python实现
时间: 2023-07-20 07:36:16 浏览: 66
好的,以下是 A*算法解决15数码问题的 Python 实现:
```python
import heapq
# 定义状态类
class State:
def __init__(self, state, g, h, parent):
self.state = state
self.g = g # 已经走过的步数
self.h = h # 启发函数估计的剩余步数
self.parent = parent # 父节点
# 定义比较方法
def __lt__(self, other):
return self.g + self.h < other.g + other.h
# 定义哈希方法
def __hash__(self):
return hash(str(self.state))
# 定义相等方法
def __eq__(self, other):
return str(self.state) == str(other.state)
# 定义启发函数,这里使用曼哈顿距离
def heuristic(state):
h = 0
for i in range(4):
for j in range(4):
if state[i][j] != 0:
x, y = divmod(state[i][j] - 1, 4)
h += abs(x - i) + abs(y - j)
return h
# 定义 A*算法
def A_star(start_state):
# 定义初始状态
start = State(start_state, 0, heuristic(start_state), None)
# 定义已经遍历过的状态
explored = set()
# 定义优先队列
pq = []
heapq.heappush(pq, start)
while pq:
# 取出 f 值最小的状态
curr = heapq.heappop(pq)
# 如果当前状态是目标状态,返回路径
if curr.h == 0:
path = []
while curr:
path.append(curr.state)
curr = curr.parent
return path[::-1]
# 将当前状态加入已遍历过的状态
explored.add(curr)
# 扩展当前状态
for move in [(0, 1), (1, 0), (0, -1), (-1, 0)]:
new_state = curr.state.copy()
i, j = [(i, j) for i in range(4) for j in range(4) if new_state[i][j] == 0][0]
ni, nj = i + move[0], j + move[1]
if 0 <= ni < 4 and 0 <= nj < 4:
new_state[i][j], new_state[ni][nj] = new_state[ni][nj], new_state[i][j]
# 生成新状态并计算 g 和 h
new_node = State(new_state, curr.g + 1, heuristic(new_state), curr)
# 如果新状态没有被遍历过,加入优先队列
if new_node not in explored:
heapq.heappush(pq, new_node)
# 如果无解,返回空列表
return []
```
你可以使用这个函数来解决 15 数码问题。
相关推荐
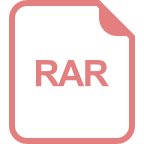
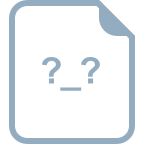














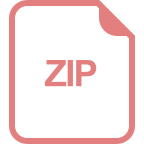