python实现36数码问题(A*算法)
时间: 2023-12-04 20:56:00 浏览: 32
36数码问题是一种经典的搜索问题,其中一个3x3的九宫格中有1~9的数字和一个空格,目标是将数字移动到正确的位置,最终形成如下状态:
1 2 3
4 5 6
7 8
在此过程中,只能将数字移动到相邻的空格中,例如,如果空格在位置(1,1),那么只能将数字2或者4移动到该位置,如果空格在位置(2,2),那么可以将数字2、5或者8移动到该位置。
A*算法是一种启发式搜索算法,可以用于解决36数码问题。下面是使用Python实现36数码问题的A*算法的代码:
```
from queue import PriorityQueue
class Puzzle:
def __init__(self, init_state, goal_state):
self.init_state = init_state
self.goal_state = goal_state
def find_blank(self, state):
for i in range(3):
for j in range(3):
if state[i][j] == 0:
return i, j
def move_blank(self, state, move):
blank_row, blank_col = self.find_blank(state)
new_row = blank_row + move[0]
new_col = blank_col + move[1]
if new_row >= 0 and new_col >= 0 and new_row < 3 and new_col < 3:
new_state = [row[:] for row in state]
new_state[blank_row][blank_col], new_state[new_row][new_col] = new_state[new_row][new_col], new_state[blank_row][blank_col]
return new_state
return None
def get_moves(self, state):
moves = []
moves.append((-1, 0))
moves.append((1, 0))
moves.append((0, -1))
moves.append((0, 1))
valid_moves = []
for move in moves:
new_state = self.move_blank(state, move)
if new_state:
valid_moves.append((new_state, move))
return valid_moves
def get_heuristic(self, state):
distance = 0
for i in range(3):
for j in range(3):
if state[i][j] != 0:
row = (state[i][j] - 1) // 3
col = (state[i][j] - 1) % 3
distance += abs(row - i) + abs(col - j)
return distance
def solve(self):
queue = PriorityQueue()
queue.put((self.get_heuristic(self.init_state), self.init_state, [], 0))
visited = set()
while not queue.empty():
_, state, path, cost = queue.get()
if state == self.goal_state:
return path
if tuple(map(tuple, state)) not in visited:
visited.add(tuple(map(tuple, state)))
for next_state, move in self.get_moves(state):
next_cost = cost + 1
next_path = path + [move]
priority = next_cost + self.get_heuristic(next_state)
queue.put((priority, next_state, next_path, next_cost))
return None
if __name__ == '__main__':
init_state = [[2, 3, 8], [1, 0, 4], [7, 6, 5]]
goal_state = [[1, 2, 3], [4, 5, 6], [7, 8, 0]]
puzzle = Puzzle(init_state, goal_state)
path = puzzle.solve()
if path:
for move in path:
print(move)
else:
print('No solution')
```
在上面的代码中,Puzzle类表示36数码问题的状态和操作,包括查找空格、移动空格、获取有效移动、获取启发式函数等。solve()方法使用A*算法搜索最优解,并返回最佳路径。
在main()函数中,我们可以指定初始状态和目标状态,然后调用solve()方法求解最优路径并输出。如果没有解,则输出"No solution"。
相关推荐
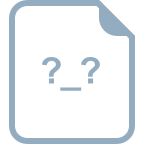










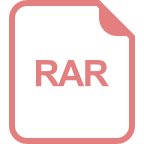
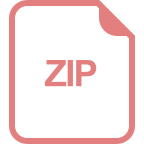