element-ui table实现增删改查
时间: 2023-09-07 10:17:46 浏览: 139
Element UI Table 提供了一些 API 和事件,可以方便地实现增删改查操作。下面是一个简单的示例:
```vue
<template>
<div>
<el-table :data="tableData" style="width:100%">
<el-table-column prop="name" label="姓名"></el-table-column>
<el-table-column prop="age" label="年龄"></el-table-column>
<el-table-column label="操作">
<template slot-scope="scope">
<el-button @click="handleEdit(scope.row)">编辑</el-button>
<el-button @click="handleDelete(scope.$index)">删除</el-button>
</template>
</el-table-column>
</el-table>
<el-dialog :visible.sync="dialogVisible">
<el-form :model="currentRow">
<el-form-item label="姓名">
<el-input v-model="currentRow.name"></el-input>
</el-form-item>
<el-form-item label="年龄">
<el-input v-model.number="currentRow.age"></el-input>
</el-form-item>
</el-form>
<div slot="footer">
<el-button @click="dialogVisible = false">取消</el-button>
<el-button type="primary" @click="handleSave">保存</el-button>
</div>
</el-dialog>
<el-button type="primary" @click="handleAdd">新增</el-button>
</div>
</template>
<script>
export default {
data() {
return {
tableData: [
{ name: '张三', age: 18 },
{ name: '李四', age: 20 },
{ name: '王五', age: 22 }
],
dialogVisible: false,
currentRow: {}
}
},
methods: {
handleAdd() {
this.currentRow = {}
this.dialogVisible = true
},
handleEdit(row) {
this.currentRow = Object.assign({}, row)
this.dialogVisible = true
},
handleDelete(index) {
this.tableData.splice(index, 1)
},
handleSave() {
if (this.currentRow.name && this.currentRow.age) {
const index = this.tableData.indexOf(this.currentRow)
if (index > -1) {
Object.assign(this.tableData[index], this.currentRow)
} else {
this.tableData.push(this.currentRow)
}
this.dialogVisible = false
}
}
}
}
</script>
```
上面的代码中,我们定义了一个 `tableData` 数组,用于存储表格数据。通过 `<el-table>` 组件和 `<el-table-column>` 组件,我们可以将数据呈现为表格形式,并显示操作按钮。
当用户点击“新增”按钮时,我们将 `currentRow` 清空,并打开弹窗。当用户点击“编辑”按钮时,我们将 `currentRow` 设置为当前行数据,并打开弹窗。当用户点击“删除”按钮时,我们从 `tableData` 中删除对应的行数据。
弹窗中包含一个表单,用于编辑行数据。当用户点击“保存”按钮时,我们根据 `currentRow` 中的数据,更新 `tableData` 中对应的行数据,或者将 `currentRow` 添加到 `tableData` 中。如果 `currentRow` 的数据不完整,我们不执行任何操作,并保持弹窗打开状态。
通过这种方式,我们可以方便地实现基本的增删改查功能。当然,如果需要更复杂的操作,可以根据具体需求进行扩展。
阅读全文
相关推荐
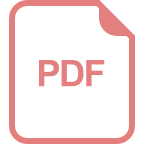
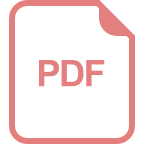
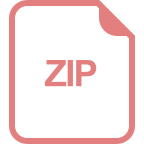





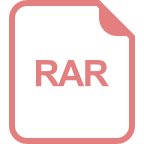
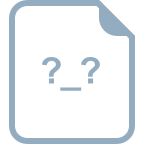
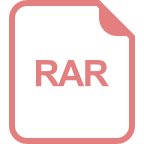
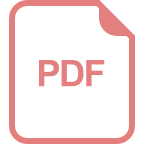
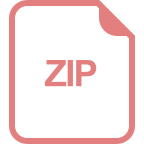
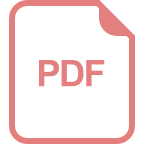
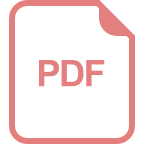
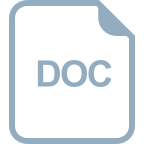
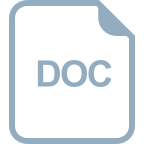
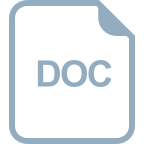
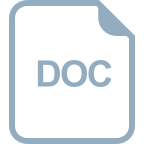