如何在Java编程中使用HBase创建名为'dept'和'emp'的表,并为它们各自定义数据列'related_data'?请提供相应的Java代码示例以展示这个过程。
时间: 2024-12-14 08:25:20 浏览: 12
在Java中,你可以使用Apache HBase的`HBaseAdmin`类来创建表和定义列族。以下是一个简单的步骤说明以及对应的Java代码示例:
首先,确保你已经添加了HBase的依赖到你的项目中。如果你使用的是Maven,可以在pom.xml文件中添加以下依赖:
```xml
<dependency>
<groupId>org.apache.hbase</groupId>
<artifactId>hbase-client</artifactId>
<version>YOUR_HBASE_VERSION</version>
</dependency>
```
然后,你需要创建一个`HBaseAdmin`实例并连接到HBase集群:
```java
import org.apache.hadoop.conf.Configuration;
import org.apache.hadoop.hbase.HBaseConfiguration;
import org.apache.hadoop.hbase.client.Admin;
public class HBaseTableCreation {
private static final String ZOOKEEPER_QUORUM = "your_zookeeper_quorum";
private static final int ZOOKEEPER_PORT = your_zookeeper_port;
private static final String REGION_SERVERS = "your_region_servers";
public static void main(String[] args) throws Exception {
Configuration conf = HBaseConfiguration.create();
// 设置Zookeeper地址、端口和Region服务器列表
conf.set("zookeeper.quorum", ZOOKEEPER_QUORUM);
conf.set("zookeeper.znode.parent", "/hbase");
conf.set("hbase.zookeeper.property.dataDir", "/path/to/zookeeper/data");
Admin admin = new Admin(conf);
try {
// 创建表
createDepartmentTable(admin);
createEmployeeTable(admin);
} finally {
admin.close(); // 关闭Admin连接
}
}
private static void createDepartmentTable(Admin admin) throws Exception {
if (!admin.tableExists("dept")) {
admin.createTable(new TableDescriptorBuilder()
.setTableName("dept")
.addFamily(new ColumnFamilyDescriptorBuilder().setColumnFamilyName("related_data").build())
.build());
System.out.println("Department table created successfully.");
} else {
System.out.println("Department table already exists.");
}
}
private static void createEmployeeTable(Admin admin) throws Exception {
if (!admin.tableExists("emp")) {
admin.createTable(new TableDescriptorBuilder()
.setTableName("emp")
.addFamily(new ColumnFamilyDescriptorBuilder().setColumnFamilyName("related_data").build())
.build());
System.out.println("Employee table created successfully.");
} else {
System.out.println("Employee table already exists.");
}
}
}
```
在这个例子中,我们创建了两个表,"dept"和"emp",都包含一个列族"related_data"。替换`your_zookeeper_quorum`、`your_zookeeper_port`和`your_region_servers`为你实际的ZooKeeper配置。
阅读全文
相关推荐
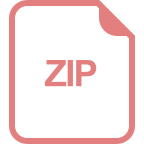
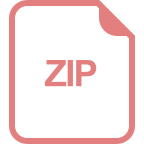
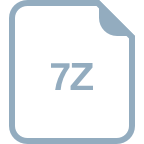



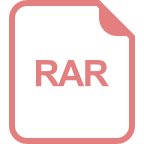
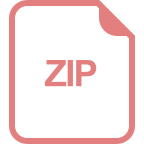
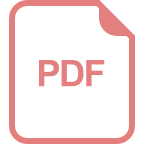
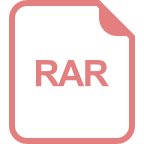
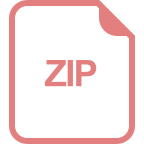
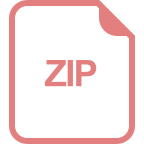
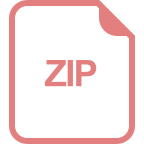
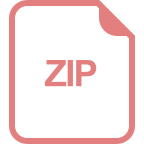
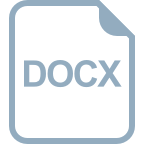
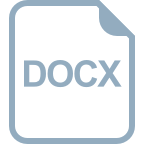