FOC高频注入启动C代码
时间: 2024-01-14 20:14:20 浏览: 192
以下是一个简单的FOC高频注入启动C代码示例:
```c
#include <stdio.h>
#include <math.h>
#define PI 3.14159265358979323846
// 定义电机参数
double Rs = 1.0;
double Ls = 0.001;
double Lr = 0.001;
double J = 0.1;
double P = 4;
// 定义FOC参数
double Ts = 0.0001;
double T = 0.01;
double f = 1000.0;
double omega = 2.0 * PI * f;
// 定义高频注入参数
double amplitude = 0.1;
double freq = 20000.0;
// 定义电机状态
double Id = 0.0;
double Iq = 0.0;
double omega_e = 0.0;
double theta_e = 0.0;
double theta_m = 0.0;
double torque = 0.0;
double speed = 0.0;
// 定义高频注入状态
double hf_amplitude = 0.0;
double hf_frequency = 0.0;
// 计算电机转矩
double calculate_torque(double Id, double Iq, double theta_e, double omega_e) {
double flux_d = Ls * Id + Lr * sin(theta_e);
double flux_q = Ls * Iq + Lr * cos(theta_e);
double torque = P * (flux_d * Iq - flux_q * Id) / (2 * PI * f);
return torque;
}
// 计算电机速度
double calculate_speed(double omega_e, double theta_e, double theta_m) {
double speed = omega_e * P / (2 * PI) + theta_m / T;
return speed;
}
// 计算高频注入信号
void calculate_hf_signal(double time) {
hf_amplitude = amplitude * sin(2.0 * PI * freq * time);
hf_frequency = freq;
}
// FOC控制
void foc_control(double Va, double Vb, double Vc, double time) {
// 计算高频注入信号
calculate_hf_signal(time);
// 计算电机电流
double Vd = Va * cos(theta_e) + Vb * cos(theta_e - 2.0 * PI / 3.0) + Vc * cos(theta_e + 2.0 * PI / 3.0);
double Vq = -Va * sin(theta_e) - Vb * sin(theta_e - 2.0 * PI / 3.0) - Vc * sin(theta_e + 2.0 * PI / 3.0);
double Iq_new = Iq + (Ts / Ls) * (Vq - Rs * Iq - omega_e * Id + hf_amplitude * sin(2.0 * PI * hf_frequency * time));
double Id_new = Id + (Ts / Ls) * (Vd - Rs * Id + omega_e * Iq - torque / flux_d + hf_amplitude * sin(2.0 * PI * hf_frequency * time));
// 更新电机状态
Id = Id_new;
Iq = Iq_new;
theta_e = theta_e + Ts * omega_e;
torque = calculate_torque(Id, Iq, theta_e, omega_e);
speed = calculate_speed(omega_e, theta_e, theta_m);
// 更新转子电机角度
theta_m = theta_m + Ts * speed;
omega_e = omega * P / 2.0 + speed;
}
int main() {
// 初始化电机状态
theta_e = 0.0;
theta_m = 0.0;
omega_e = omega * P / 2.0;
// FOC控制循环
for (double time = 0.0; time < T; time += Ts) {
// 生成三相电压
double Va = 0.2;
double Vb = 0.2;
double Vc = 0.2;
// FOC控制
foc_control(Va, Vb, Vc, time);
// 输出电机状态
printf("Time: %.5f, Id: %.5f, Iq: %.5f, Theta_m: %.5f, Speed: %.5f\n", time, Id, Iq, theta_m, speed);
}
return 0;
}
```
这是一个基本的FOC高频注入启动C代码示例,其中包含了电机参数、FOC参数和高频注入参数的定义,以及电机状态的计算和更新。在FOC控制循环中,我们生成了三相电压,并通过FOC控制计算电机电流和状态。最后,我们输出了电机状态,包括电流、转子角度、速度等。
阅读全文
相关推荐
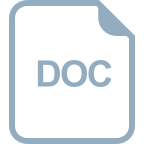
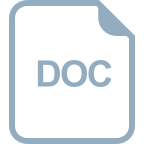
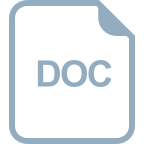



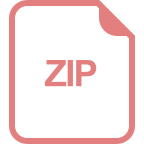


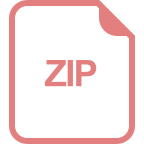
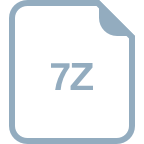
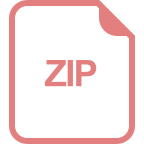
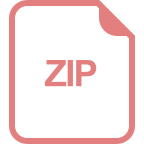
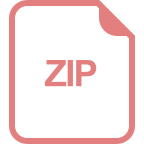



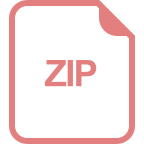
