pygame点击图片后换成另一张图
时间: 2023-09-12 18:05:15 浏览: 133
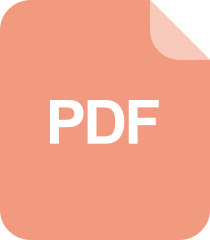
鼠标移动到一张图片时变为另一张图片
你可以使用 Pygame 中的鼠标事件和图像绘制功能来实现点击图片后换成另一张图的效果,具体步骤如下:
1. 加载需要显示的两张图片,例如:
```
img1 = pygame.image.load('image1.png')
img2 = pygame.image.load('image2.png')
```
2. 定义一个变量来记录当前显示的图片,初始值为第一张图片:
```
current_img = img1
```
3. 在游戏主循环中监听鼠标事件,例如:
```
for event in pygame.event.get():
if event.type == pygame.MOUSEBUTTONDOWN:
# 判断鼠标是否点击了图片区域
if img_rect.collidepoint(event.pos):
# 切换图片
if current_img == img1:
current_img = img2
else:
current_img = img1
```
其中,`img_rect` 是图片的矩形区域,可以使用 `get_rect()` 方法获取,例如:
```
img_rect = img1.get_rect()
```
4. 在游戏主循环中绘制当前显示的图片,例如:
```
screen.blit(current_img, img_rect)
```
完整的示例代码如下:
```
import pygame
pygame.init()
# 加载图片
img1 = pygame.image.load('image1.png')
img2 = pygame.image.load('image2.png')
# 设置窗口尺寸和标题
size = (800, 600)
screen = pygame.display.set_mode(size)
pygame.display.set_caption('Click to Change Image')
# 获取图片矩形区域
img_rect = img1.get_rect()
# 初始显示第一张图片
current_img = img1
# 游戏主循环
while True:
for event in pygame.event.get():
if event.type == pygame.QUIT:
pygame.quit()
quit()
elif event.type == pygame.MOUSEBUTTONDOWN:
if img_rect.collidepoint(event.pos):
# 切换图片
if current_img == img1:
current_img = img2
else:
current_img = img1
# 绘制当前显示的图片
screen.blit(current_img, img_rect)
# 更新屏幕显示
pygame.display.update()
```
在示例代码中,我假设了图片文件名为 `image1.png` 和 `image2.png`,你需要将其替换为实际的图片文件名。
阅读全文
相关推荐
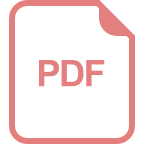















