这个工具类不错,能把get请求也带body吗
时间: 2024-03-03 19:51:27 浏览: 17
GET请求本身是不支持带有请求体(RequestBody)的,因为GET请求的参数是通过URL传递的。但是,有些情况下,我们可能需要在GET请求中传递一些复杂的参数,比如JSON格式的参数,这时候可以把参数拼接到URL中,然后以普通的形式发送GET请求。下面是一个示例代码:
```java
import org.apache.http.HttpEntity;
import org.apache.http.client.methods.CloseableHttpResponse;
import org.apache.http.client.methods.HttpGet;
import org.apache.http.client.methods.HttpPost;
import org.apache.http.entity.StringEntity;
import org.apache.http.impl.client.CloseableHttpClient;
import org.apache.http.impl.client.HttpClients;
import org.apache.http.util.EntityUtils;
import java.io.IOException;
import java.net.URLEncoder;
public class HttpUtil {
/**
* 发送GET请求
*
* @param url 请求地址
* @return 响应结果
* @throws IOException
*/
public static String doGet(String url) throws IOException {
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpGet httpGet = new HttpGet(url);
CloseableHttpResponse response = null;
try {
response = httpClient.execute(httpGet);
HttpEntity entity = response.getEntity();
if (entity != null) {
return EntityUtils.toString(entity);
}
} finally {
if (response != null) {
response.close();
}
httpClient.close();
}
return null;
}
/**
* 发送带有请求体的GET请求
*
* @param url 请求地址
* @param data 请求参数,JSON格式
* @return 响应结果
* @throws IOException
*/
public static String doGetWithBody(String url, String data) throws IOException {
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpGet httpGet = new HttpGet(url + "?" + URLEncoder.encode(data, "utf-8"));
CloseableHttpResponse response = null;
try {
response = httpClient.execute(httpGet);
HttpEntity entity = response.getEntity();
if (entity != null) {
return EntityUtils.toString(entity);
}
} finally {
if (response != null) {
response.close();
}
httpClient.close();
}
return null;
}
/**
* 发送POST请求
*
* @param url 请求地址
* @param data 请求参数,JSON格式
* @return 响应结果
* @throws IOException
*/
public static String doPost(String url, String data) throws IOException {
CloseableHttpClient httpClient = HttpClients.createDefault();
HttpPost httpPost = new HttpPost(url);
CloseableHttpResponse response = null;
try {
StringEntity entity = new StringEntity(data, "utf-8");
httpPost.setEntity(entity);
httpPost.setHeader("Content-Type", "application/json");
response = httpClient.execute(httpPost);
HttpEntity responseEntity = response.getEntity();
if (responseEntity != null) {
return EntityUtils.toString(responseEntity);
}
} finally {
if (response != null) {
response.close();
}
httpClient.close();
}
return null;
}
}
```
我们新增了一个名为doGetWithBody的方法,用于发送带有请求体的GET请求。在这个方法中,我们将请求体拼接到URL中,然后将URL作为参数传递给HttpGet实例。在使用这个方法时,我们只需要将请求体以JSON格式传递给这个方法即可。
需要注意的是,在将请求体拼接到URL中时,需要对请求体进行URL编码,否则可能会导致请求失败或响应结果不正确。
相关推荐
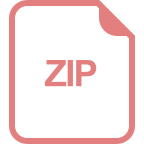
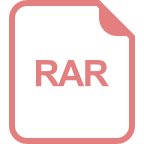
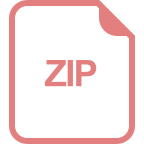














