通过库中的函数写BA无标度网络
时间: 2023-12-03 18:46:27 浏览: 84
BA无标度网络可以通过以下步骤生成:
1. 导入必要的库,如 `networkx`。
```python
import networkx as nx
import matplotlib.pyplot as plt
```
2. 初始化一个空的图。
```python
G = nx.Graph()
```
3. 添加初始节点和边。为了让网络开始具有一些结构,我们需要在开始时添加一些节点和连边。添加几个节点并使它们相互连接。
```python
G.add_edge(0, 1)
G.add_edge(0, 2)
G.add_edge(0, 3)
```
4. 为每个新节点添加连边。在BA模型中,每个新节点都会连接到一些现有节点。这些现有节点被选择的概率与它们的度数成正比。因此,我们需要编写一个函数来选择这些节点。
```python
def add_node_with_edges(G):
new_node = len(G)
nodes = list(G.nodes())
degrees = [G.degree(node) for node in nodes]
probabilities = [degree / sum(degrees) for degree in degrees]
chosen_node = np.random.choice(nodes, p=probabilities)
G.add_edge(new_node, chosen_node)
```
这个函数首先找到所有节点的度数。然后,它计算每个节点被选中的概率。最后,它从这些节点中随机选择一个并添加新节点和连边。
5. 循环添加节点并连接边。我们可以通过多次调用 `add_node_with_edges` 函数来生成一个BA无标度网络。
```python
for i in range(10):
add_node_with_edges(G)
```
6. 可视化网络。最后,我们可以使用 `matplotlib` 库将这个网络可视化。
```python
nx.draw(G, with_labels=True)
plt.show()
```
完整代码如下:
```python
import networkx as nx
import matplotlib.pyplot as plt
import numpy as np
G = nx.Graph()
G.add_edge(0, 1)
G.add_edge(0, 2)
G.add_edge(0, 3)
def add_node_with_edges(G):
new_node = len(G)
nodes = list(G.nodes())
degrees = [G.degree(node) for node in nodes]
probabilities = [degree / sum(degrees) for degree in degrees]
chosen_node = np.random.choice(nodes, p=probabilities)
G.add_edge(new_node, chosen_node)
for i in range(10):
add_node_with_edges(G)
nx.draw(G, with_labels=True)
plt.show()
```
阅读全文
相关推荐
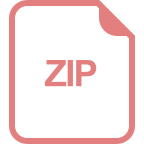
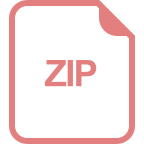
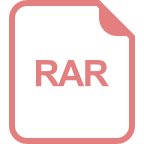
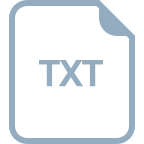
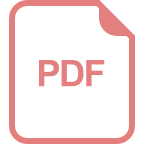
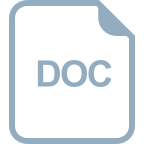
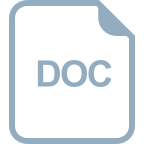
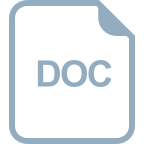







