MAC地址获取组件
时间: 2023-11-12 07:05:34 浏览: 12
在大多数编程语言中,可以使用以下代码获取本机MAC地址:
```python
import uuid
mac = uuid.getnode()
mac_address = ':'.join(['{:02x}'.format((mac >> i) & 0xff) for i in range(0, 48, 8)])
print(mac_address)
```
其中,`mac_address`即为本机MAC地址。需要注意的是,有些操作系统可能需要管理员权限才能获取MAC地址。
相关问题
用Java实现一下内容:1.实现一个图形用户界面的MAC地址获取系统。 2.功能: 2.1接收用户输入的IP地址,判定IP地址的合法性,判定是否与本地主机在同一子网内。 2.2若给定IP合法,并与本地主机地址在同一子网内,则利用ARP包的收发,获取该IP地址对应主机的MAC地址。 2.3将获取的MAC地址显示在窗口的相应组件中。 3.用户界面:界面上包括IP地址输入组件、结果显示组件及操作按钮或菜单等。
这是一个基于Java的图形用户界面的MAC地址获取系统的简单实现。你可以使用Java的Swing库来创建图形界面,以下是一个示例代码:
```java
import javax.swing.*;
import java.awt.*;
import java.awt.event.ActionEvent;
import java.awt.event.ActionListener;
import java.net.InetAddress;
import java.net.NetworkInterface;
import java.net.SocketException;
import java.net.UnknownHostException;
public class MacAddressSystem extends JFrame {
private JTextField ipTextField;
private JLabel resultLabel;
public MacAddressSystem() {
super("MAC地址获取系统");
// 创建界面组件
JLabel ipLabel = new JLabel("IP地址:");
ipTextField = new JTextField(15);
JButton getMacButton = new JButton("获取MAC地址");
resultLabel = new JLabel();
// 设置布局
setLayout(new FlowLayout());
// 添加组件到界面
add(ipLabel);
add(ipTextField);
add(getMacButton);
add(resultLabel);
// 设置按钮点击事件
getMacButton.addActionListener(new ActionListener() {
@Override
public void actionPerformed(ActionEvent e) {
String ip = ipTextField.getText();
if (isValidIP(ip)) {
if (isInSameSubnet(ip)) {
String macAddress = getMacAddress(ip);
resultLabel.setText("MAC地址: " + macAddress);
} else {
resultLabel.setText("IP地址不在同一子网内");
}
} else {
resultLabel.setText("无效的IP地址");
}
}
});
}
private boolean isValidIP(String ip) {
try {
InetAddress.getByName(ip);
return true;
} catch (UnknownHostException e) {
return false;
}
}
private boolean isInSameSubnet(String ip) {
try {
InetAddress localHost = InetAddress.getLocalHost();
NetworkInterface networkInterface = NetworkInterface.getByInetAddress(localHost);
short networkPrefixLength = networkInterface.getInterfaceAddresses().get(0).getNetworkPrefixLength();
byte[] localAddress = localHost.getAddress();
byte[] targetAddress = InetAddress.getByName(ip).getAddress();
for (int i = 0; i < networkPrefixLength; i++) {
if (localAddress[i] != targetAddress[i]) {
return false;
}
}
} catch (UnknownHostException | SocketException e) {
e.printStackTrace();
}
return true;
}
private String getMacAddress(String ip) {
StringBuilder sb = new StringBuilder();
try {
InetAddress ipAddress = InetAddress.getByName(ip);
NetworkInterface networkInterface = NetworkInterface.getByInetAddress(ipAddress);
byte[] macAddressBytes = networkInterface.getHardwareAddress();
for (int i = 0; i < macAddressBytes.length; i++) {
sb.append(String.format("%02X%s", macAddressBytes[i], (i < macAddressBytes.length - 1) ? "-" : ""));
}
} catch (UnknownHostException | SocketException e) {
e.printStackTrace();
}
return sb.toString();
}
public static void main(String[] args) {
MacAddressSystem macAddressSystem = new MacAddressSystem();
macAddressSystem.setSize(300, 150);
macAddressSystem.setDefaultCloseOperation(JFrame.EXIT_ON_CLOSE);
macAddressSystem.setVisible(true);
}
}
```
这段代码创建了一个继承自JFrame的`MacAddressSystem`类,其中包括了IP地址输入框、结果显示标签和一个获取MAC地址的按钮。当用户点击获取MAC地址按钮时,程序会判定输入的IP地址的合法性和是否与本地主机在同一子网内,并根据结果显示相应的信息。
你可以根据需要进行进一步的界面美化和功能拓展。
springboot获取mac地址
要获取MAC地址,可以通过Java的NetworkInterface类来实现。下面是一个使用Spring Boot获取MAC地址的示例代码:
```java
import org.springframework.stereotype.Component;
import java.net.InetAddress;
import java.net.NetworkInterface;
import java.net.SocketException;
import java.net.UnknownHostException;
@Component
public class MacAddressUtil {
public String getMacAddress() throws UnknownHostException, SocketException {
InetAddress ipAddress = InetAddress.getLocalHost();
NetworkInterface networkInterface = NetworkInterface.getByInetAddress(ipAddress);
byte[] macAddressBytes = networkInterface.getHardwareAddress();
StringBuilder macAddressBuilder = new StringBuilder();
for (int i = 0; i < macAddressBytes.length; i++) {
macAddressBuilder.append(String.format("%02X", macAddressBytes[i]));
if (i < macAddressBytes.length - 1) {
macAddressBuilder.append("-");
}
}
return macAddressBuilder.toString();
}
}
```
在上面的代码中,我们通过调用`getMacAddress()`方法来获取MAC地址,并使用`String`类型的返回值将其返回。要使用此代码,您需要在Spring Boot应用程序中注入`MacAddressUtil`组件并调用其`getMacAddress()`方法。
阅读全文
相关推荐
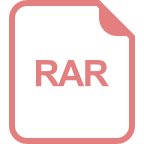
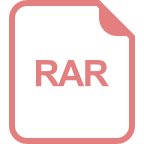
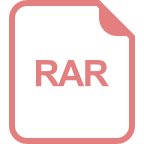
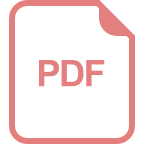
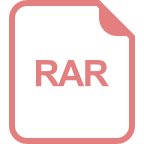
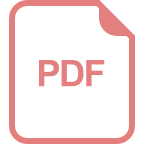
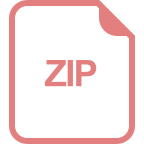
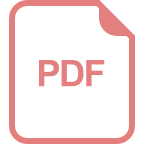
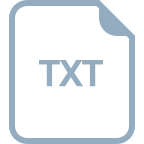
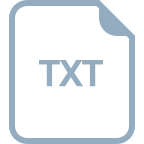
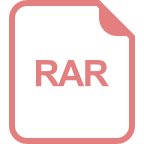
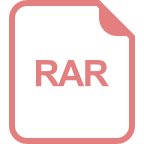
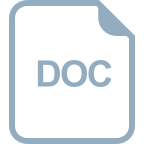