public static String f(int a) { Random s = new Random(); String cout = ""; for (int i = 0; i < a; i++) { int w = s.nextInt(); if (w>=0 && w<= 9 || w>='A' && w<='Z'|| w>='a' && w<='z'){ char zq = (char) ((char) s.nextInt(75) + 48); cout += zq; }else { i--; } } return cout; }依然输出特殊字符
时间: 2024-02-14 14:18:15 浏览: 47
在这段代码中,字符的随机生成是通过生成随机的ASCII码值来实现的,但是在生成ASCII码值时条件判断不完整,会导致生成了一些特殊字符。
要避免生成特殊字符,可以修改代码中的if语句,增加对生成的ASCII码值的判断,只生成数字和字母的ASCII码值。修改后的代码如下:
```java
public static String f(int a) {
Random s = new Random();
String cout = "";
for (int i = 0; i < a; i++) {
int w = s.nextInt();
if ((w >= '0' && w <= '9') || (w >= 'A' && w <= 'Z') || (w >= 'a' && w <= 'z')) {
char zq = (char) ((char) s.nextInt(75) + 48);
cout += zq;
} else {
i--;
}
}
return cout;
}
```
在修改后的代码中,我们增加了对生成的ASCII码值的判断,只有在生成的值为数字或字母的ASCII码值时才会生成字符,并将其添加到输出字符串中。如果生成的值不是数字或字母的ASCII码值,则不生成字符,将循环变量i减1,重新生成字符。这样就可以避免输出特殊字符了。
相关问题
public static String f(int a) { Random s = new Random(); String cout = ""; for (int i = 0; i < a; i++) { int w = s.nextInt(); if (int w>0 && w<= 9){ char z = (char) ((char) s.nextInt(75) + 48); cout += z; } } return cout; } }报错
这段代码中存在语法错误。具体来说,以下两行代码有问题:
```
int w = s.nextInt();
if (int w>0 && w<= 9){
```
第一行中,应该给 `nextInt()` 方法传入一个参数,指定一个随机数的上界。比如,如果想要生成 0 到 9 之间的随机数,应该写成 `s.nextInt(10)`。
第二行中,应该去掉 `int` 这个关键字。因为 `w` 变量已经在上一行定义过了,不能再次声明。
修正后的代码应该如下所示:
```
public static String f(int a) {
Random s = new Random();
String cout = "";
for (int i = 0; i < a; i++) {
int w = s.nextInt(10);
if (w > 0 && w <= 9) {
char z = (char) ((char) s.nextInt(75) + 48);
cout += z;
}
}
return cout;
}
```
public class Anli2 { public static void main(String[] args) { // Scanner t = new Scanner(System.in); // int i = t.nextInt(); System.out.println(f(5)); } public static String f(int a) { Random s = new Random(); String cout = ""; for (int i = 0; i < a; i++) { int w = s.nextInt(); if (w>0 && w<= 9){ w = (char) ((char) s.nextInt(75) + 48); cout += w; } } return cout; } }没有打印内容
在你的代码中,你调用了 `System.out.println(f(5))` 来输出一个字符串,但是你的 `f` 方法内部存在问题,导致 `f` 方法返回的字符串为空。具体来说,以下两行代码有问题:
```
if (w>0 && w<= 9){
w = (char) ((char) s.nextInt(75) + 48);
```
第一行中,`w` 变量是用来保存生成的随机数的,但是它的取值范围是 `-2147483648` 到 `2147483647`,并不是 1 到 9。因此,你需要修改这个条件,让它只保留 1 到 9 的随机数。
第二行中,你将 `w` 变量重新赋值为一个字符,这显然是错误的。因为你要生成一个随机的数字字符,应该使用 `48` 到 `57` 之间的随机数,然后将它转换成字符类型。具体来说,你可以使用 `(char) (s.nextInt(10) + 48)` 来生成随机的数字字符。
修正后的代码应该如下所示:
```
public class Anli2 {
public static void main(String[] args) {
System.out.println(f(5));
}
public static String f(int a) {
Random s = new Random();
String cout = "";
for (int i = 0; i < a; i++) {
int w = s.nextInt(9) + 1;
char z = (char) (w + 48);
cout += z;
}
return cout;
}
}
```
这个代码会生成一个长度为 5 的随机数字串,并将其输出到控制台上。
阅读全文
相关推荐
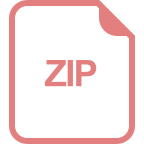
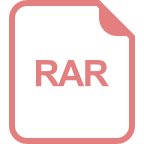
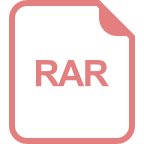
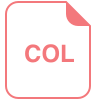
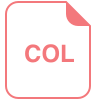



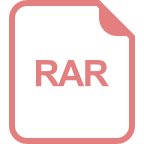
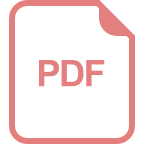
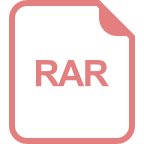
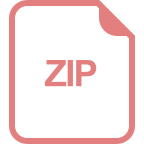
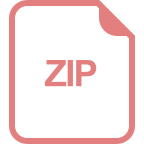
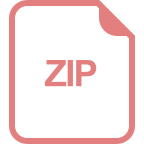
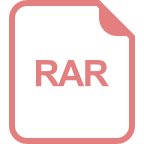
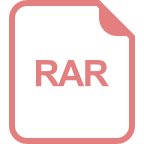