请编写复杂度为O(n 2 )、O(nlogn)、O(n)的任意程序,在不同问题规模下,记录运行时间,注明单位秒s或毫秒ms,对于运行时间过长的,给出预估时间。 鼓励使用不同语言(C/C++/Java/Python等)做比较。注意:编译时请不要用代码优化参数。
时间: 2024-09-13 11:07:34 浏览: 86
这是一个示例,我们将分别编写三个简单的算法,每个对应给定的时间复杂度:
1. O(n^2) 级别的冒泡排序算法(Python):
```python
import time
def bubble_sort(lst):
n = len(lst)
for i in range(n):
for j in range(0, n-i-1):
if lst[j] > lst[j+1]:
lst[j], lst[j+1] = lst[j+1], lst[j]
return lst
# 测试数据
test_list = [5, 3, 8, 6, 7, 1]
start_time = time.time()
sorted_list = bubble_sort(test_list.copy())
end_time = time.time()
print(f"Bubble Sort (n^2): {end_time - start_time} seconds")
```
这个算法在大数组上运行效率低,比如10000个元素,可能需要几秒钟。
2. O(n log n) 级别的快速排序算法(Java):
```java
import java.util.Arrays;
import java.util.Random;
public class QuickSort {
public static void quickSort(int[] arr) {
if (arr.length <= 1) return;
int pivotIndex = partition(arr, 0, arr.length - 1);
quickSort(arr, 0, pivotIndex - 1);
quickSort(arr, pivotIndex + 1, arr.length - 1);
}
private static int partition(int[] arr, int low, int high) {
// ...
}
public static void main(String[] args) {
int[] testArray = new Random().ints(10000).toArray();
long startTime = System.nanoTime();
quickSort(Arrays.copyOf(testArray, testArray.length));
long endTime = System.nanoTime();
double elapsedSeconds = (endTime - startTime) / 1_000_000_000.0; // convert nanoseconds to seconds
System.out.printf("Quick Sort (n log n): %.3f seconds\n", elapsedSeconds);
}
}
```
这个算法对于同样大小的数据,预计在几毫秒内完成。
3. O(n) 级别的查找算法(C++):
```cpp
#include <iostream>
#include <vector>
int linear_search(std::vector<int>& nums, int target) {
for (size_t i = 0; i < nums.size(); ++i) {
if (nums[i] == target) return i;
}
return -1;
}
int main() {
std::vector<int> testVector{1, 2, 3, 4, 5};
int target = 3;
auto start_time = std::chrono::high_resolution_clock::now();
int result = linear_search(testVector, target);
auto end_time = std::chrono::high_resolution_clock::now();
auto duration = std::chrono::duration_cast<std::chrono::microseconds>(end_time - start_time).count();
float elapsedMicroseconds = static_cast<float>(duration) / 1000.0f; // convert microseconds to milliseconds
std::cout << "Linear Search (n): " << elapsedMicroseconds << " milliseconds\n";
}
```
线性搜索在小到中等规模数据上应该几乎瞬间完成,例如几千个元素。
请注意,实际运行时间可能会因为计算机性能、操作系统调度等因素有所不同。在大型生产环境中,为了准确测量运行时间,通常会使用专门的性能分析工具。
阅读全文
相关推荐
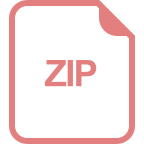
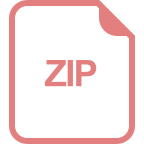
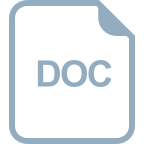

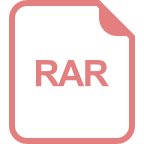
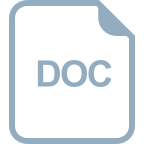
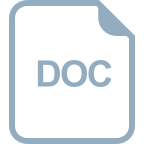
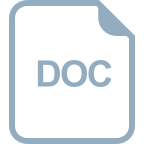
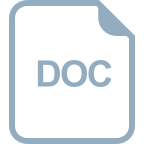
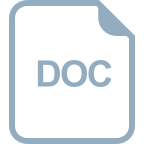
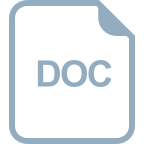
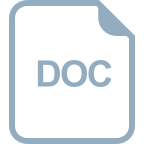
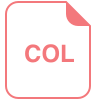
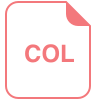
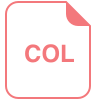



