将字符串s中的字符按正序存放到字符串t中, 然后把s中的字符按逆序连接到字符串t的后面。 例如,当s中的字行串为"ABCDE”时 则t中的字符串应为"ABCDEEDCBA”。char s[100],t[100],i,j,s1;
时间: 2024-04-29 10:22:19 浏览: 40
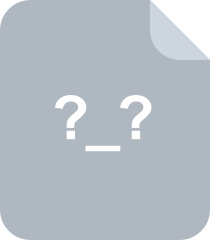
输入一个字符串,把这个字符串按逆序重新存放

可以使用字符串函数 strlen() 和 strcat() 来实现这个功能。具体代码如下:
```c
#include <stdio.h>
#include <string.h>
int main()
{
char s[100], t[100];
int i, j, len;
printf("请输入字符串s:");
scanf("%s", s);
len = strlen(s);
for (i = 0; i < len; i++) {
t[i] = s[i]; // 正序存放到t中
}
for (j = 0; j < len; j++) {
t[i+j] = s[len-j-1]; // 倒序连接到t的后面
}
t[i+j] = '\0'; // 字符串末尾加上'\0'
printf("连接后的字符串t为:%s\n", t);
return 0;
}
```
输入:
```
请输入字符串s:ABCDE
```
输出:
```
连接后的字符串t为:ABCDEEDCBA
```
阅读全文
相关推荐














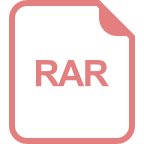
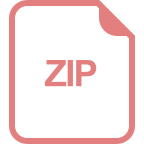