生成一个get请求的http工具类
时间: 2023-11-12 09:07:12 浏览: 58
以下是一个简单的 Java 工具类,可用于发送 GET 请求并返回响应:
```java
import java.io.BufferedReader;
import java.io.IOException;
import java.io.InputStreamReader;
import java.net.HttpURLConnection;
import java.net.URL;
public class HttpUtils {
/**
* 发送 GET 请求并返回响应
*
* @param urlStr 请求的URL字符串
* @return 响应字符串
* @throws IOException
*/
public static String sendGet(String urlStr) throws IOException {
StringBuilder result = new StringBuilder();
URL url = new URL(urlStr);
HttpURLConnection conn = (HttpURLConnection) url.openConnection();
conn.setRequestMethod("GET");
conn.setUseCaches(false);
conn.setConnectTimeout(5000);
conn.setReadTimeout(5000);
BufferedReader reader = new BufferedReader(new InputStreamReader(conn.getInputStream()));
String line;
while ((line = reader.readLine()) != null) {
result.append(line);
}
reader.close();
conn.disconnect();
return result.toString();
}
}
```
使用示例:
```java
try {
String response = HttpUtils.sendGet("https://www.example.com/api/v1/products?category=shoes");
System.out.println(response);
} catch (IOException e) {
e.printStackTrace();
}
```
注意:在实际应用中,需要根据需要设置请求头、请求参数、超时时间等参数。此处仅提供了一个简单的示例。
阅读全文
相关推荐
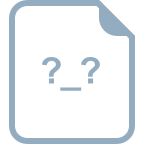
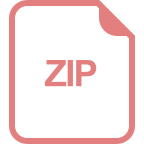
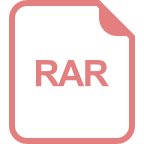
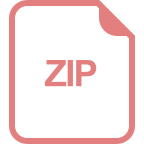
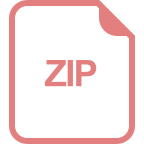
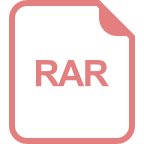
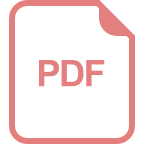
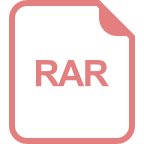
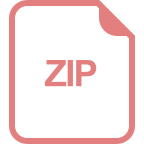
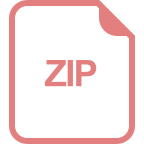
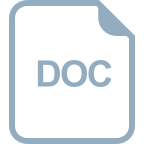
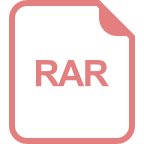
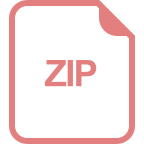
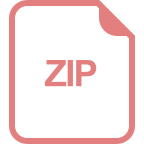
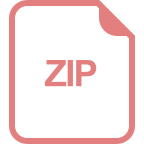