python with open('source/4wirespi.csv', 'w', newline='') as csvfile: 怎么将source/4wirespi.csv变为可变量
时间: 2023-08-08 09:07:35 浏览: 49
你可以将文件路径保存到一个变量中,然后在with语句中使用该变量,例如:
```
file_path = 'source/4wirespi.csv'
with open(file_path, 'w', newline='') as csvfile:
# do something with the file
```
现在你可以在代码中使用`file_path`变量来表示文件路径,而不需要硬编码文件路径。
相关问题
用python对该https://shenzhen.anjuke.com/sale/?from=HomePage_TopBar网站进行动态网页爬取数据并存储
好的,这也是一个非常好的问题。我们可以使用Python中的selenium和BeautifulSoup库来实现动态网页爬取和数据存储。
以下是一个简单的步骤列表:
1. 安装selenium和BeautifulSoup库。在命令行中输入`pip install selenium beautifulsoup4`即可安装。
2. 安装Chrome浏览器和对应的ChromeDriver。selenium需要与浏览器进行交互,因此需要安装Chrome浏览器和对应的ChromeDriver。你可以在此处下载:https://sites.google.com/a/chromium.org/chromedriver/downloads
3. 使用selenium打开网页并模拟操作。以下是一个示例:
```
from selenium import webdriver
from selenium.webdriver.common.keys import Keys
driver = webdriver.Chrome('/path/to/chromedriver') # 指定ChromeDriver的路径
driver.get('https://shenzhen.anjuke.com/sale/') # 打开网页
# 模拟滚动到页面底部,加载更多数据
while True:
try:
# 找到“更多”按钮并点击
more_button = driver.find_element_by_css_selector('.multi-page .a-next')
more_button.click()
except:
# 没有更多数据了
break
```
4. 使用BeautifulSoup解析HTML并提取数据。以下是一个示例:
```
from bs4 import BeautifulSoup
html = driver.page_source
soup = BeautifulSoup(html, 'html.parser')
# 找到所有房源信息
listings = soup.find_all('li', class_='list-item')
# 遍历所有房源信息并提取数据
for listing in listings:
# 获取房源标题
title = listing.find('div', class_='house-title').text.strip()
# 获取房源总价
total_price = listing.find('span', class_='price-det').text.strip()
# 获取房源单价
unit_price = listing.find('span', class_='unit-price').text.strip()
# 获取房源链接
link = listing.find('a', class_='houseListTitle')['href']
# 存储房源数据
# ...
```
5. 存储数据。可以将提取的数据存储到数据库中,也可以将其存储到CSV文件中。以下是一个示例:
```
import csv
# 打开CSV文件并写入数据
with open('anjuke_listings.csv', 'w', newline='') as csvfile:
writer = csv.writer(csvfile)
writer.writerow(['标题', '总价', '单价', '链接']) # 写入表头
for listing in listings:
# 获取房源数据
# ...
# 将房源数据写入CSV文件中
writer.writerow([title, total_price, unit_price, link])
```
希望这可以帮助你开始对安居客深圳二手房网站进行动态网页爬取和数据存储。
python 把1.csv写到with文件夹
### 回答1:
假设你已经创建了一个名为`with`的文件夹,可以使用以下代码将`1.csv`文件写入该文件夹中:
```python
import csv
with open('with/1.csv', 'w', newline='') as csvfile:
writer = csv.writer(csvfile)
writer.writerow(['Column1', 'Column2', 'Column3'])
writer.writerow(['Value1', 'Value2', 'Value3'])
```
这段代码使用了Python内置的`csv`模块,通过`open()`函数打开`with/1.csv`文件并将其设置为写入模式(`'w'`)。然后,使用`csv.writer()`函数创建一个`writer`对象,并使用`writerow()`方法将数据写入文件中。注意,`newline=''`参数可以用来避免写入CSV文件时出现空行。最后,使用`with`语句来确保在写入完成后正确关闭文件。
### 回答2:
在Python中,将1.csv文件写入with文件夹可以通过以下步骤完成。
首先,我们需要导入所需的模块,包括`os`模块和`shutil`模块。
```python
import os
import shutil
```
接下来,我们创建一个名为`with`的文件夹,并将其路径保存在一个变量中。
```python
folder_path = './with' # with文件夹的路径
os.makedirs(folder_path, exist_ok=True) # 创建with文件夹,如果已经存在则忽略
```
然后,通过使用`shutil`模块的`copy`函数,将1.csv文件复制到with文件夹中。
```python
source_file = './1.csv' # 1.csv文件的路径
destination_file = os.path.join(folder_path, '1.csv') # 目标文件的路径
shutil.copy(source_file, destination_file) # 复制1.csv文件到with文件夹中
```
最后,我们可以通过检查with文件夹中是否存在1.csv文件来验证操作是否成功。
```python
if os.path.exists(destination_file):
print("成功将1.csv文件写入with文件夹。")
else:
print("写入操作失败,请检查路径和权限。")
```
以上是将1.csv文件写入with文件夹的Python代码示例,确保在运行代码之前,1.csv文件存在于当前工作目录中。
相关推荐
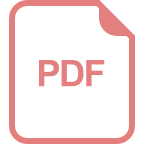
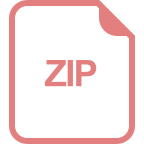
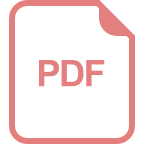











利用python爬虫,提取C:/Users/test/Desktop/DIDItest文件夹下多个文件内的html文件源代码,并提取源代码中的ID、时间、发送号码、接收号码、发送内容,如果发送内容为音频则提取音频所在位置,反之则保留发送内容,并将爬取的内容写入csv中 网页内源代码如下: <html> <meta http-equiv="Content=-Type" content="text/html; charset=utf-8"> <head> </head> <body>[talkid:138031361]2014年4月20日 03:55:45 , 434343 向 232323 发送 我们已经是好友了,开始聊天吧! () [talkid:138031362]2014年4月20日 04:45:45 , 434343 向 123456 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.m4a"]>音频
() [talkid:138031363]2014年4月20日 04:55:45 , 434343 向 123456 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.jpg"]>图片 () [talkid:138031364]2014年4月20日 05:55:45 , 434343 向 3234221 发送 我们已经是好友了,开始聊天吧! () [talkid:138031365]2014年4月20日 06:55:45 , 434343 向 1359075 发送 我们已经是好友了,开始聊天吧! () </body> </html>
网页内源代码模板如下: <html> <meta http-equiv="Content=-Type" content="text/html; charset=utf-8"> <head> </head> <body>[talkid:138031361]2014年4月20日 03:55:45 , 434343 向 232323 发送 我们已经是好友了,开始聊天吧! () [talkid:138031362]2014年4月20日 04:45:45 , 434343 向 123456 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.m4a"]>音频
() [talkid:138031363]2014年4月20日 04:55:45 , 434343 向 123456 发送 音频 :[<ahref="files/f/f123fsasfsfsjdfrhf_n.jpg"]>图片 () [talkid:138031364]2014年4月20日 05:55:45 , 434343 向 3234221 发送 我们已经是好友了,开始聊天吧! () [talkid:138031365]2014年4月20日 06:55:45 , 434343 向 1359075 发送 我们已经是好友了,开始聊天吧! () </body> </html> 利用python爬虫,打开C:/Users/test/Desktop/DIDItest文件夹下多个文件夹内的html文件源代码,并爬取源代码中的ID、时间、发送号码、接收号码、发送内容,如果发送内容为音频则提取音频所在位置,反之则保留发送内容,并将爬取的内容写入csv中
