蝴蝶曲线如何用代码实现
时间: 2023-09-07 12:05:00 浏览: 203
### 回答1:
蝴蝶曲线可以通过以下代码实现:
```python
import matplotlib.pyplot as plt
import numpy as np
t = np.linspace(0, 2*np.pi, 1000)
x = np.sin(t)*(np.exp(np.cos(t))-2*np.cos(4*t)-np.sin(t/12)**5)
y = np.cos(t)*(np.exp(np.cos(t))-2*np.cos(4*t)-np.sin(t/12)**5)
plt.plot(x, y)
plt.axis('equal')
plt.show()
```
这段代码使用了 NumPy 和 Matplotlib 库,通过参数方程来计算蝴蝶曲线上的点,并使用 Matplotlib 绘制出曲线。
### 回答2:
要用代码实现蝴蝶曲线,需要使用数学库和绘图库。
首先,我们需要导入所需的库,如NumPy和Matplotlib。然后,创建一个空的图形对象,并定义x和y的范围。
接下来,我们要定义蝴蝶曲线的方程。蝴蝶曲线的方程可以使用参数方程表示。例如:x = sin(t) * (exp(cos(t)) - 2*cos(4*t) - sin(t/12)^5),y = cos(t) * (exp(cos(t)) - 2*cos(4*t) - sin(t/12)^5)。
然后,在一个循环中,从0到2π之间取一系列的t值,并计算对应的x和y坐标。我们使用NumPy库来计算sin、cos和exp函数的值。
接下来,将计算得到的坐标点添加到图形对象中。
最后,通过调用绘图库的绘图函数,将蝴蝶曲线显示出来。
以下是代码实现的一个示例:
```python
import numpy as np
import matplotlib.pyplot as plt
# 创建一个空的图形对象
fig = plt.figure()
# 定义x和y的范围
xmin, xmax = -5, 5
ymin, ymax = -3, 3
# 定义蝴蝶曲线的方程
def butterfly_curve(t):
x = np.sin(t) * (np.exp(np.cos(t)) - 2 * np.cos(4 * t) - np.sin(t / 12) ** 5)
y = np.cos(t) * (np.exp(np.cos(t)) - 2 * np.cos(4 * t) - np.sin(t / 12) ** 5)
return x, y
# 生成一系列的t值
t = np.linspace(0, 2 * np.pi, 1000)
# 计算对应的x和y坐标
x, y = butterfly_curve(t)
# 将坐标点添加到图形对象
plt.plot(x, y)
# 设置x和y的范围
plt.xlim(xmin, xmax)
plt.ylim(ymin, ymax)
# 显示蝴蝶曲线
plt.show()
```
运行这段代码,就可以得到蝴蝶曲线的图形。根据需要,可以调整方程中的参数以及x和y的范围,以获得不同形状和大小的蝴蝶曲线图形。
相关推荐
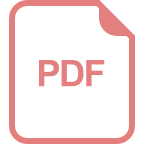
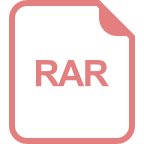














