编写代码实现java注解
时间: 2024-01-26 11:08:36 浏览: 26
下面是一个简单的Java注解的示例代码:
```java
import java.lang.annotation.*;
@Retention(RetentionPolicy.RUNTIME)
@Target(ElementType.METHOD)
public @interface MyAnnotation {
String value();
int number() default 0;
}
```
这个注解有两个属性:`value`和`number`。其中`value`属性是必需的,而`number`属性是可选的,默认值为0。
下面是一个使用这个注解的示例:
```java
public class MyClass {
@MyAnnotation(value = "hello", number = 42)
public void myMethod() {
// method body
}
}
```
在这个例子中,`MyClass`类中的`myMethod()`方法被注解为`@MyAnnotation(value = "hello", number = 42)`。这意味着这个方法有一个`value`属性,值为`"hello"`,还有一个`number`属性,值为`42`。
相关问题
编写代码实现Java注解
好的,以下是一段用于自定义Java注解的示例代码:
```java
import java.lang.annotation.ElementType;
import java.lang.annotation.Retention;
import java.lang.annotation.RetentionPolicy;
import java.lang.annotation.Target;
@Retention(RetentionPolicy.RUNTIME) // 设置注解保留时间为运行时
@Target(ElementType.METHOD) // 设置注解适用范围为方法
public @interface MyAnnotation {
String name() default ""; // 定义注解属性name,默认值为""
int age() default 0; // 定义注解属性age,默认值为0
}
```
这段代码定义了一个名为MyAnnotation的注解,该注解有两个属性:name和age。其中,name属性类型为String,age属性类型为int,两个属性都有默认值。
接下来是一个使用该注解的示例:
```java
public class MyClass {
@MyAnnotation(name = "张三", age = 20) // 使用自定义注解,设置name和age属性值
public void doSomething() {
// 方法内容
}
}
```
这段代码中,我们在doSomething()方法上使用了自定义注解MyAnnotation,并设置了name和age属性的值。在编译时,注解信息会被编译器存储在class文件中,运行时可以通过反射机制获取到注解信息。
编写Java代码,实现hive UDTF函数
好的,下面是一个简单的 Java 代码示例,演示如何实现一个 Hive UDTF 函数。
```java
import org.apache.hadoop.hive.ql.exec.UDFArgumentException;
import org.apache.hadoop.hive.ql.exec.UDFArgumentLengthException;
import org.apache.hadoop.hive.ql.exec.UDFArgumentTypeException;
import org.apache.hadoop.hive.ql.exec.UDFType;
import org.apache.hadoop.hive.ql.udf.generic.GenericUDTF;
import org.apache.hadoop.hive.serde2.objectinspector.ObjectInspector;
import org.apache.hadoop.hive.serde2.objectinspector.StructField;
import org.apache.hadoop.hive.serde2.objectinspector.StructObjectInspector;
import org.apache.hadoop.hive.serde2.objectinspector.primitive.PrimitiveObjectInspectorFactory;
import org.apache.hadoop.hive.serde2.objectinspector.primitive.StringObjectInspector;
import org.apache.hadoop.io.Text;
import java.util.ArrayList;
import java.util.List;
@UDFType(deterministic = true, stateful = false)
public class MyUDTF extends GenericUDTF {
private StringObjectInspector inputOI = null;
@Override
public StructObjectInspector initialize(ObjectInspector[] args) throws UDFArgumentException {
if (args.length != 1) {
throw new UDFArgumentLengthException("MyUDTF takes only one argument");
}
if (args[0].getCategory() != ObjectInspector.Category.PRIMITIVE
|| !args[0].getTypeName().equals("string")) {
throw new UDFArgumentTypeException(0, "Argument 1 must be a string");
}
inputOI = (StringObjectInspector) args[0];
// Define the output column names and types
List<String> fieldNames = new ArrayList<String>();
List<ObjectInspector> fieldOIs = new ArrayList<ObjectInspector>();
fieldNames.add("word");
fieldOIs.add(PrimitiveObjectInspectorFactory.writableStringObjectInspector);
return ObjectInspectorFactory.getStandardStructObjectInspector(fieldNames, fieldOIs);
}
@Override
public void process(Object[] args) throws HiveException {
String input = inputOI.getPrimitiveJavaObject(args[0]).toString();
String[] words = input.split(" ");
for (String word : words) {
Text t = new Text(word);
forward(new Object[]{t});
}
}
@Override
public void close() throws HiveException {
// do nothing
}
}
```
在上面的代码中,我们定义了一个名为 `MyUDTF` 的类,继承了 Hive 的 `GenericUDTF` 类,并重写了其中的 `initialize`、`process` 和 `close` 方法。
其中,`initialize` 方法用于初始化 UDTF 函数,我们在这里检查函数的输入参数是否符合要求,并定义了函数的输出列名和类型;`process` 方法用于处理每一行输入数据,并将结果输出;`close` 方法用于清理资源。
具体实现中,我们将输入字符串按空格分割成单词,并将每个单词作为一个输出行返回。在 `forward` 方法中,我们将每个单词封装成一个 `Text` 类型的对象,并通过 `Object[]` 数组的形式传递给 Hive 引擎。
需要注意的是,在类上我们添加了 `@UDFType` 注解,指定了该 UDTF 函数是确定性的(即相同的输入一定产生相同的输出)和无状态的(即不依赖于之前的输入状态)。这个注解可以让 Hive 引擎更好地优化查询执行计划。
相关推荐
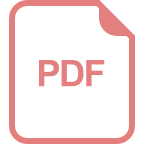
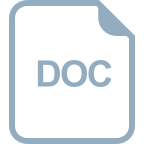












