利用transformer压缩图片后输入100维的向量的代码
时间: 2023-03-22 20:00:38 浏览: 51
以下是利用transformer压缩图片后输入100维的向量的代码:
首先,我们需要导入必要的库:
```python
import torch
import torch.nn as nn
import torch.nn.functional as F
from torchvision.transforms import transforms
```
然后,我们定义一个用于压缩图片的TransformerEncoder模型:
```python
class ImageTransformerEncoder(nn.Module):
def __init__(self, input_dim, num_layers, num_heads, hidden_dim, output_dim):
super(ImageTransformerEncoder, self).__init__()
self.positional_encoding = nn.Parameter(torch.zeros(1, input_dim, hidden_dim))
nn.init.normal_(self.positional_encoding, mean=0, std=0.1)
self.layers = nn.ModuleList([nn.TransformerEncoderLayer(d_model=hidden_dim, nhead=num_heads) for _ in range(num_layers)])
self.fc = nn.Linear(hidden_dim, output_dim)
def forward(self, x):
b, c, h, w = x.shape
x = x.view(b, c, h*w).permute(0, 2, 1) # (b, h*w, c)
x = x + self.positional_encoding[:, :h*w, :]
for layer in self.layers:
x = layer(x)
x = x.mean(dim=1)
x = self.fc(x)
return x
```
其中,`input_dim`表示输入图片的通道数,`num_layers`表示TransformerEncoder中包含的EncoderLayer的数量,`num_heads`表示每个EncoderLayer中的多头注意力机制头数,`hidden_dim`表示EncoderLayer中隐藏层的维度,`output_dim`表示最终输出的向量维度。
在模型的前向传播中,我们将输入图片reshape成`(batch_size, height*width, channel)`的形状,并加上位置编码后输入TransformerEncoder中。最后,我们将TransformerEncoder的输出取平均并经过一个全连接层得到最终的100维向量。
最后,我们定义一个图片压缩函数,该函数将输入图片通过ImageTransformerEncoder模型压缩成100维向量:
```python
def compress_image(image_path):
transform = transforms.Compose([
transforms.Resize((256, 256)),
transforms.ToTensor(),
transforms.Normalize(mean=[0.485, 0.456, 0.406], std=[0.229, 0.224, 0.225])
])
image = Image.open(image_path)
image = transform(image).unsqueeze(0)
model = ImageTransformerEncoder(input_dim=3, num_layers=6, num_heads=8, hidden_dim=512, output_dim=100)
model.load_state_dict(torch.load('image_transformer_encoder.pth', map_location=torch.device('cpu')))
model.eval()
with torch.no_grad():
output = model(image)
return output
```
其中,我们还使用了`PIL`库中的`Image`类来读取图片,并通过`transforms`模块对图片进行预处理。在模型的使用过程中,我们需要将模型加载进来,并将模型设为评估模式。最后,我们通过模型将输入图片压缩成100维向量并返回。
相关推荐
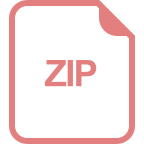














