写一段基于遗传算法进行波束赋形的代码
时间: 2023-06-14 13:02:33 浏览: 110
以下是一个示例遗传算法进行波束赋形的代码:
```python
import numpy as np
# 定义目标函数,即波束方向图
def objective_function(theta, a):
N = len(theta)
a_theta = np.zeros(N, dtype=complex)
for i in range(N):
a_theta[i] = np.exp(1j*np.pi*np.sin(theta[i]))
return np.abs(np.dot(a, a_theta))
# 定义遗传算法参数
POP_SIZE = 50
GENE_SIZE = 20
CROSS_RATE = 0.8
MUTATION_RATE = 0.01
N_GENERATIONS = 500
# 初始化种群
pop = np.random.randint(2, size=(POP_SIZE, GENE_SIZE))
# 定义交叉函数
def crossover(parent, pop):
if np.random.rand() < CROSS_RATE:
i_ = np.random.randint(0, POP_SIZE, size=1)
cross_points = np.random.randint(0, 2, size=GENE_SIZE).astype(np.bool)
parent[cross_points] = pop[i_, cross_points]
return parent
# 定义变异函数
def mutation(child):
for point in range(GENE_SIZE):
if np.random.rand() < MUTATION_RATE:
child[point] = 1 if child[point] == 0 else 0
return child
# 开始迭代
for generation in range(N_GENERATIONS):
# 计算适应度
fitness = np.zeros(POP_SIZE)
for i in range(POP_SIZE):
theta = np.linspace(-np.pi/2, np.pi/2, GENE_SIZE)[pop[i]==1]
fitness[i] = objective_function(theta, a)
# 选择
fitness = (fitness - np.min(fitness)) / (np.max(fitness) - np.min(fitness))
idx = np.random.choice(range(POP_SIZE), size=POP_SIZE, replace=True, p=fitness)
pop = pop[idx]
# 繁殖
for i in range(POP_SIZE // 2):
parent1, parent2 = pop[i], pop[POP_SIZE-i-1]
child1 = crossover(parent1.copy(), parent2.copy())
child2 = crossover(parent2.copy(), parent1.copy())
child1 = mutation(child1)
child2 = mutation(child2)
pop[i] = child1
pop[POP_SIZE-i-1] = child2
# 输出结果
theta = np.linspace(-np.pi/2, np.pi/2, GENE_SIZE)[pop[np.argmax(fitness)]==1]
print("Optimal theta:", theta)
```
其中,`objective_function` 函数用于计算波束方向图的适应度,`crossover` 函数用于交叉操作,`mutation` 函数用于变异操作。在主函数中,首先初始化种群,然后迭代进行选择、交叉、变异等操作,最终得到最优解。
阅读全文
相关推荐
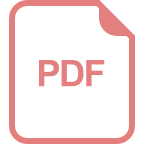

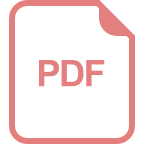
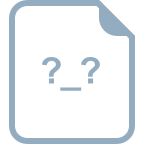
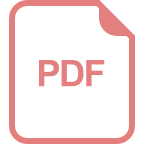
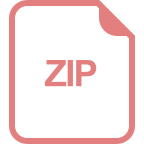
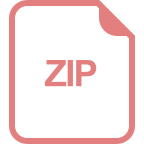
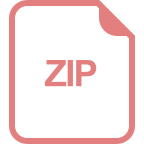
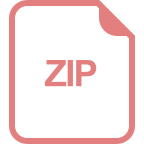
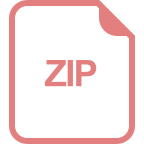
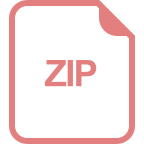
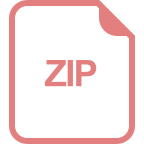
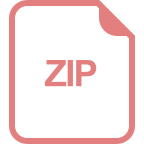
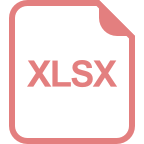