用C++ 定义一个字符数组s1,并输入一个字符串1,写一段代码,要求把字符串1中的字母拷贝到字符数组s2中并输出。然后再优化代码
时间: 2023-06-02 18:06:13 浏览: 90
可以参考以下代码:
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 100
int main() {
char s1[MAX_LEN];
char s2[MAX_LEN];
printf("请输入字符串1:\n");
scanf("%s", s1);
// 复制字符串1到字符串2
strcpy(s2, s1);
printf("拷贝后的字符串2为:%s\n", s2);
return 0;
}
```
优化代码可以使用 strncpy() 函数,该函数可以指定拷贝的长度,防止越界访问。
```c
#include <stdio.h>
#include <string.h>
#define MAX_LEN 100
int main() {
char s1[MAX_LEN];
char s2[MAX_LEN];
printf("请输入字符串1:\n");
scanf("%s", s1);
// 复制字符串1到字符串2
strncpy(s2, s1, MAX_LEN - 1);
s2[MAX_LEN - 1] = '\0';
printf("拷贝后的字符串2为:%s\n", s2);
return 0;
}
```
相关问题
C++程序中定义了2个字符数组,要求把s1复制给s2,然后输出s2
好的,以下是您要求的小程序,使用 C++ 语言编写:
```cpp
#include <iostream>
#include <cstring>
int main() {
const int max_length = 20; // 定义数组的最大长度
char s1[max_length] = "I am a student."; // 定义字符数组s1并初始化
char s2[max_length]; // 定义字符数组s2
std::strcpy(s2, s1); // 将s1复制给s2
std::cout << s2 << std::endl; // 输出s2
return 0;
}
```
程序中定义了两个字符数组 `s1` 和 `s2`,并设定其最大长度为 `max_length`。首先对 `s1` 进行初始化,然后使用 `std::strcpy()` 函数将 `s1` 复制给 `s2`。最后使用 `std::cout` 输出 `s2` 中的字符串,并添加换行符 `std::endl`。最终输出结果为:
```
I am a student.
```
输入两个字符串s1和$2。验证其中一个字符串是否是另一个字符串的子串。用c++
在C++中,验证一个字符串`s1`是否是另一个字符串`s2`的子串,可以使用`substr()`函数或者直接遍历的方式。这里是一个简单的示例:
```cpp
#include <iostream>
#include <string>
bool isSubstr(const std::string &s1, const std::string &s2) {
// 使用substr()函数检查s1是否是s2的子串
if (s2.find(s1) != std::string::npos) {
return true;
} else {
return false;
}
}
int main() {
std::string s1 = "hello";
std::string s2 = "world hello";
if (isSubstr(s1, s2)) {
std::cout << s1 << " is a substring of " << s2 << std::endl;
} else {
std::cout << s1 << " is not a substring of " << s2 << std::endl;
}
return 0;
}
```
在这个例子中,`find()`函数用于查找`s1`在`s2`中的位置。如果找到(返回值不是`std::string::npos`),则说明`s1`是`s2`的子串。
阅读全文
相关推荐
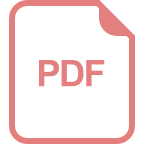
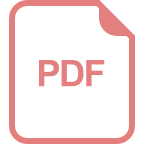
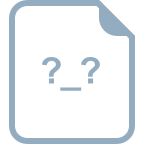













