Vue中如何实现样式的动态切换
时间: 2023-05-12 07:05:29 浏览: 89
在Vue中,可以使用v-bind:class指令来实现样式的动态切换。具体来说,可以在模板中使用v-bind:class指令来绑定一个对象,该对象的属性名是样式类名,属性值是一个布尔值,表示该样式类是否应该被应用。例如:
```
<template>
<div v-bind:class="{ active: isActive }"></div>
</template>
<script>
export default {
data() {
return {
isActive: true
}
}
}
</script>
```
在上面的例子中,如果isActive为true,那么该元素会应用active样式类;如果isActive为false,那么该元素不会应用active样式类。可以通过改变isActive的值来动态切换样式。
相关问题
vue 实现类似于tab切换样式不变重新渲染数据
在Vue中,可以使用动态绑定类的方式实现类似于tab切换样式不变重新渲染数据的效果。具体实现步骤如下:
1. 首先,在Vue的data中定义一个变量,用于表示当前选中的tab索引,例如`currentIndex`。
2. 在HTML模板中,通过v-for指令渲染tab的列表,并为每个tab项绑定一个点击事件,将当前tab的索引传递给一个方法,例如`changeTab`。
3. 在`changeTab`方法中,将传递过来的tab索引赋值给`currentIndex`变量,用于保存当前选中的tab索引。
4. 在tab的样式中,使用动态绑定类的方式来添加/移除一个表示选中状态的样式类。可以使用计算属性来根据`currentIndex`和当前tab索引的关系动态地返回一个表示是否选中的类名。
5. 根据`currentIndex`的变化来决定渲染显示哪个tab的内容。可以使用v-if或v-show指令来根据条件显示/隐藏不同tab的内容。
通过以上步骤,即可实现类似于tab切换样式不变重新渲染数据的效果。每次切换tab时,只会重新渲染指定tab的数据,并保持其他tab的样式不变。
阅读全文
相关推荐
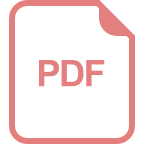
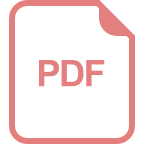
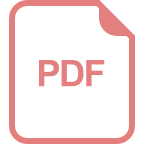
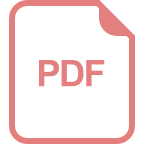
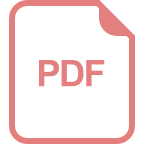
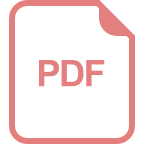
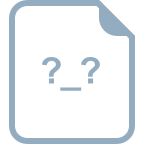
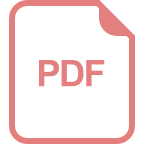
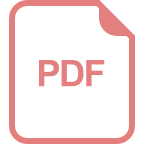
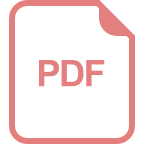
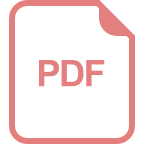
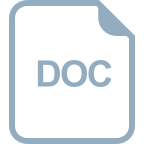
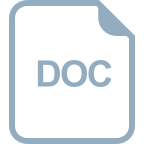
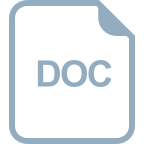
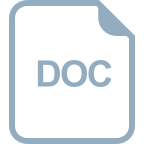
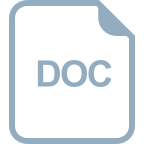
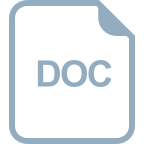
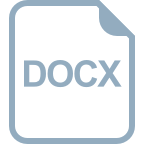