"本文将介绍如何在Vue.js框架中实现一个简单的图片查看组件,该组件支持图片左右旋转、查看上一张和下一张图片的功能。这个组件可以被集成到Element UI库中,虽然示例代码可能不够完美,但具有一定的参考价值。" 在Vue.js开发中,我们经常需要创建自定义组件来满足特定的需求。在这个案例中,我们将讨论一个用于查看图片的组件,它包含了左右旋转图片以及切换上下张图片的功能。这个组件可以在一个父组件中被调用,并且可以通过传递参数来控制显示。 首先,父组件模板部分包含了一个名为`<see-attachment>`的子组件,这个子组件会在`showmask`变量为真时显示。当用户触发查看图片的事件(例如点击某个按钮)时,`lookImg`方法会被调用,设置`showmask`为真并传递当前选中的图片对象`imgFile`给子组件。 ```html <template> <div> <see-attachment :filesLists="files" :file="imgFile" v-if="showmask" @hideMask='showmask=false'></see-attachment> </div> </template> <script> import SeeAttachment from "./seeAttachment.vue"; export default { data() { return { showmask: false, imgFile: {} }; }, components: { SeeAttachment }, methods: { lookImg(f) { this.showmask = true; this.imgFile = f; } } }; </script> ``` 接下来,我们看子组件的代码。子组件`<see-attachment>`的模板中包含了一个图片容器`<div class="img_box">`,用于显示图片,并有一个`@click="stopEvent"`事件处理器来阻止点击事件的默认行为(比如防止图片被点击后跳转)。图片的路径、宽度和高度可以通过props从父组件传递进来。 ```html <template> <div class="proview_box" @click="hideMask"> <div class="img_box"> <img :src="imgPath" :width="width" :height="height" @click="stopEvent" id='img'/> </div> </div> <!-- ... --> </template> ``` 此外,子组件还包括了四个操作图片的按钮:上一张图片、左旋转、右旋转和下一张图片。每个按钮都有对应的点击事件处理函数,如`prevOne`、`rotate(0)`、`rotate(1)`和`nextOne`。 ```html <!-- ... --> <div class="handleImg_box"> <div @click="prevOne"><img src="../../../../static/img/prev.png"/></div> <div @click="rotate(0)"><img src="../../../../static/img/turn_left.png"/></div> <div @click="rotate(1)"><img src="../../../../static/img/turn_right.png"/></div> <div @click="nextOne"><img src="../../../../static/img/next.png"/></div> </div> <!-- ... --> ``` 在子组件的JavaScript部分,我们需要实现这些方法。`rotate`方法应该接收一个参数,表示旋转的角度(0度或180度),并更新图片的CSS样式来实现旋转效果。`prevOne`和`nextOne`方法则需要更改当前显示的图片,这可以通过维护一个图片数组和索引来实现。 ```javascript <script> export default { props: { filesLists: Array, // 图片数组 file: Object, // 当前显示的图片对象 width: Number, // 图片宽度 height: Number // 图片高度 }, data() { return { imgPath: '', // 图片路径 index: 0, // 当前图片索引 rotateAngle: 0 // 旋转角度 }; }, methods: { hideMask() { // 隐藏组件 }, stopEvent(e) { e.stopPropagation(); // 阻止事件冒泡 }, rotate(direction) { this.rotateAngle += direction * 90; // 更新旋转角度 // 使用CSS transform来改变图片的旋转角度 document.getElementById('img').style.transform = `rotate(${this.rotateAngle}deg)`; }, prevOne() { // 切换到上一张图片 if (this.index > 0) { this.index--; } else { this.index = this.filesLists.length - 1; } this.imgPath = this.filesLists[this.index].url; }, nextOne() { // 切换到下一张图片 if (this.index < this.filesLists.length - 1) { this.index++; } else { this.index = 0; } this.imgPath = this.filesLists[this.index].url; } }, created() { this.imgPath = this.file.url; // 初始化图片路径 this.index = this.filesLists.indexOf(this.file); // 初始化图片索引 } }; </script> ``` 以上就是使用Vue.js实现图片查看组件的基本步骤。这个组件可以方便地集成到其他Vue项目中,为用户提供便捷的图片浏览功能。通过调整样式和增加更多功能,你可以将其优化成一个更完善的图片查看器组件。
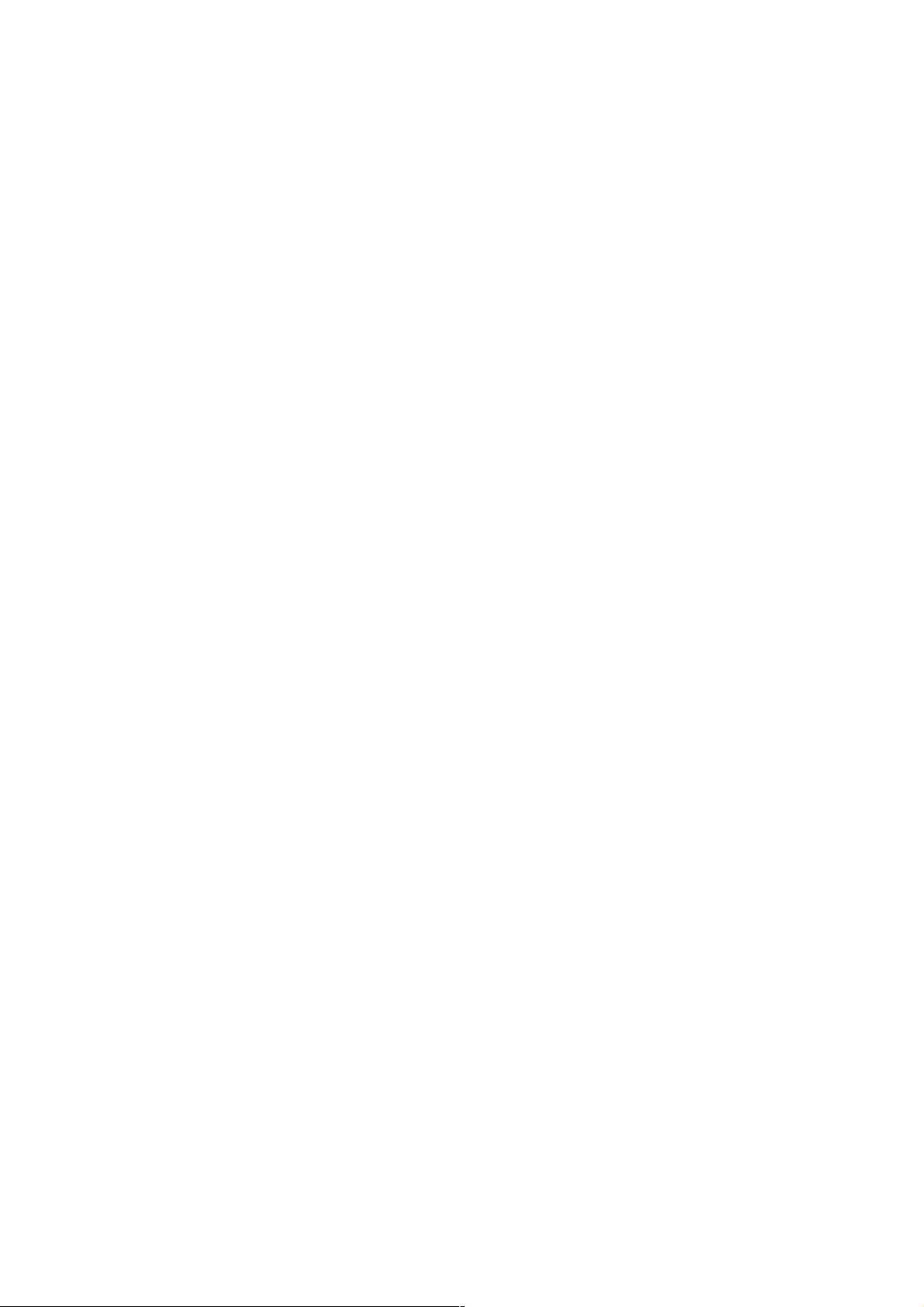
下载后可阅读完整内容,剩余3页未读,立即下载
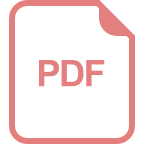
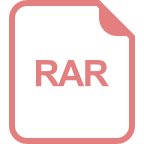

















- 粉丝: 4
- 资源: 927
我的内容管理 展开
我的资源 快来上传第一个资源
我的收益
登录查看自己的收益我的积分 登录查看自己的积分
我的C币 登录后查看C币余额
我的收藏
我的下载
下载帮助

最新资源
- C++多态实现机制详解:虚函数与早期绑定
- Java多线程与异常处理详解
- 校园导游系统:无向图实现最短路径探索
- SQL2005彻底删除指南:避免重装失败
- GTD时间管理法:提升效率与组织生活的关键
- Python进制转换全攻略:从10进制到16进制
- 商丘物流业区位优势探究:发展战略与机遇
- C语言实训:简单计算器程序设计
- Oracle SQL命令大全:用户管理、权限操作与查询
- Struts2配置详解与示例
- C#编程规范与最佳实践
- C语言面试常见问题解析
- 超声波测距技术详解:电路与程序设计
- 反激开关电源设计:UC3844与TL431优化稳压
- Cisco路由器配置全攻略
- SQLServer 2005 CTE递归教程:创建员工层级结构

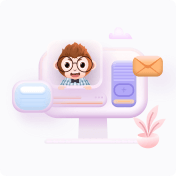
