java利用多线程技术模拟龟兔赛跑的场面,设计一个线程类模拟参与赛跑的角色,创建该类的两个对象,代表乌龟和兔子,让兔子的速度快,但休息时间长,到终点时线性运行结束
时间: 2023-12-11 12:02:14 浏览: 145
好的,以下是 Java 代码实现:
```java
public class Racing implements Runnable {
private String name; // 参赛选手名称
private int speed; // 参赛选手速度
private int restTime; // 参赛选手休息时间
private int totalDistance; // 总路程
private int currentDistance = 0; // 当前路程
public Racing(String name, int speed, int restTime, int totalDistance) {
this.name = name;
this.speed = speed;
this.restTime = restTime;
this.totalDistance = totalDistance;
}
@Override
public void run() {
try {
while (currentDistance < totalDistance) {
currentDistance += speed; // 参赛选手前进
System.out.println(name + "已经跑了" + currentDistance + "米");
if (currentDistance >= totalDistance) { // 判断是否已经到达终点
System.out.println(name + "已经到达终点!");
break;
}
if (name.equals("兔子")) { // 兔子休息
Thread.sleep(restTime);
}
}
} catch (InterruptedException e) {
e.printStackTrace();
}
}
public static void main(String[] args) {
Racing tortoise = new Racing("乌龟", 10, 0, 100); // 创建乌龟线程
Racing rabbit = new Racing("兔子", 20, 1000, 100); // 创建兔子线程
Thread t1 = new Thread(tortoise); // 创建乌龟线程对象
Thread t2 = new Thread(rabbit); // 创建兔子线程对象
t1.start(); // 启动乌龟线程
t2.start(); // 启动兔子线程
}
}
```
上述代码中,我们通过实现 `Runnable` 接口并重写 `run()` 方法来实现线程的逻辑。在 `main()` 方法中,我们创建了乌龟和兔子两个线程对象,并启动它们的线程。
在 `run()` 方法中,我们不断更新当前路程 `currentDistance`,并输出当前路程。如果当前路程已经到达终点,就输出到达终点的信息并结束线程。对于兔子,我们还要在每次更新路程后让它休息一段时间。
阅读全文
相关推荐
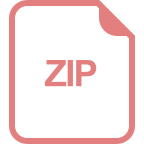
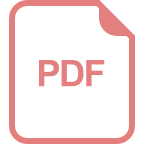














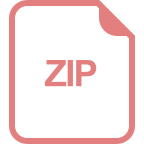
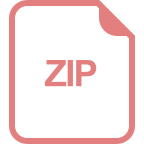