public Form1() { InitializeComponent(); } private void timer1_Tick(object sender, EventArgs e)//定时器 { string tradeTime = DateTime.Now.ToString("yyyy年MM月dd日 HH:mm:ss", System.Globalization.DateTimeFormatInfo.InvariantInfo); m_curtime.Text = tradeTime; if (m_bRuning) { //时间到 if (DateTime.Now.Second == 0 && DateTime.Now.Hour == int.Parse(m_hour.Text) && DateTime.Now.Minute == int.Parse(m_min.Text)) { m_text.Visible = true; int nIndex = m_music.SelectedIndex; WarnPlayer = new System.Media.SoundPlayer(System.Windows.Forms.Application.StartupPath + musics[nIndex]); //System.Threading.Thread t = new System.Threading.Thread(PlayWarnSound);//创建了线程 //t.Start();//开启线程 WarnPlayer.PlayLooping();//循环播放 } //持续时间判断 long lTime = GetTimeStamp(); if (lTime - m_nTimeStart >= m_nTimeLong * 1000) { if (WarnPlayer != null)//停止播放 WarnPlayer.Stop(); m_bRuning = false; m_text.Visible = false; } if (m_text.Visible == true)//重复显示黑色和红色 { m_text.Text = m_msg.Text; if (DateTime.Now.Second % 2 == 0) m_text.ForeColor = Color.Black; else m_text.ForeColor = Color.Red; } } }
时间: 2024-02-10 17:32:41 浏览: 111
这段代码中,timer1_Tick 是一个定时器事件,每隔一定时间就会执行一次。在事件处理程序中,首先获取当前时间并在界面上显示。接下来,如果计时器正在运行中,则判断是否到达预设的时间点,如果是,则显示一个文本框并开始循环播放音频文件;同时,还会根据设定的持续时间判断是否需要停止播放,并隐藏文本框。最后,如果文本框可见,则会重复显示设定的文本,并在黑色和红色之间交替显示。
相关问题
FilterInfoCollection videoDevices;//摄像头设备集合 VideoCaptureDevice videoSource;//捕获设备源 const int delaytime = 500; //扫码间隔时间 ms int totalTime; //扫码最长时间 public Form1() { InitializeComponent(); } private void comboBox1_SelectedIndexChanged(object sender, EventArgs e) { } private void button1_Click(object sender, EventArgs e) { comboBox1.Items.Clear(); videoDevices = new FilterInfoCollection(FilterCategory.VideoInputDevice); for (int i = 0; i < videoDevices.Count; i++) comboBox1.Items.Add(videoDevices[i].Name); comboBox1.Text = comboBox1.Items[0].ToString(); } private void button2_Click(object sender, EventArgs e) { if (comboBox1.Text == null) return; videoSource = new VideoCaptureDevice(videoDevices[comboBox1.SelectedIndex].MonikerString); videoSourcePlayer1.VideoSource = videoSource; videoSourcePlayer1.Start(); textBox1.Text = "开始识读"; timer1.Interval = delaytime; timer1.Start(); totalTime = 0; } private void timer1_Tick(object sender, EventArgs e) { if (totalTime >= 20000)// 最多读20秒 { timer1.Stop(); textBox1.Text = "未识别到条形码"; return; } Bitmap barcodeImage; barcodeImage = videoSourcePlayer1.GetCurrentVideoFrame();//拍摄 if (barcodeImage != null) { // 识读条形码 BarcodeReader reader = new BarcodeReader(); reader.Options.CharacterSet = "UTF-8"; reader.Options.PossibleFormats = new List<BarcodeFormat>() { BarcodeFormat.CODE_128 }; Result resultBarcode = reader.Decode(barcodeImage); if (resultBarcode != null) { textBox1.Text = ""; textBox1.AppendText(resultBarcode.Text); timer1.Stop(); return; } } totalTime += delaytime; textBox1.Text = totalTime.ToString() + " ms"; //if (totalTime == 3000) // picture.Save("测试图片.bmp");怎么设置锁定后置摄像头不打开前置摄像头
要想实现锁定后置摄像头,不打开前置摄像头,可以在获取摄像头设备源时,指定后置摄像头的设备。具体实现方法如下:
1. 获取所有视频设备的集合
```
videoDevices = new FilterInfoCollection(FilterCategory.VideoInputDevice);
```
2. 遍历视频设备集合,找到后置摄像头对应的设备
```
foreach (FilterInfo device in videoDevices)
{
if (device.Name == "后置摄像头名称")
{
videoSource = new VideoCaptureDevice(device.MonikerString);
break;
}
}
```
3. 如果找到后置摄像头对应的设备,则使用该设备进行视频捕捉,否则提示用户没有找到后置摄像头
```
if (videoSource != null)
{
videoSourcePlayer1.VideoSource = videoSource;
videoSourcePlayer1.Start();
}
else
{
MessageBox.Show("没有找到后置摄像头!");
}
```
需要注意的是,不同手机的后置摄像头名称可能不同,需要根据具体手机进行调试和修改。同时,如果找不到后置摄像头对应的设备,则需要在代码中处理异常情况。
public partial class Form1 : Form { private ushort _CardID = 0; public Form1() { InitializeComponent(); } private void Form1_Load(object sender, EventArgs e) { short num = LTDMC.dmc_board_init();//获取卡数量 if (num <= 0 || num > 8) { MessageBox.Show("初始卡失败!", "出错"); } ushort _num = 0; ushort[] cardids = new ushort[8]; uint[] cardtypes = new uint[8]; short res = LTDMC.dmc_get_CardInfList(ref _num, cardtypes, cardids); if (res != 0) { MessageBox.Show("获取卡信息失败!"); } _CardID = cardids[0]; // timer1.Start(); }
这段代码是一个 Windows 窗体应用程序中的 Form1 类,它的 Load 事件处理程序。该处理程序使用了 LTDMC 库来初始化运动控制卡,并获取卡的信息。
首先,使用 LTDMC 库中的 dmc_board_init() 函数获取卡数量,如果数量不在 1 到 8 之间,则显示一个错误消息框。
然后,使用 LTDMC 库中的 dmc_get_CardInfList() 函数获取每个卡的类型和 ID,如果获取失败,则显示一个错误消息框。
最后,将第一个卡的 ID 存储在私有变量 _CardID 中,并启动一个计时器 timer1。
这段代码的目的是初始化运动控制卡,并获取第一个卡的 ID,以便在后续的代码中使用。
阅读全文
相关推荐
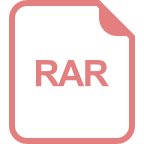
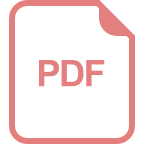
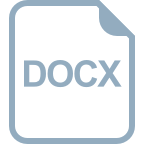
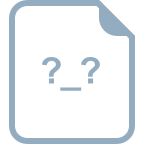
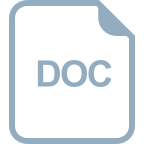
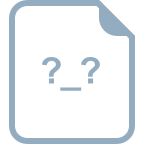










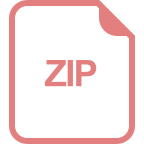
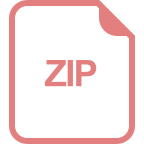