def __init__(self): self.window = QMainWindow() self.window.resize(550, 400) self.window.move(300, 310) self.window.setWindowTitle('产生式系统') self.textEdit = QPlainTextEdit(self.window) self.textEdit.setPlaceholderText("请输入已有特征信息的序号(以空格分隔)") self.textEdit.move(10, 25) self.textEdit.resize(300, 350) self.button1 = QPushButton('输入完成', self.window) self.button1.move(380, 220) self.button1.clicked.connect(self.handle) self.button2 = QPushButton('所有植物特征信息', self.window) self.button2.resize(150, 100) self.button2.move(360, 80) self.button2.clicked.connect(self.display_init)
时间: 2024-02-23 08:57:06 浏览: 17
这段代码是一个基于PyQt5的GUI应用程序,它创建了一个主窗口,并在窗口中添加了一个文本编辑框和两个按钮。
在程序初始化时,首先创建了一个QMainWindow对象,并设置了它的大小、位置和标题。然后,创建了一个QPlainTextEdit对象,并将其添加到窗口中,该文本编辑框用于输入已有特征信息的序号。接下来,创建了两个QPushButton对象,一个用于提交已有特征信息,另一个用于显示所有植物的特征信息。
按钮1的clicked信号连接了一个名为handle的槽函数,该函数在用户提交已有特征信息时被调用。按钮2的clicked信号连接了一个名为display_init的槽函数,该函数用于显示所有植物的特征信息。
这段代码还需要完成其他函数的定义才能正常运行。
相关问题
import pandas as pd from PyQt5.QtWidgets import QApplication, QTableView, QMainWindow, QVBoxLayout, QWidget, QPushButton, QFileDialogfrom PyQt5 import QtCoreclass ExcelViewer(QMainWindow): def __init__(self): super().__init__() self.df = None # 初始值为 None self.table_view = QTableView() self.setCentralWidget(self.table_view) self.initUI() def initUI(self): self.setWindowTitle("Excel Viewer") self.resize(800, 600) # 添加查询按钮 self.button = QPushButton("查询", self) self.button.setGeometry(QtCore.QRect(340, 20, 80, 30)) self.button.clicked.connect(self.selectExcel) def selectExcel(self): # 弹出文件选择窗口 file_path, _ = QFileDialog.getOpenFileName(self, "选择Excel文件", "", "Excel Files (*.xlsx *.xls)") if file_path: # 读取 Excel 文件并将其转换为 pandas DataFrame 对象 self.df = pd.read_excel(file_path) # 将 DataFrame 数据设置为表格模型并在 QTableView 中显示 model = pdTableModel(self.df) self.table_view.setModel(model)class pdTableModel(QtCore.QAbstractTableModel): def __init__(self, data): super().__init__() self._data = data def data(self, index, role): if role == QtCore.Qt.DisplayRole: return str(self._data.iloc[index.row(), index.column()]) def rowCount(self, index): return self._data.shape[0] def columnCount(self, index): return self._data.shape[1]if __name__ == '__main__': app = QApplication([]) excel_viewer = ExcelViewer() excel_viewer.show() app.exec_()帮我修改,不需要自己选择文件
好的,如果不需要手动选择文件,可以在 ExcelViewer 类的 initUI() 方法中直接调用 pd.read_excel() 方法读取固定的 Excel 文件,代码如下:
```python
import pandas as pd
from PyQt5.QtWidgets import QApplication, QTableView, QMainWindow, QVBoxLayout, QWidget, QPushButton, QFileDialog
from PyQt5 import QtCore
class ExcelViewer(QMainWindow):
def __init__(self):
super().__init__()
self.df = None # 初始值为 None
self.table_view = QTableView()
self.setCentralWidget(self.table_view)
self.initUI()
def initUI(self):
self.setWindowTitle("Excel Viewer")
self.resize(800, 600)
# 添加查询按钮
self.button = QPushButton("查询", self)
self.button.setGeometry(QtCore.QRect(340, 20, 80, 30))
self.button.clicked.connect(self.selectExcel)
def selectExcel(self):
# 读取 Excel 文件并将其转换为 pandas DataFrame 对象
self.df = pd.read_excel("example.xlsx")
# 将 DataFrame 数据设置为表格模型并在 QTableView 中显示
model = pdTableModel(self.df)
self.table_view.setModel(model)
class pdTableModel(QtCore.QAbstractTableModel):
def __init__(self, data):
super().__init__()
self._data = data
def data(self, index, role):
if role == QtCore.Qt.DisplayRole:
return str(self._data.iloc[index.row(), index.column()])
def rowCount(self, index):
return self._data.shape[0]
def columnCount(self, index):
return self._data.shape[1]
if __name__ == '__main__':
app = QApplication([])
excel_viewer = ExcelViewer()
excel_viewer.show()
app.exec_()
```
在这里,我们直接将文件名设置为 "example.xlsx",并在 selectExcel() 方法中删除了弹出文件选择窗口的代码,这样程序启动后,表格中就会显示 example.xlsx 文件的内容。
import pandas as pd from PyQt5.QtWidgets import QApplication, QTableView, QMainWindow, QVBoxLayout, QWidget class ExcelViewer(QMainWindow): def __init__(self, file_path): super().__init__() # 读取 Excel 文件并将其转换为 pandas DataFrame 对象 self.df = pd.read_excel(file_path) # 创建 QTableView 组件并将其设置为中心窗口部件 self.table_view = QTableView() self.setCentralWidget(self.table_view) # 将 DataFrame 数据设置为表格模型并在 QTableView 中显示 model = pdTableModel(self.df) self.table_view.setModel(model) class pdTableModel(QtCore.QAbstractTableModel): def __init__(self, data): super().__init__() self._data = data def data(self, index, role): if role == QtCore.Qt.DisplayRole: return str(self._data.iloc[index.row(), index.column()]) def rowCount(self, index): return self._data.shape[0] def columnCount(self, index): return self._data.shape[1] if __name__ == '__main__': app = QApplication([]) excel_viewer = ExcelViewer('example.xlsx') excel_viewer.show() app.exec_()帮我修改成按查询按钮显示Excel
好的,以下是修改后的代码,添加了一个查询按钮和一个槽函数,点击查询按钮后会弹出一个文件选择窗口,用户选择Excel文件后,程序会读取该文件并在主窗口中显示:
```
import pandas as pd
from PyQt5.QtWidgets import QApplication, QTableView, QMainWindow, QVBoxLayout, QWidget, QPushButton, QFileDialog
from PyQt5 import QtCore
class ExcelViewer(QMainWindow):
def __init__(self):
super().__init__()
self.df = None # 初始值为 None
self.table_view = QTableView()
self.setCentralWidget(self.table_view)
self.initUI()
def initUI(self):
self.setWindowTitle("Excel Viewer")
self.resize(800, 600)
# 添加查询按钮
self.button = QPushButton("查询", self)
self.button.setGeometry(QtCore.QRect(340, 20, 80, 30))
self.button.clicked.connect(self.selectExcel)
def selectExcel(self):
# 弹出文件选择窗口
file_path, _ = QFileDialog.getOpenFileName(self, "选择Excel文件", "", "Excel Files (*.xlsx *.xls)")
if file_path:
# 读取 Excel 文件并将其转换为 pandas DataFrame 对象
self.df = pd.read_excel(file_path)
# 将 DataFrame 数据设置为表格模型并在 QTableView 中显示
model = pdTableModel(self.df)
self.table_view.setModel(model)
class pdTableModel(QtCore.QAbstractTableModel):
def __init__(self, data):
super().__init__()
self._data = data
def data(self, index, role):
if role == QtCore.Qt.DisplayRole:
return str(self._data.iloc[index.row(), index.column()])
def rowCount(self, index):
return self._data.shape[0]
def columnCount(self, index):
return self._data.shape[1]
if __name__ == '__main__':
app = QApplication([])
excel_viewer = ExcelViewer()
excel_viewer.show()
app.exec_()
```
注意事项:
1. `ExcelViewer` 类的构造函数中不再接收 `file_path` 参数,因为文件路径是在点击查询按钮后由用户选择的。
2. `ExcelViewer` 类中添加了一个 `selectExcel` 槽函数,用于响应用户点击查询按钮的事件。
3. `selectExcel` 槽函数通过调用 `QFileDialog.getOpenFileName` 方法弹出文件选择窗口,让用户选择 Excel 文件。
4. `selectExcel` 槽函数读取用户选择的 Excel 文件并将其转换为 pandas DataFrame 对象,如果读取成功则将其在主窗口中显示。
相关推荐
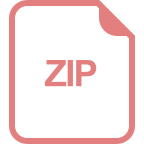
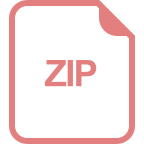












