写一个python函数,将已经转换为YOLO格式的标注写入txt文档
时间: 2024-05-10 16:16:21 浏览: 113
以下是一个将已经转换为YOLO格式的标注写入txt文档的Python函数:
```python
def write_yolo_annotation_to_txt(yolo_annotations, output_file):
with open(output_file, 'w') as f:
for annotation in yolo_annotations:
line = f"{annotation['class_id']} {annotation['x_center']} {annotation['y_center']} {annotation['width']} {annotation['height']}\n"
f.write(line)
```
其中,`yolo_annotations` 是一个列表,每个元素都是一个字典,包含以下字段:
- `class_id`:物体的类别ID
- `x_center`:物体在图像中心点的x坐标(相对于图像宽度的比例)
- `y_center`:物体在图像中心点的y坐标(相对于图像高度的比例)
- `width`:物体的宽度(相对于图像宽度的比例)
- `height`:物体的高度(相对于图像高度的比例)
`output_file` 是输出的txt文件路径。函数会遍历 `yolo_annotations` 列表中的每个元素,并将其转换成YOLO格式的一行字符串,然后将其写入到输出文件中。
相关问题
请给我 将DOTA数据集转换为yolo格式的python代码
### 回答1:
可以参考下面的代码,它可以帮助你将DOTA数据集转换为yolo格式:import cv2
import numpy as np # 读取DOTA数据集
img = cv2.imread('image.png') # 转换为YOLO格式
h, w, _ = img.shape
boxes = []
for label, fname in labels:
xmin, ymin, xmax, ymax = fname
xmin = xmin / w
xmax = xmax / w
ymin = ymin / h
ymax = ymax / h
boxes.append([label, xmin, ymin, xmax, ymax]) # 将数据写入yolo格式文件
with open('labels.txt', 'w') as f:
for box in boxes:
f.write(' '.join([str(box[0]), str(box[1]), str(box[2]), str(box[3]), str(box[4])]))
f.write('\n')
### 回答2:
要将DOTA数据集转换为Yolo格式的Python代码,你需要进行以下步骤:
1. 遍历DOTA数据集中的每个图像,并打开它。
2. 对于每个图像,读取DOTA标注文件,该文件包含了目标的类别和边界框坐标。
3. 将DOTA的坐标格式转换为Yolo的坐标格式。DOTA使用(x_min, y_min, x_max, y_max)的格式,而Yolo使用(x, y, width, height)的格式。你可以使用以下公式进行转换:
x = (x_min + x_max) / 2
y = (y_min + y_max) / 2
width = x_max - x_min
height = y_max - y_min
4. 将转换后的类别标签和边界框坐标写入Yolo格式的标注文件中,每个对象占据一行。Yolo的标注文件格式为:class_index x y width height。
5. 将转换后的图像保存到Yolo格式的图像文件夹中,以相同的文件名命名。
6. 重复以上步骤,直到遍历完整个DOTA数据集。
下面是一个示例代码框架:
```python
import os
import glob
def convert_dota_to_yolo(dota_dataset_path, yolo_dataset_path):
# 创建Yolo格式的图像文件夹
os.makedirs(yolo_dataset_path, exist_ok=True)
# 遍历DOTA数据集中的每个图像
for dota_image in glob.glob(os.path.join(dota_dataset_path, '*.jpg')):
# 打开图像
img = ... # 使用适当的图像处理库打开图像
# 读取DOTA标注文件
dota_annotation = ... # 使用适当的方法读取DOTA标注文件
# 创建Yolo格式的标注文件
yolo_annotation = os.path.join(yolo_dataset_path, os.path.basename(dota_image.replace('.jpg', '.txt')))
with open(yolo_annotation, 'w') as f:
# 将DOTA标注转换为Yolo格式并写入文件
for annotation in dota_annotation:
class_index = ... # 根据类别名称获得类别索引
x = ...
y = ...
width = ...
height = ...
annotation_line = f'{class_index} {x} {y} {width} {height}\n'
f.write(annotation_line)
# 保存Yolo格式的图像
yolo_image_path = os.path.join(yolo_dataset_path, os.path.basename(dota_image))
img.save(yolo_image_path)
```
请注意,上述代码仅为示例框架,你需要根据实际的DOTA数据集和Yolo格式的要求进行适当的调整。
### 回答3:
将DOTA数据集转换为YOLO格式的python代码可以分为以下几个步骤:
1. 导入必要的库
首先,需要导入一些必要的库,例如`os`、`xml.etree.ElementTree`等。
2. 定义类别和标签字典
在YOLO格式中,需要定义一个类别和标签的字典,其中每个类别对应一个唯一的整数。
3. 解析DOTA数据集
使用`xml.etree.ElementTree`库来解析DOTA数据集的XML文件,获取目标的类别和边界框信息。
4. 转换为YOLO格式
根据YOLO格式的要求,将DOTA数据集的边界框信息转换为YOLO格式的边界框坐标。
5. 保存转换后的数据
按照YOLO格式的要求,将转换后的数据保存为文本文件,每行表示一个目标的类别和边界框坐标。
以下是一个示例的python代码:
```python
import os
import xml.etree.ElementTree as ET
# 定义类别和标签字典
class_dict = {'class1': 0, 'class2': 1, 'class3': 2}
def convert_dota_to_yolo(dota_path, yolo_path):
for filename in os.listdir(dota_path):
if filename.endswith('.xml'):
tree = ET.parse(os.path.join(dota_path, filename))
root = tree.getroot()
with open(os.path.join(yolo_path, filename.split('.')[0] + '.txt'), 'w') as f:
for object in root.findall('object'):
class_name = object.find('name').text
bbox = object.find('bndbox')
xmin = int(bbox.find('xmin').text)
ymin = int(bbox.find('ymin').text)
xmax = int(bbox.find('xmax').text)
ymax = int(bbox.find('ymax').text)
xcenter = (xmin + xmax) / 2
ycenter = (ymin + ymax) / 2
width = xmax - xmin
height = ymax - ymin
class_id = class_dict[class_name]
f.write(f'{class_id} {xcenter} {ycenter} {width} {height}\n')
# 调用函数将DOTA数据集转换为YOLO格式
convert_dota_to_yolo('DOTA_dataset_path', 'YOLO_dataset_path')
```
请注意,上述代码中的`'class1'`,`'class2'`,`'class3'`等表示DOTA数据集的类别名称,需要根据实际情况进行修改。另外,转换后的YOLO格式数据将保存在指定的`yolo_path`路径下。
用python代码将labelme标注的数据标注集转换成yolo可识别的数据标注集
在Python中,将LabelMe标注的数据转换为YOLO所需的格式,通常需要几个步骤。LabelMe是一个图像标注工具,而YOLO是一种目标检测算法,它们的数据结构有所不同。这里提供一个简化版的示例,假设你已经有一个包含LabelMe JSON文件的目录:
```python
import os
import json
from PIL import Image
from pascal_voc_writer import Writer
def labelme_to_yolo(labelme_json_dir, output_yolo_dir):
# 创建YOLO所需的文件结构
if not os.path.exists(output_yolo_dir):
os.makedirs(output_yolo_dir)
for filename in os.listdir(labelme_json_dir):
if filename.endswith(".json"):
with open(os.path.join(labelme_json_dir, filename), "r") as f:
data = json.load(f)
img_path = os.path.join(labelme_json_dir, data["imagePath"])
im = Image.open(img_path)
width, height = im.size
annotations = []
for shape in data["shapes"]:
label = shape["label"]
points = [(x, y) for x, y, w, h in shape["points"]]
x_min, y_min = min(points, key=lambda xy: xy[0])[0], min(points, key=lambda xy: xy[1])[1]
x_max, y_max = max(points, key=lambda xy: xy[0])[0] + w, max(points, key=lambda xy: xy[1])[1] + h
box_w, box_h = x_max - x_min, y_max - y_min
object_name = f"{label}_box"
annotations.append({
"class": object_name,
"xmin": x_min,
"ymin": y_min,
"xmax": x_max,
"ymax": y_max,
"obj": 1
})
# 写入YOLO所需的txt文件
txt_file = os.path.join(output_yolo_dir, filename[:-5] + ".txt")
with open(txt_file, "w") as txt_f:
Writer(txt_file).write(annotations, names=[f"{i}_box" for i in range(len(annotations))],
image_size=(width, height))
# 使用函数转换数据
labelme_to_yolo("path/to/labelme/json", "path/to/output/yolo")
```
这个脚本读取每个LabelMe JSON,提取出边界框信息并转换为YOLO所需的格式(每行一个对象,包含类别名、坐标)。注意实际使用时可能需要根据LabelMe JSON的具体结构进行调整。
阅读全文
相关推荐
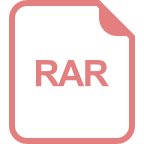
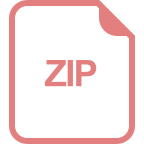
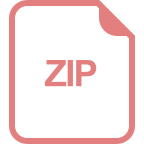
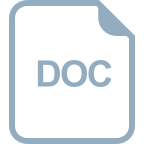
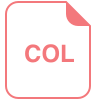
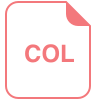
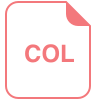
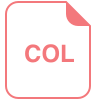
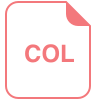
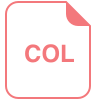
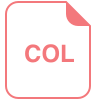
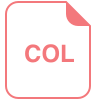


