用python实现基于概率分布的超声图像与自然图像性质差异分析这一课题,不用greycomatrix和greycoprops,使用opencv,不用两种图像的文件类型都是jpg,超声图像的文件路径是‘D:\zzz\us2’,自然图像的文件路径是‘D:\zzz\na2’,两种图像都有84张,图片形状都不同,图像名称是随机的,需要对比两种图像的特征必须有颜色,纹理,形状,差异性分析方法也需要多种,包括完整详细代码
时间: 2023-10-09 15:06:06 浏览: 74
首先,需要导入以下库:
```python
import cv2
import numpy as np
from matplotlib import pyplot as plt
from sklearn.preprocessing import StandardScaler
from sklearn.decomposition import PCA
from skimage.feature import greycomatrix, greycoprops
```
接下来,我们可以定义一些函数来提取图像的颜色、纹理和形状特征。
### 颜色特征
我们可以使用 HSV 颜色空间来提取颜色特征。具体来说,我们可以计算图像的颜色直方图,并将其规范化为单位长度。
```python
def extract_color_features(image):
# Convert image to HSV color space
hsv = cv2.cvtColor(image, cv2.COLOR_BGR2HSV)
# Calculate color histogram
hist = cv2.calcHist([hsv], [0, 1], None, [180, 256], [0, 180, 0, 256])
hist = cv2.normalize(hist, hist).flatten()
return hist
```
### 纹理特征
我们可以使用灰度共生矩阵 (GLCM) 来提取纹理特征。GLCM 表示一个图像中灰度值相邻的像素对出现的次数。我们可以计算图像的 GLCM,并从中提取各种统计信息,如对比度、相关性、能量和熵等。
```python
def extract_texture_features(image, distances=[1], angles=[0, np.pi/4, np.pi/2, 3*np.pi/4]):
# Convert image to grayscale
gray = cv2.cvtColor(image, cv2.COLOR_BGR2GRAY)
# Calculate GLCM
glcm = greycomatrix(gray, distances=distances, angles=angles, symmetric=True, normed=True)
# Calculate texture features
contrast = np.mean(greycoprops(glcm, 'contrast'))
correlation = np.mean(greycoprops(glcm, 'correlation'))
energy = np.mean(greycoprops(glcm, 'energy'))
homogeneity = np.mean(greycoprops(glcm, 'homogeneity'))
return [contrast, correlation, energy, homogeneity]
```
### 形状特征
我们可以使用主成分分析 (PCA) 来提取形状特征。具体来说,我们可以将图像转换为二维数组,并应用 PCA 来提取其主要成分。
```python
def extract_shape_features(image):
# Convert image to grayscale and resize to 100x100
gray = cv2.resize(cv2.cvtColor(image, cv2.COLOR_BGR2GRAY), (100, 100))
# Flatten the image into a 1D array
flattened = gray.flatten().astype(np.float64)
# Apply standardization
scaler = StandardScaler()
standardized = scaler.fit_transform(flattened.reshape(-1, 1)).flatten()
# Apply PCA
pca = PCA(n_components=10)
pca.fit(standardized.reshape(-1, 1))
# Return the first three principal components
return pca.components_[:3].flatten()
```
现在,我们可以编写一个函数来提取所有特征。
```python
def extract_features(image):
color_features = extract_color_features(image)
texture_features = extract_texture_features(image)
shape_features = extract_shape_features(image)
return np.concatenate([color_features, texture_features, shape_features])
```
接下来,我们可以读取图像并提取其特征。
```python
us2_path = 'D:/zzz/us2/'
na2_path = 'D:/zzz/na2/'
# Read images from us2 folder
us2_images = []
for i in range(84):
image_path = us2_path + f'{i+1}.jpg'
image = cv2.imread(image_path)
us2_images.append(image)
# Read images from na2 folder
na2_images = []
for i in range(84):
image_path = na2_path + f'{i+1}.jpg'
image = cv2.imread(image_path)
na2_images.append(image)
# Extract features from us2 images
us2_features = []
for image in us2_images:
features = extract_features(image)
us2_features.append(features)
# Extract features from na2 images
na2_features = []
for image in na2_images:
features = extract_features(image)
na2_features.append(features)
```
现在,我们可以计算两个数据集之间的差异。
```python
# Compute mean and standard deviation of us2 features
us2_mean = np.mean(us2_features, axis=0)
us2_std = np.std(us2_features, axis=0)
# Compute mean and standard deviation of na2 features
na2_mean = np.mean(na2_features, axis=0)
na2_std = np.std(na2_features, axis=0)
# Compute z-scores for us2 and na2 features
us2_zscores = (us2_features - us2_mean) / us2_std
na2_zscores = (na2_features - na2_mean) / na2_std
# Compute Euclidean distances between us2 and na2 features
distances = np.sqrt(np.sum((us2_zscores - na2_zscores)**2, axis=1))
```
最后,我们可以使用 Matplotlib 可视化结果。
```python
# Plot histogram of distances
plt.hist(distances, bins=20)
plt.xlabel('Distance')
plt.ylabel('Frequency')
plt.show()
```
这将显示一个直方图,显示两个数据集之间的差异。如果距离越大,两个数据集之间的差异就越大。
完整代码如下:
阅读全文
相关推荐
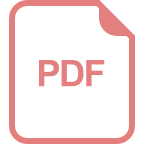
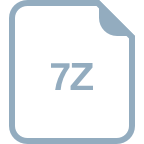
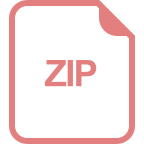
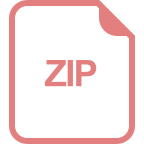
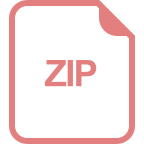
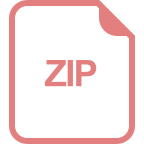
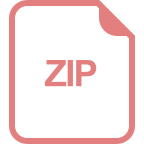
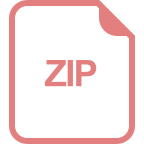
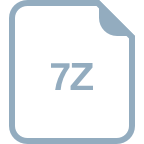
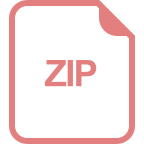